Question
import java.util.Scanner; public class Coins { // TODO: add class components with corresponding comment labels public static void main(String[] args) { Scanner keyboard = new
import java.util.Scanner;
public class Coins { // TODO: add class components with corresponding comment labels public static void main(String[] args) { Scanner keyboard = new Scanner(System.in);
int getQuarters; // Number of quarters, to be input by the user. int getDimes; // Number of dimes, to be input by the user. int getNickles; // Number of nickles, to be input by the user. int getPennies; // Number of pennies, to be input by the user.
double findCentsValue; // Total value of all the coins, in dollars.
/* Ask the user for the number of each type of coin. */
System.out.println("Enter the number of quarters: "); getQuarters = keyboard.nextInt();
System.out.println("Enter the number of dimes: "); getDimes = keyboard.nextInt();
System.out.println("Enter the number of nickles: "); getNickles = keyboard.nextInt();
System.out.println("Enter the number of pennies: "); getPennies = keyboard.nextInt();
/* Add up the values of the coins, in dollars. */
findCentsValue = (0.25 * getQuarters) + (0.10 * getDimes) + (0.05 * getNickles) + (0.01 * getPennies);
/* Report the result back to the user. */
System.out.println("The total in dollars is $" + findCentsValue);
} }
Make the following modifications to the Coins class:
(1) Add a default constructor that takes no parameters and initializes all the fields to 0 except for 1 penny.
(2) Add a single parameter constructor that takes a double as a total starting amount in dollars and calculates the number of each denomination of coin. To accomplish this, you will need to convert the parameter to an integer number of cents and then repeatedly use integer division starting with the largest denomination (quarters) to determine the maximum number of each denomination this amount contains. Be sure to remove the amount of each denomination from the total before calculating the number of coins in the next smaller denomination.
(3) Add a single parameter constructor that takes a Coins object and initializes the fields to the same values as the parameter object. Note: This is essentially making a copy of the parameter object. It is good practice to use the getter methods for the parameter object, however since the object is in the same class, the fields can actually be accessed directly.
(4) Add an equals method that takes a Coins object as a parameter and returns a boolean. The method should return true if all the fields of the class are equal to those of the parameter, and false otherwise.
Make the following modifications to the CoinsTest class:
(1) Add several additional test Coins references.
(2) In the setUp method, instantiate at least one new Coins object using each of the new constructors.
(3) Add assert tests in the various getter test methods to check for proper initialization of the fields in the new test objects. Note: For the object instantiated using the Coins object parameter, use an assertTrue test that checks both that the field values are equal and the references for the new object and the one passed to the constructor are different.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
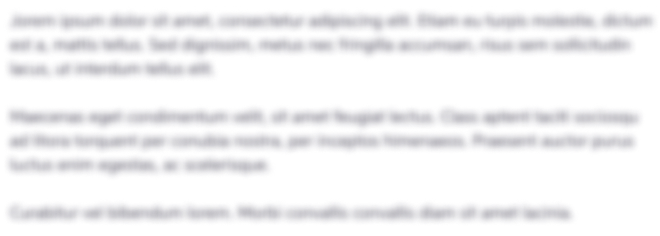
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started