Question
import java.util.ArrayList; /* * * A program to test the Partitionable interface using two classes: Employee and CreditCard */ public class QI3 { //Prints out
import java.util.ArrayList;
/*
*
* A program to test the Partitionable interface using two classes: Employee and CreditCard
*/
public class QI3
{
//Prints out the parts of each object in the given array objects.
//Hint: make use of the getParts() method of each object
static void printParts(Partitionable[] objects)
{
ArrayList parts = new ArrayList();
//-----------Start below here. To do: approximate lines of code = 4
// For each object, print each part of an object on a separate line
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
public static void main(String[] args)
{
Employee[] employees = new Employee[3];
String[] names = {"Al", "Betty", "Connie"};
String[] addresses = {"2 Bloor Street", "5000 Yonge Street", "61 University Avenue"};
for (int i = 0; i < employees.length; i++)
{
employees[i] = new Employee(names[i], addresses[i]);
}
printParts(employees);
System.out.println("Expected:2BloorStreet5000YongeStreet61UniversityAvenue");
CreditCard[] cards = new CreditCard[3];
String[] numbers = {"4532123157644657", "4532132957623657", "4519123157666651"};
for (int i = 0; i < cards.length; i++)
{
cards[i] = new CreditCard(names[i], numbers[i]);
}
printParts(cards);
System.out.println("Expected:453212315764465745321329576236574519123157666651");
}
}
—
—
—
import java.util.ArrayList;
import java.util.Scanner;
/*
* A basic employee class holding the name and address string
*/
//-----------Start below here. To do: approximate lines of code = 1
// Make class Employee implement the Partitionable interface
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
{
String fullName;
String address;
public Employee(String name, String address)
{
this.fullName = name;
this.address = address;
}
//-----------Start below here. To do: approximate lines of code = 6
// Implement the Partitionable interface (see Partitionable.java)
//For an employee, the parts are the parts of the address
//For example: if the address string is "12 Drury Lane" then the parts are "12", "Drury" and "Lane"
//Hint: use a scanner to get the part strings. Don't forget to create an empty array list initially
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
—
—
—
import java.util.ArrayList;
/*
* Simple CreditCard class that holds a name of the card owner and their creditcard number
*
* A credit card number string consists of 16 digits. There are 4 parts of 4 digits each
* For example, if the card number string is "4518354201187632" then the parts are "4518", "3542", "0118", "7632"
*/
//-----------Start below here. To do: approximate lines of code = 1
// Make class CreditCard implement the Partitionable interface
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
{
private String name;
private String number;
public CreditCard(String name, String number)
{
this.name = name;
this.number = number;
}
//-----------Start below here. To do: approximate lines of code = 7
// Implement the Partitionable interface (see Partitionable.java)
//Hint: use the substring() method of class String. Don't forget to create an empty array list initially.
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
Partitionable.java
import java.util.ArrayList;
public interface Partitionable
{
// Takes any string and returns an array list of its parts.
ArrayList getParts();
}
Include the output screenshot yo, so we can double all is working good!
Step by Step Solution
3.40 Rating (156 Votes )
There are 3 Steps involved in it
Step: 1
QI3java import javautilArrayList A program to test the Partitionable interface using two classes Employee and CreditCard public class QI3 Prints out the parts of each object in the given array objects ...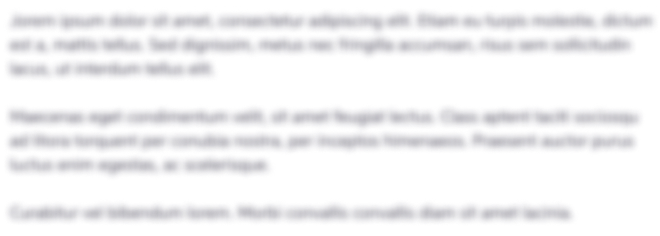
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started