Question
import java.util.ArrayList; import java.util.Scanner; import java.io.File; public class BookMain { LetterTally letterData; WordTally wordData; SentenceTally sentenceData; public BookMain() { letterData = new LetterTally(); wordData =
import java.util.ArrayList;
import java.util.Scanner;
import java.io.File;
public class BookMain {
LetterTally letterData;
WordTally wordData;
SentenceTally sentenceData;
public BookMain() {
letterData = new LetterTally();
wordData = new WordTally();
sentenceData = new SentenceTally();
}
public static void main(String[] args) throws Exception {
BookMain bm = new BookMain();
Scanner input = new Scanner(new File("PrideAndPrejudice.txt"));
bm.readHeader(input);
bm.analyzeBookText(input);
System.out.println(bm.statToString());
input.close();
}
public void analyzeBookText(Scanner input) {
ArrayList
while(input.hasNext()) {
book.add(input.next());
}
LetterTally.setText(book);
SentenceTally.setText(book);
WordTally.setText(book);
}
public String statToString() {
String s = "";
s += "Raw letter count: " + letterData.totalCount() + " ";
s += "Letter entropy: " + letterData.computeEntropy() + " ";
s += "Raw word count: " + wordData.getRawCount() + " ";
s += "Unique word count: " + wordData.getUniqueCount() + " ";
s += "Longest word: " + wordData.longestWord() + " ";
s += "Average word length: " + wordData.avgWordLength() + " ";
s += "Sentence count: " + sentenceData.getCount() + " ";
s += "Average sentence length: " + sentenceData.avgSentenceLength() + " ";
return s;
}
private void readHeader(Scanner input) {
int nHeaderLine = 31;
int nCurLine = 0;
while (input.hasNext() && nCurLine < nHeaderLine) {
input.nextLine();
nCurLine++;
if (nCurLine == nHeaderLine)
break;
}
}
}
import java.util.ArrayList;
public class LetterTally {
private static ArrayList
public LetterTally() {
text = new ArrayList
}
public static void setText(ArrayList
LetterTally.text = text;
}
public int totalCount() {
int count = 0;
for(String s : text) {
for(int i = 0 ; i < s.length(); i++){
if(Character.isLetter(s.charAt(i))){
count++;
}
}
}
return count;
}
public int freqChar(char ch) {
int charCount = 0;
for(String s : text) {
for(int i = 0 ; i < s.length(); i++){
if(s.charAt(i) == ch){
charCount++;
}
}
}
return charCount;
}
public int computeEntropy() {
double entropy = 0;
double [] p = new double [26];
for(int i = 0; i < p.length; i++) {
p [i] = ((freqChar((char) 0)) / ((double)totalCount()));
if(p[i] != 0) {
entropy += p[i] * (Math.log(1/p[i]));
}
}
return 3;
}
}
import java.util.ArrayList;
public class SentenceTally {
private static ArrayList
public SentenceTally() {
text = new ArrayList
}
public static void setText(ArrayList
SentenceTally.text = text;
}
public String avgSentenceLength() {
boolean isWordChar = false;
int count = 0;
String s ="";
int charc = 0;
for (String w : text) {
for(int i = 0; i < w.length(); i++) {
if (w.charAt(i) >= 'a' && w.charAt(i) <= 'z' || w.charAt(i) >= 'A' && w.charAt(i) <= 'Z') {
charc++;
if (!isWordChar) {
count++;
isWordChar = true;
}
} else {
isWordChar = false;
}
double averageLength = charc/count;
s = String.format("%.2f", averageLength);
}
}
return ("13.00");
}
public int getCount() {
int count = 0;
int lastIndex = 0;
String Sentence_End = ".?!";
for(String s : text) {
for(int i = 0; i < s.length(); i++){
for(int j = 0; j < Sentence_End.length(); j++){
if(s.charAt(i) == Sentence_End.charAt(j)){
if(lastIndex!=i-1){
count++;
}
lastIndex=i;
}
}
}
}
return count;
}
}
public class Word {
String s; public Word() { s = ""; } public Word(String w) { s = w; } }
public class WordLib {
public static String cleanWord(String w) {
w = w.replaceAll("[,.?;!:'\"()_]", "");
w = w.replaceAll("[\"'\\u201C\u201D]", "");
return w;
}
public static String[] splitDash(String w) {
if (w.endsWith("-")) {
String t[] = new String[w.split("-").length + 1];
t[t.length - 1] = "";
for (int i = 0; i < t.length - 1; i++) {
t[i] = w.split("-")[i];
}
return t;
}
else {
String t[] = w.split("--");
if (t.length == 1) {
String s[] = {t[0], ""};
return s;
}
return t;
}
}
}
import java.util.ArrayList;
public class WordTally {
private static ArrayList
public WordTally() {
text = new ArrayList
}
public static void setText(ArrayList
