Question
import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.Tab; import javafx.scene.control.TabPane; import javafx.scene.layout.StackPane; import java.util.ArrayList; public class Assignment6 extends Application { private TabPane tabPane; private CreatePane
import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.Tab; import javafx.scene.control.TabPane; import javafx.scene.layout.StackPane; import java.util.ArrayList;
public class Assignment6 extends Application { private TabPane tabPane; private CreatePane createPane; private ReviewPane reviewPane; private ArrayList
public void start(Stage stage) { StackPane root = new StackPane();
//movieList to be used in both createPane & reviewPane movieList = new ArrayList
reviewPane = new ReviewPane(movieList); createPane = new CreatePane(movieList, reviewPane);
tabPane = new TabPane();
Tab tab1 = new Tab(); tab1.setText("Movie Creation"); tab1.setContent(createPane);
Tab tab2 = new Tab(); tab2.setText("Movie Review"); tab2.setContent(reviewPane);
tabPane.getSelectionModel().select(0); tabPane.getTabs().addAll(tab1, tab2);
root.getChildren().add(tabPane);
Scene scene = new Scene(root, 700, 400); stage.setTitle("Movie Review Apps"); stage.setScene(scene); stage.show(); }
public static void main(String[] args) { launch(args); } }
public class Movie { private String movieTitle; private int year; private int length; private Review bookReview; //Constructor to initialize all member variables public Movie() { movieTitle = "?"; length = 0; year = 0; bookReview = new Review(); }
//Accessor methods public String getMovieTitle() { return movieTitle; }
public int getLength() { return length; }
public int getYear() { return year; }
public Review getReview() { return bookReview; }
//Mutator methods public void setMovieTitle(String aTitle) { movieTitle = aTitle; }
public void setLength(int aLength) { length = aLength; }
public void setYear(int aYear) { year = aYear; }
public void addRating(double rate) { bookReview.updateRating(rate); }
//toString() method returns a string containg the information on the movie public String toString() { String result = " Movie Title:\t\t" + movieTitle + " Movie Length:\t\t" + length + " Movie Year:\t\t" + year + " " + bookReview.toString() + " "; return result; } }
import java.text.DecimalFormat;
public class Review { private int numberOfReviews; private double sumOfRatings; private double average;
//Constructor to initialize all member variables public Review() { numberOfReviews = 0; sumOfRatings = 0.0; average = 0.0; }
//It updates the number of REviews and avarage based on the //an additional rating specified by its parameter public void updateRating(double rating) { numberOfReviews++; sumOfRatings += rating; if (numberOfReviews > 0) { average = sumOfRatings/numberOfReviews; } else average = 0.0; }
//toString() method returns a string containg its review average //and te number of Reviews public String toString() { DecimalFormat fmt = new DecimalFormat("0.00"); String result = "Reviews:\t" + fmt.format(average) + "(" + numberOfReviews + ")"; return result;
import java.util.ArrayList; import javafx.scene.layout.HBox; import javafx.application.Application; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.layout.GridPane; //*** import javafx.geometry.Insets; import javafx.geometry.Pos; import javafx.geometry.HPos; //import all other necessary javafx classes here //----
public class CreatePane extends HBox { private ArrayList
//constructor public CreatePane(ArrayList
//Step #1: initialize each instance variable and set up the layout //---- //create a GridPane hold those labels & text fields //consider using .setPadding() or setHgap(), setVgap() //to control the spacing and gap, etc. //---- public void list (stage primaryStage) { // Create a GridPane object and set its properties GridPane pane = new GridPane(); pane.setAlignment(Pos.CENTER); //always place the GridPane in the center of scene pane.setPadding(new Insets(11.5, 12.5, 13.5, 14.5)); pane.setHgap(5.5); //set horizontal gap between columns pane.setVgap(5.5); //set vertical gap between rows
} //You might need to create a sub pane to hold the button //---- //Set up the layout for the left half of the CreatePane. //---- //the right half of this pane is simply a TextArea object //Note: a ScrollPane will be added to it automatically when there are no //enough space //Add the left half and right half to the CreatePane //Note: CreatePane extends from HBox //---- //Step #3: register source object with event handler //---- } //end of constructor
//Step 2: Create a ButtonHandler class //ButtonHandler listens to see if the button "Create a Movie" is pushed or not, //When the event occurs, it get a movie's Title, Year, and Length //information from the relevant text fields, then create a new movie and add it inside //the movieList. Meanwhile it will display the movie's information inside the text area. //It also does error checking in case any of the textfields are empty or non-numeric string is typed private class ButtonHandler implements EventHandler
import javafx.scene.control.ListView; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.scene.layout.VBox; import javafx.event.ActionEvent; //**Need to import to handle event import javafx.event.EventHandler; //**Need to import to handle event
import java.util.ArrayList; import javafx.scene.layout.HBox;
//import all other necessary javafx classes here //----
public class ReviewPane extends VBox { private ArrayList
//A ListView to display movies created private ListView
//declare all other necessary GUI variables here //---- //constructor public ReviewPane(ArrayList
//set up the layout //---- //ReviewPane is a VBox - add the components here //---- //Step #3: Register the button with its handler class //---- } //end of constructor
//This method refresh the ListView whenever there's new movie added in CreatePane //you will need to update the underline ObservableList object in order for ListView //object to show the updated movie list public void updateMovieList(Movie newMovie) { //------- }
//Step 2: Create a RatingHandler class private class RatingHandler implements EventHandler
Step by Step Solution
There are 3 Steps involved in it
Step: 1
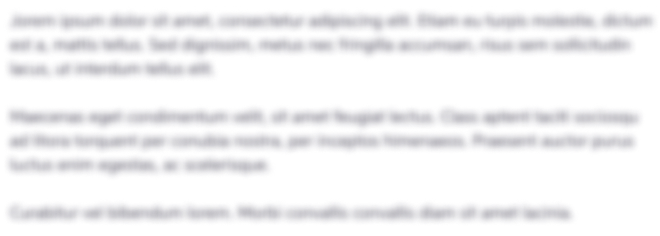
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started