Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.*; public class MyLinkedList{ public static void main(String[] args){ } } //copy MyList to here interface MyList extends Collection { /* MyList will inherite
*24.3 (Implement a doubly linked list) The MyLinkedL ist class used in Listing 24.5 is a one-way directional linked list that enables one-way traversal of the list. Modify the Node class to add the new data field name previous to refer to the previous node in the list, as follows: public class Node \{ E element; Node next: Node previous: public Node(E e) \{ element = e: \} \} Implement a new class named TwoWayLinkedList that uses a doubly linked list to store elements. Define TwowayLinkedList to implements MyList. You need to implement all the methods defined in MyLinkedList as well as the methods listiterator () and listiterator (int index). Both return an instance of j ava. util.Listiterator (see Figure 20.4 ). The former sets the cursor to the head of the list and the latter to the element at the specified index. *24.3 (Implement a doubly linked list) The MyLinkedL ist class used in Listing 24.5 is a one-way directional linked list that enables one-way traversal of the list. Modify the Node class to add the new data field name previous to refer to the previous node in the list, as follows: public class Node \{ E element; Node next: Node previous: public Node(E e) \{ element = e: \} \} Implement a new class named TwoWayLinkedList that uses a doubly linked list to store elements. Define TwowayLinkedList to implements MyList. You need to implement all the methods defined in MyLinkedList as well as the methods listiterator () and listiterator (int index). Both return an instance of j ava. util.Listiterator (see Figure 20.4 ). The former sets the cursor to the head of the list and the latter to the element at the specified indeximport java.util.*; public class MyLinkedList{ public static void main(String[] args){ } } //copy MyList to here interface MyList
extends Collection { /* MyList will inherite all methods from Collection public boolean add(E e); public boolean addAll(Collection extends E> c); public void clear(); public boolean contains(Object o); public boolean containsAll(Collection> c); public boolean isEmpty(); public boolean remove(Object o); public boolean removeAll(Collection> c); public boolean retainAll(Collection> c); public int size(); public Object[] toArray(); public T[] toArray(T[] arr); */ //abstract method public void add(int index, E e); public E get(int index); public int indexOf(Object e); public int lastIndexOf(E e); public E remove(int index); public E set(int index, E e); //default method @Override public default boolean add(E e){ add(size(), e); return true; } @Override public default boolean isEmpty(){ return size()==0; } @Override public default boolean remove(Object e){ if(indexOf(e) >= 0){ remove(indexOf(e)); return true; } else{ return false; } } @Override public default boolean containsAll(Collection> c){ for(Object e : c){ if(!contains(e)){ return false; } } return true; } @Override public default boolean addAll( Collection extends E> c){ for(E e : c){ add(e); } return true; } /* removeAll: this[1,1,2,3] c[1,2,4] this.removeAll(c) [3] */ @Override public default boolean removeAll( Collection> c){ for(Object e : c){ while(contains(e)){ this.remove(e); } } return true; } /* retainAll this[1,1,2,3] c[1,2,4] this.retainAll(c) [1,1,2] */ @Override public default boolean retainAll( Collection> c){ for(E e : this){ if(!c.contains(e)){ this.remove(e); } } return true; } @Override public default Object[] toArray(){ Object[] results = new Object[size()]; int i = 0; for(E e : this){ results[i++] = e; } return results; } /* T[] array */ @Override public default T[] toArray(T[] array){ int i = 0; for(E e : this){ array[i++] = (T)e; } return array; } } class TwoWayLinkedList implements MyList { //inner static class Node public class Node { E element; Node next; Node previous; public Node(E e){ element = e; } } private Node head, tail; private int size = 0; public TwoWayLinkedList(){ /* head = null; tail = null; size = 0; */ } public TwoWayLinkedList(E[] objects){ } public E getFirst(){ return null; } public E getLast(){ return null; } public void addFirst(E e){ } public void addLast(E e){ } public E removeFirst(){ return null; } public E removeLast(){ return null; } @Override public void add(int index, E e){ } @Override public E remove(int index){ return null; } @Override public void clear(){ } @Override public boolean contains(Object e){ return false; } @Override public E get(int index){ return null; } @Override public int indexOf(Object e){ return -1; } @Override public int lastIndexOf(Object e){ return -1; } @Override public E set(int index, E e){ return null; } @Override public int size(){ return size; } //toString() @Override public String toString(){ //[1,2,3] StringBuilder result = new StringBuilder("["); for(Node current = head; current != null; current = current.next) { result.append(current.element); if(current != tail){ result.append(", "); } } result.append("]"); return result.toString(); } @Override public Iterator iterator(){ return new LinkedListIterator(); } //enhenced for loop //inner class private class LinkedListIterator implements Iterator { private Node current = head; @Override public boolean hasNext(){ return current != null; } @Override public E next(){ E e = current.element; current = current.next; return e; } @Override public void remove(){ /ot call next() yet if(current == head){ throw new IllegalStateException("next() yet called yet"); } else if(current == null){ TwoWayLinkedList.this.removeLast(); } else{ int indexOfremoved = indexOf(current.element) - 1; TwoWayLinkedList.this.remove(indexOfremoved); } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
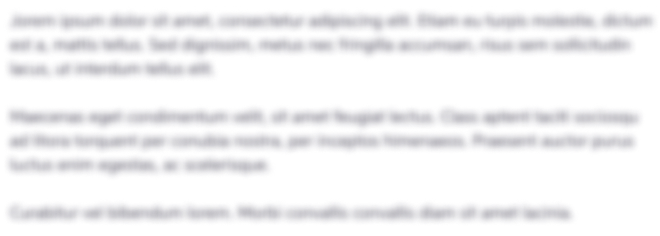
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started