Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.Scanner; /** * The Circle class defines a circle specified in double precision. * */ public class Circle { /** * The circle's immutable
import java.util.Scanner;
/**
* The Circle class defines a circle specified in double precision.
*
*/
public class Circle {
/**
* The circle's immutable radius.
*/
private final double radius;
/**
* Constructs a new Circle with the specified radius
*
* @param radius the radius of the circle
*/
public Circle(double radius) {
this.radius = radius;
}
/**
* Returns the radius of this Circle in double precision.
*
* @return the radius of this Circle.
*/
public double getRadius() {
return radius;
}
/**
* Returns the diameter of this Circle in double precision.
*
* @return the diameter of this Circle.
*/
public double getDiameter() {
return 2 * 3.1415926 * radius;
}
/**
* Returns the area of this Circle in double precision.
*
* @return the area of this Circle.
*/
public double getArea() {
return 3.1415926 * radius * radius;
}
/**
* Returns the length of an arc for this Circle given an angle specified in radians.
*
* @param theta the angle in radians.
* @return the arc length for the given angle.
*/
public double getRadiansArcLength(double theta) {
return radius * theta;
}
/**
* Returns the length of an arc for this Circle given an angle specified in degrees.
*
* @param alpha the angle in degrees.
* @return the arc length for the given angle.
*/
public double getDegreesArcLength(double alpha) {
return 3.1415926 * radius * alpha / 180;
}
/**
* Returns the area of a sector for this Circle given an angle specified in radians.
*
* @param theta the angle in radians.
* @return the sector area for the given angle.
*/
public double getRadiansSectorArea(double theta) {
return radius * radius * theta / 2;
}
/**
* Returns the area of a sector for this Circle given an angle specified in degrees.
*
* @param alpha the angle in degrees.
* @return the sector area for the given angle.
*/
public double getDegreesSectorArea(double alpha) {
return 3.1415926 * radius * radius * alpha / 360;
}
/**
* Main method that tests the calculation of Circle properties.
*
* @param args the unused command line arguments.
*/
public static void main(String[] args) {
System.out.println("------------------------------------------------------------");
System.out.println("CircleCalc v1.0");
System.out.println();
System.out.println("Calculates and prints information for a user-supplied radius");
System.out.println("------------------------------------------------------------");
System.out.print("Enter the circle's radius: ");
Scanner in = new Scanner(System.in);
Circle c = new Circle(in.nextDouble());
System.out.println();
System.out.println("Circle properties:");
System.out.println("\tRadius: " + c.getRadius());
System.out.println("\tDiameter: " + c.getDiameter());
System.out.println("\tArea: " + c.getDiameter());
System.out.println("\tArc length at 1.57 radian: " + c.getRadiansArcLength(1.57));
System.out.println("\tArc length at 90 degrees: " + c.getDegreesArcLength(90));
System.out.println("\tSector area at 1.57 radian: " + c.getRadiansSectorArea(1.57));
System.out.println("\tSector area at 90 degrees: " + c.getDegreesSectorArea(90));
}
}
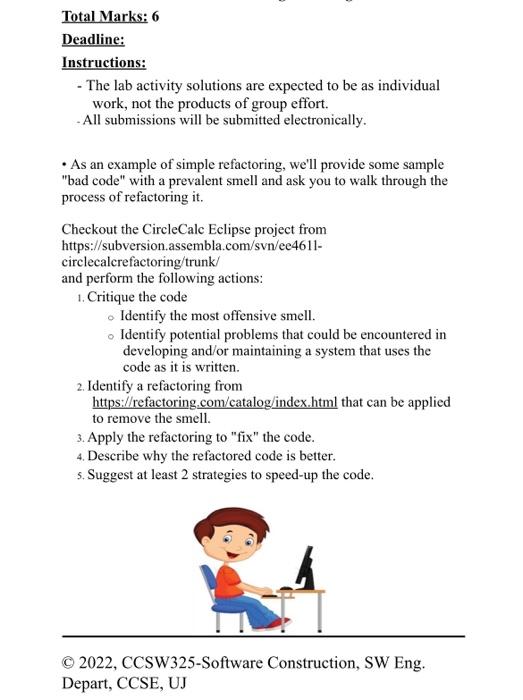
Step by Step Solution
There are 3 Steps involved in it
Step: 1
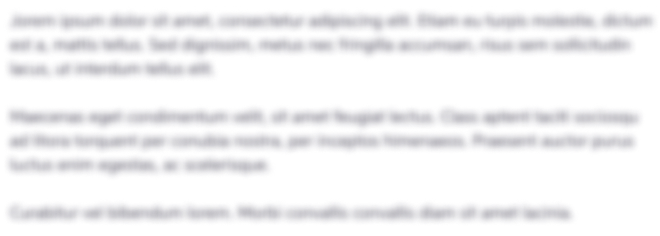
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started