Question
================================================================== import java.util.Scanner; public class Hex2Dec { private String hex;// NULL public Hex2Dec(String hexStr){ hex = hexStr.toUpperCase(); } // accessor or get method for member
==================================================================
import java.util.Scanner; public class Hex2Dec { private String hex;// NULL public Hex2Dec(String hexStr){ hex = hexStr.toUpperCase(); } // accessor or get method for member variable hex public String getHex(){return hex;} // mutator or set method for member variable hex public void setHex(String newHex){hex = newHex.toUpperCase();} private int hexToDecimal() { int decimalValue = 0; for (int i = 0; i < hex.length(); i++) { char hexChar = hex.charAt(i); decimalValue = decimalValue * 16 + hexCharToDecimal(hexChar); } return decimalValue; }
public int hexCharToDecimal(char ch) { if (ch >= 'A' && ch <= 'F') return 10 + ch - 'A'; else if (ch >= '0' && ch <= '9'){// ch is '0', '1', ..., or '9' return ch - '0'; } return 0; } @Override public String toString(){ return "Hex: " + hex + "; Decimal: " + hexToDecimal(); } public static void main(String[] args) { // Create a Scanner Scanner input = new Scanner(System.in); // Prompt the user to enter a string System.out.print("Enter a hex number: "); String hex = input.nextLine(); Hex2Dec hd = new Hex2Dec(hex); System.out.println("The decimal value for hex number " + hex + " is " + hd.hexToDecimal()); System.out.print("Enter a hex number again: "); hd.setHex(input.nextLine()); System.out.println(hd); } }
====================================================================
mport java.util.Scanner;
public class PrintCalendar { /** Main method * @param args */ public static void main(String[] args) { Scanner input = new Scanner(System.in);
// Prompt the user to enter year System.out.print("Enter full year (e.g., 2001): "); int year = input.nextInt();
// Prompt the user to enter month System.out.print("Enter month in number between 1 and 12: "); int month = input.nextInt();
// Print calendar for the month of the year printMonth(year, month); }
/** Print the calendar for a month in a year * @param year * @param month */ public static void printMonth(int year, int month) { // Print the headings of the calendar printMonthTitle(year, month);
// Print the body of the calendar printMonthBody(year, month); }
/** Print the month title, e.g., May, 1999 * @param year * @param month */ public static void printMonthTitle(int year, int month) { System.out.println(" " + getMonthName(month) + " " + year); System.out.println("-----------------------------"); System.out.println(" Sun Mon Tue Wed Thu Fri Sat"); }
/** Get the English name for the month * @param month * @return */ public static String getMonthName(int month) { String monthName = ""; switch (month) { case 1 -> monthName = "January"; case 2 -> monthName = "February"; case 3 -> monthName = "March"; case 4 -> monthName = "April"; case 5 -> monthName = "May"; case 6 -> monthName = "June"; case 7 -> monthName = "July"; case 8 -> monthName = "August"; case 9 -> monthName = "September"; case 10 -> monthName = "October"; case 11 -> monthName = "November"; case 12 -> monthName = "December"; }
return monthName; }
/** Print month body * @param year * @param month */ public static void printMonthBody(int year, int month) { // Get start day of the week for the first date in the month int startDay = getStartDay(year, month);
// Get number of days in the month int numberOfDaysInMonth = getNumberOfDaysInMonth(year, month);
// Pad space before the first day of the month int i = 0; for (i = 0; i < startDay; i++) System.out.print(" ");
for (i = 1; i <= numberOfDaysInMonth; i++) { System.out.printf("%4d", i);
if ((i + startDay) % 7 == 0) System.out.println(); }
System.out.println(); }
/** Get the start day of month/1/year * @param year * @param month * @return */ public static int getStartDay(int year, int month) { final int START_DAY_FOR_JAN_1_1800 = 3; // Get total number of days from 1/1/1800 to month/1/year int totalNumberOfDays = getTotalNumberOfDays(year, month);
// Return the start day for month/1/year return (totalNumberOfDays + START_DAY_FOR_JAN_1_1800) % 7; }
/** Get the total number of days since January 1, 1800 * @param year * @param month * @return */ public static int getTotalNumberOfDays(int year, int month) { int total = 0;
// Get the total days from 1800 to 1/1/year for (int i = 1800; i < year; i++) if (isLeapYear(i)) total = total + 366; else total = total + 365;
// Add days from Jan to the month prior to the calendar month for (int i = 1; i < month; i++) total = total + getNumberOfDaysInMonth(year, i);
return total; }
/** Get the number of days in a month * @param year * @param month * @return */ public static int getNumberOfDaysInMonth(int year, int month) { if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12) return 31;
if (month == 4 || month == 6 || month == 9 || month == 11) return 30;
if (month == 2) return isLeapYear(year) ? 29 : 28;
return 0; // If month is incorrect }
/** Determine if it is a leap year * @param year * @return */ public static boolean isLeapYear(int year) { return year % 400 == 0 || (year % 4 == 0 && year % 100 != 0); } }
******************************************************************************************************************
Question:
Redesign PrintCalendar classes in the form of Hex2Dec class above.
******************************************************************************************************************
Step by Step Solution
There are 3 Steps involved in it
Step: 1
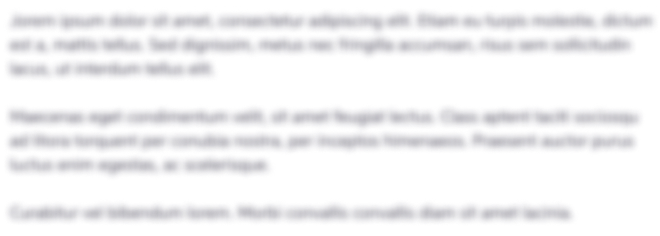
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started