Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.Iterator; public class KeyedListWithIterators implements KeyedListWithIteratorsInterface { private Node firstNode, lastNode; private int numEntries; public KeyedListWithIterators() { firstNode = lastNode = null; numEntries =
import java.util.Iterator; public class KeyedListWithIteratorsimplements KeyedListWithIteratorsInterface { private Node firstNode, lastNode; private int numEntries; public KeyedListWithIterators() { firstNode = lastNode = null; numEntries = 0; } /* * returns the Node with the given key, * or null if key is not present * * @param key the given key * @return the Node with key or null */ private Node findKeyed(K key) { Node curr = firstNode; while (curr != null) { if (key.equals(curr.key)) { break; } curr = curr.next; } // end while return curr; } // end findKeyed /** * Adds the key/value pair to the list if the key * is not yet present and returns null * Otherwise, replaces the key's current value, * and returns the current value * * @param key the given key * @param value the new value for the key * @return the key's current value or null */ @Override public V add(K key, V newValue) { V oldValue = null; if (isEmpty()) { firstNode = lastNode = new Node(key, newValue); numEntries++; } else { // if key in list, replace value // otherwise, add key/value pair to end Node keyNode = findKeyed(key); if (keyNode != null) { // replace value in currNode oldValue = keyNode.value; keyNode.value = newValue; } else { // add to end of list Node newNode = new Node(key, newValue); lastNode.next = newNode; lastNode = newNode; numEntries++; } // end inner if/else } // end outer if/else return oldValue; } // end add /** * returns the value with the given key * returns null if the key is not present * * @param key the given key * @return the key's current value or null */ @Override public V get(K key) { V value = null; Node keyNode = findKeyed(key); if (keyNode != null) { value = keyNode.value; } return value; } // end get /* * returns the Node prior to the Node * with the given key, * or null if key is not present * * @param key the given key * @return the Node prior to the key or null */ private Node findB4Keyed(K key) { Node curr = firstNode; Node prev = null; while (curr != null) { if (key.equals(curr.key)) { break; } prev = curr; curr = curr.next; } // end while return prev; } // end findB4Keyed /** * removes and returns the value with the given key * returns null if the key is not present * * @param key the given key * @return the key's current value or null */ @Override public V remove(K key) { V value = null; if (isEmpty()) { return null; } Node prevNode = findB4Keyed(key); if (prevNode != null) { Node currNode = prevNode.next; if (currNode != null && key.equals(currNode.key)) { // currNode contains key value = currNode.value; prevNode.next = currNode.next; numEntries--; } } else { if (key.equals(firstNode.key)) { // key is in first node value = firstNode.value; firstNode = firstNode.next; if (firstNode == null) { lastNode = null; } numEntries--; } } // end outer if/else return value; } // end remove /** * indicates whether the list is empty * * @return true is list is empty, false otherwise */ @Override public boolean isEmpty() { return numEntries == 0; } /** * removes all nodes from the list */ @Override public void clear() { firstNode = lastNode = null; System.gc(); numEntries = 0; } // end clear /** * Returns an iterator for the keys * * @return Iterator for the keys in the list */ @Override public Iterator keyIterator() { return new KeyIterator (); } /** * Returns an iterator for the values * * @return Iterator for the values in the list */ @Override public Iterator valueIterator() { return new ValueIterator (); } /** * returns the list as a string of format: * [ (k1,v1) (k2,v2) ... (kn,vn) ] */ @Override public String toString() { StringBuilder sb = new StringBuilder("[ "); Node curr = firstNode; while (curr != null) { sb.append(curr); sb.append(" "); curr = curr.next; } sb.append("]"); return sb.toString(); } // end toString //================================================================================================== private class Node { K key; V value; Node next; Node(K key, V value, Node next) { this.key = key; this.value = value; this.next = next; } Node(K key, V value) { this(key, value, null); } /** * returns the key/value pair as a string */ @Override public String toString() { return "("+key+","+value+")"; } } // end Node class //================================================================================================ class KeyIterator implements Iterator { private Node curr; public KeyIterator() { curr = firstNode; } /** * indicates whether there's another node * * @return false if past end of list, true otherwise */ @Override public boolean hasNext() { return curr != null; } // end hasNext /** * returns key in curr and moves curr to next node * returns null if past end of list */ @Override @SuppressWarnings("unchecked") public K1 next() { K1 nextKey = null; if (hasNext()) { nextKey = (K1)curr.key; curr = curr.next; } return nextKey; } // end next @Override public void remove() { throw new UnsupportedOperationException("remove method not implemented for this class"); } // end remove } // end KeyIterator //================================================================================================ class ValueIterator implements Iterator { private Node curr; public ValueIterator() { curr = firstNode; } /** * indicates whether there's another node * * @return false if past end of list, true otherwise */ @Override public boolean hasNext() { return curr != null; } // end hasNext /** * returns value in curr and moves curr to next node * returns null if past end of list */ @Override @SuppressWarnings("unchecked") public V1 next() { V1 nextValue = null; if (hasNext()) { nextValue = (V1)curr.value; curr = curr.next; } return nextValue; } // end next @Override public void remove() { throw new UnsupportedOperationException("remove method not implemented for this class"); } // end remove } // end ValueIterator } // end KeyedListWithIterators generic
Use the KeyedListWithIterators.java generic develop a SortedListWithIterators.java generic.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
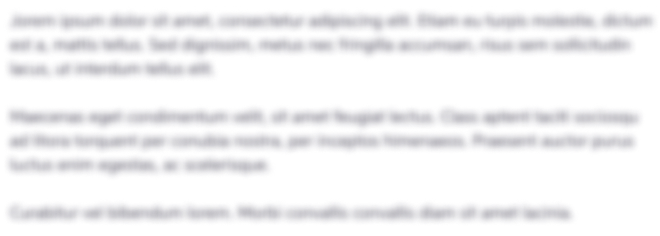
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started