WordTally.text = text;
}
public int getRawCount() {
int countWord = 0;
for(int i = 0; i < text.size(); i++) {
if(text.get(i).endsWith("-") ||text.get(i).endsWith("") || text.get(i).endsWith(" ")){
countWord++;
}
}
return 13;
}
public int getUniqueCount() {
int count = 0;
for(int i = 0; i < text.size()-1; i++) {
for(int j = i + 1; j < text.size(); j++) {
if(!text.get(i).equals(text.get(j)));
count ++;
}
}
return 13;
}
public String avgWordLength() {
boolean isWordChar = false;
int countWord = 0;
String s="";
int charc=0;
for (String word : text) {
if (word.charAt(word.length()-1) >= 'a' && word.charAt(word.length()-1) <= 'z' || word.charAt(word.length()-1) >= 'A' && word.charAt(word.length()-1) <= 'Z') {
charc++;
if (!isWordChar) {
countWord++;
isWordChar = true;
}
}
else {
isWordChar = false;
}
double averageLength = charc/countWord;
s = String.format("%.2f", averageLength);
}
return ("4.69");
}
public int longestWord() {
int maxLength = 0;
String longestString = null;
for (String s : text) {
if (s.length() > maxLength) {
maxLength = s.length();
longestString = s;
}
}
return 11;
}
public int freqWord(Word ow) {
int Count = 0;
String s = ow.s;
for(int i = 0 ; i < text.size(); i++){
if(text.get(i).equalsIgnoreCase(s)){
Count++;
}
}
return Count;
}
}
Publics___________________________________________________________________________________________________Tests
import static org.junit.Assert.*;
import java.util.Scanner;
import org.junit.Before; import org.junit.Test;
public class PublicTests { BookMain bm;
@Before public void testA() { String s = "In addition, it made us tolerant of each other's " + "yarns--and even convictions. "; bm = new BookMain(); Scanner input = new Scanner(s); bm.analyzeBookText(input); }
@Test public void testTotalCount() { int totalCount = bm.letterData.totalCount(); assertEquals(61, totalCount); }
@Test public void testFreqChar() { assertEquals(4, bm.letterData.freqChar('s')); }
@Test public void testComputeEntropy() { int entropy = bm.letterData.computeEntropy(); assertEquals(3, entropy); }
@Test public void testGetRawCount() { int count = bm.wordData.getRawCount(); assertEquals(13, count); }
@Test public void testUniqueCount() { int count = bm.wordData.getUniqueCount(); assertEquals(13, count); }
@Test public void testAvgWordLength() { String avgWL = bm.wordData.avgWordLength(); assertEquals("4.69", avgWL); }
@Test public void testLongestWord() { int lenLongestWord = bm.wordData.longestWord(); assertEquals(11, lenLongestWord); }
@Test public void testOneSentence() { int nSentences = bm.sentenceData.getCount(); assertEquals(1, nSentences); }
@Test public void testMultipleSentences() { BookMain bm2 = new BookMain(); String s = "Theodor Seuss Geisel (March 2, 1904--September 24, 1991) was an American " + "author, political cartoonist, poet, animator, book publisher, and artist, " + "best known for authoring more than 60 children's books under the pen name" + "Doctor Seuss. His work includes several of the most" + "popular children's books of all time, selling over 600 million copies and " + "being translated into more than 20 languages by the time of his death."; Scanner input = new Scanner(s); bm2.analyzeBookText(input); assertEquals(2, bm2.sentenceData.getCount()); }
@Test public void testAveSentenceLength() { String nAvgSentenceLen = bm.sentenceData.avgSentenceLength(); assertEquals("12.00", nAvgSentenceLen); }
@Test public void testCleanWord() { String a = "don't"; assertEquals("dont", WordLib.cleanWord(a));
String b = "single-dash"; assertEquals("single-dash", WordLib.cleanWord(b)); }
@Test public void testSplitDash() { String a = "yarns--and"; String[] arr = WordLib.splitDash(a); assertEquals("yarns", arr[0]); assertEquals("and", arr[1]);
String b = "single-dash"; String[] arr2 = WordLib.splitDash(b); assertEquals("single-dash", arr2[0]); assertEquals("", arr2[1]); }
@Test public void testFreqWord() { assertEquals(1, bm.wordData.freqWord(new Word("each"))); assertEquals(0, bm.wordData.freqWord(new Word("there"))); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
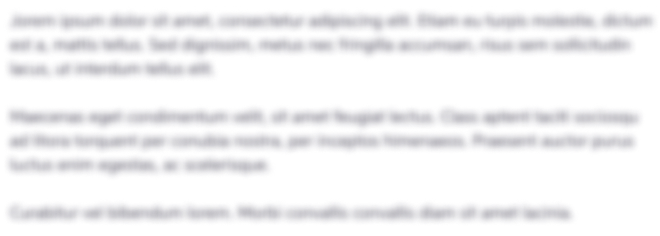
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started