Question
import java.util.ArrayList; import java.util.Scanner; public class Driver { private static ArrayList dogList = new ArrayList (); private static ArrayList monkeyList = new ArrayList (); public
import java.util.ArrayList; import java.util.Scanner;
public class Driver { private static ArrayList
public static void main(String[] args) { Scanner input = new Scanner(System.in);
initializeDogList(); initializeMonkeyList();
// Add a loop that displays the menu, accepts the users input // and takes the appropriate action. // For the project submission you must also include input validation // and appropriate feedback to the user. // Hint: create a Scanner and pass it to the necessary // methods // Hint: Menu options 4, 5, and 6 should all connect to the printAnimals() method. while(true) { displayMenu(); int choice = input.nextInt(); input.nextLine(); if(choice == 1) intakeNewDog(input); else if(choice == 2) intakeNewMonkey(input); else if(choice == 3) reserveAnimal(input); else if(choice == 4) printAnimals("dog"); else if(choice == 5) printAnimals("monkey"); else if(choice == 6) printAnimals("available"); else if(choice == 'q') System.exit(0); else System.out.println("Wrong choice"); } }
// This method prints the menu options public static void displayMenu() { System.out.println(" "); System.out.println("\t\t\t\tRescue Animal System Menu"); System.out.println("[1] Intake a new dog"); System.out.println("[2] Intake a new monkey"); System.out.println("[3] Reserve an animal"); System.out.println("[4] Print a list of all dogs"); System.out.println("[5] Print a list of all monkeys"); System.out.println("[6] Print a list of all animals that are not reserved"); System.out.println("[q] Quit application"); System.out.println(); System.out.println("Enter a menu selection"); }
// Adds dogs to a list for testing public static void initializeDogList() { Dog dog1 = new Dog("Spot", "German Shepherd", "male", "1", "25.6", "05-12-2019", "United States", "intake", false, "United States"); Dog dog2 = new Dog("Rex", "Great Dane", "male", "3", "35.2", "02-03-2020", "United States", "Phase I", false, "United States"); Dog dog3 = new Dog("Bella", "Chihuahua", "female", "4", "25.6", "12-12-2019", "Canada", "in service", true, "Canada");
dogList.add(dog1); dogList.add(dog2); dogList.add(dog3); }
// Adds monkeys to a list for testing //Optional for testing public static void initializeMonkeyList() { Monkey monkey1 = new Monkey("Drek","male","5", "450", "Mandril", "2.3", "1.4", "4.5", "12-12-2019", "United States", "in service", false, "United states"); monkeyList.add(monkey1); }
// Complete the intakeNewDog method // The input validation to check that the dog is not already in the list // is done for you public static void intakeNewDog(Scanner scanner) { System.out.println("What is the dog's name? "); String name = scanner.nextLine(); for(Dog dog: dogList) { if(dog.getName().equalsIgnoreCase(name)) { System.out.println(" This dog is already in our system "); return; //returns to menu } } // Add the code to instantiate a new dog and add it to the appropriate list System.out.print("Breed: "); String breed = scanner.nextLine(); System.out.print("Gender (male/female): "); String gender = scanner.nextLine(); System.out.print("Age (in years): "); String age = scanner.nextLine(); System.out.print("Weight: "); String weight = scanner.nextLine(); System.out.print("Acquisition date: "); String acqDate = scanner.nextLine(); System.out.print("Acquisition country: "); String country1 = scanner.nextLine(); System.out.print("Training status: "); String status = scanner.nextLine(); System.out.print("Reserved (1 for true, 0 for false): "); String choice = scanner.nextLine(); boolean reserved; if(choice.equals("1")) reserved = true; else reserved = false; System.out.println("In service country: "); String country2 = scanner.nextLine(); Dog newDog = new Dog(name, breed, gender, age, weight, acqDate, country1, status, reserved, country2); dogList.add(newDog); }
I have to short the paragraph because it reached max length.
Here are some feedbacks I have received after I checked the code on instant feedback. I guess i need help an Array list for Monkey object.
Feedback
Your Driver class only has one Array List for Dogs. You should also have an Array List for Monkey objects.
Feedback
You used an if-else structure to process the user input. Good job with that! It's also useful to learn how switch case works for problems like this.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
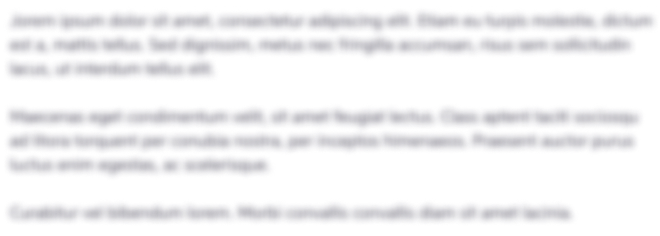
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started