Question
import java.util.Iterator; import java.util.NoSuchElementException; public class CiscArrayList implements CiscList { //ArrayList myList = new ArrayList ; /** * Default initial capacity of elementData. */ private
import java.util.Iterator; import java.util.NoSuchElementException; public class CiscArrayList implements CiscList { //ArrayList myList = new ArrayList<>; /** * Default initial capacity of elementData. */ private static final int DEFAULT_CAPACITY = 10; /** * The array into which the elements of the CiscArrayList are stored. */ private E[] elementData; /** * The size of the CiscArrayList (the number of elements it contains). */ private int size; /** * Constructs an empty list with an initial capacity of DEFAULT_CAPACITY. */ public CiscArrayList() { this(DEFAULT_CAPACITY); } /** * Constructs an empty list with the specified initial capacity. * * @param initialCapacity the initial capacity of the list * @throws IllegalArgumentException if the specified initial capacity is negative */ public CiscArrayList(int initialCapacity) { if(initialCapacity < 0){ throw new IllegalArgumentException(); } elementData = (E[])new Object[initialCapacity]; size = 0; } /** * Returns the number of elements in this list. * * * @return the number of elements in this list */ @Override public int size() { return size; } /** * Returns {@code true} if this list contains no elements. * * * @return {@code true} if this list contains no elements */ @Override public boolean isEmpty() { return size ==0; } /** * Returns {@code true} if this list contains the specified element (compared using the {@code equals} method). * * * @param o element whose presence in this list is to be tested * @return {@code true} if this list contains the specified element * @throws NullPointerException if the specified element is null */ @Override public boolean contains(Object o) { if(o == null){ throw new NullPointerException(); } for(int i=0; i= size){ throw new IndexOutOfBoundsException(); } return elementData[index]; } /** * Appends the specified element to the end of this list. * * *
Lists may place the specified element at arbitrary locations if desired. In particular, an ordered list will * insert the specified element at its sorted location. List classes should clearly specify in their documentation * how elements will be added to the list if different from the default behavior (end of this list). * * @param e element to be appended to this list * @return {@code true} * @throws NullPointerException if the specified element is null */// @Override public boolean add(E e) { if (e == null){ throw new NullPointerException(); } int i = 0; for (i = 0; i< size; i++){ if (elementData[i].equals(e)){ break; } if (i< size){ for(int k = i+1; k= size) { throw new IndexOutOfBoundsException(); } if (element == null) { throw new NullPointerException(); } E old = elementData[index]; elementData[index] = element; return old; } /** * Returns an array containing all of the elements in this list in proper sequence (from first to last element). * * *
The returned array will be "safe" in that no references to it are maintained by this list. (In other words, * this method must allocate a new array even if this list is backed by an array). The caller is thus free to modify * the returned array. * * @return an array containing all of the elements in this list in proper sequence */ @Override public Object[] toArray() { Object[]array = new Object[size]; for (int i =0; i= size) { throw new IndexOutOfBoundsException(); } E e = elementData[index]; for(int j = index; j size) { throw new IndexOutOfBoundsException(); } if (element == null) { throw new NullPointerException(); } for (int i = 0; i < element.index(); i++){ add(index++, element.get(i)); } return true; } /** * Appends all elements in the specified list to the end of this list, in the order that they are returned by the * specified list's iterator (optional operation). * * * @param c list containing elements to be added to this list * @return {@code true} if this list changed as a result of the call * @throws UnsupportedOperationException if the {@code addAll} operation is not supported by this list * @throws NullPointerException if the specified list is null */ @Override public boolean addAll(CiscList c) { if (elementData == null){ throw new NullPointerException(); } for(int i = 0; i < c.size(); i++){ add(c.get(i)); } return true; } /** * Returns an iterator over the elements in this list in proper sequence. * * * @return an iterator over the elements in this list in proper sequence */ @Override public Iterator iterator() { return new CiscArrayListIterator(); } /** * Ensures that the capacity of the underlying array is at least {@code minCapacity}. If not, the underlying array * will be replaced with a new array whose capacity is either {@code minCapacity} or double the current capacity + 1 * (whichever is larger). * * * @param minCapacity minimum capacity of the underlying array */ private void ensureCapacity(int minCapacity) { } private class CiscArrayListIterator implements Iterator { /** * Stores the index of the next element to be returned. */ int nextIndex = -1; public Object nextindex() { nextIndex++; return elementData[nextIndex]; } /** * Returns {@code true} if the iteration has more elements. (In other words, returns {@code true} if * {@link #next} would return an element rather than throwing an exception.) * * @return {@code true} if the iteration has more elements */ @Override public boolean hasNext() { return nextIndex +1 < size; } /** * Returns the next element in the iteration. * * @return the next element in the iteration * @throws NoSuchElementException if the iteration has no more elements */ @Override public E next() { return null; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
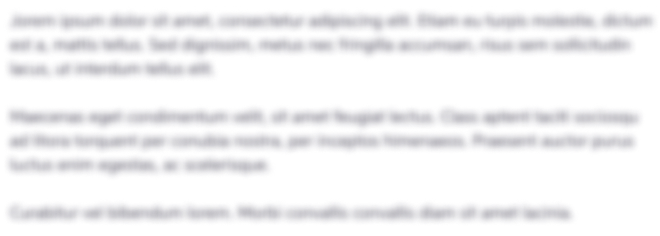
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started