Question
import java.util.Scanner; /* This application manages a collection of media items for a user. The items * are representeed by MediaItem objects. The items are
import java.util.Scanner; /* This application manages a collection of media items for a user. The items * are representeed by MediaItem objects. The items are managed by a MediaList object. * Their are currently three types of MediaItems: books (electronic format), movies, and songs. * Each type is modeled as a subclass of MediaItem. * A user has several options that are displayed in a menu format. * This class runs a console interface between a user and the mediaList */ import java.io.*;
public class LibraryMain { public static void main(String[] args) throws IOException{ System.out.println("My Media Library"); Scanner scan = new Scanner(System.in); MediaList mediaList = new MediaList(5); boolean keepGoing = true; String userStr = ""; int position; while(keepGoing) { System.out.println("Main Menu:"); System.out.println("Enter A to add a new media item."); System.out.println("Enter F to find media items."); System.out.println("Enter P to view all media items."); System.out.println("Enter X to quit."); System.out.println(""); userStr = scan.nextLine(); if (userStr.equalsIgnoreCase("A")){ MediaItem item = null; System.out.println("Enter the title: "); String title = scan.nextLine(); System.out.println("Enter the author/artist/director: "); String author = scan.nextLine(); System.out.println("Enter the genre: "); String genre = scan.nextLine(); System.out.println("What type of media? Enter B for book, M for movie, S for song: "); String type = scan.nextLine(); if(type.equalsIgnoreCase("B")){ System.out.println("Enter the number of pages: "); String pages = scan.nextLine(); System.out.println("Enter the preferred font size: "); String font = scan.nextLine(); item = new Book(title, author, genre, Integer.parseInt(pages), Double.parseDouble(font)); } else if(type.equalsIgnoreCase("M")){ System.out.println("Enter the playing time (minutes): "); String playTime = scan.nextLine(); System.out.println("Enter the lead actor: "); String actor = scan.nextLine(); System.out.println("Enter the release year YYYY: "); String year = scan.nextLine(); item = new Movie(title, author, genre, Integer.parseInt(playTime), actor, year); } else if(type.equalsIgnoreCase("S")){ System.out.println("Enter the playing time (minutes): "); String playTime = scan.nextLine(); System.out.println("Enter the album: "); String album = scan.nextLine(); System.out.println("Enter the label: "); String label = scan.nextLine(); item = new Song(title, author, genre, Double.parseDouble(playTime), album, label); } else System.out.println("Unrecognized type."); mediaList.addItem(item); } else if (userStr.equalsIgnoreCase("F")){ System.out.println("Enter T to search by title, G to search by genre:"); userStr = scan.nextLine(); if(userStr.equalsIgnoreCase("T")){ System.out.println("Enter the title:"); String title = scan.nextLine(); MediaItem[] items = mediaList.getItemsByTitle(title); if(items.length>0){ String listStr = getItemListAsString(items); System.out.println(listStr); } else System.out.println("Could not find "+title+" in the list."); } else if(userStr.equalsIgnoreCase("G")){ System.out.println("Enter the genre:"); String genre = scan.nextLine(); MediaItem[] items = mediaList.getItemsByGenre(genre); if(items.length>0){ String listStr = getItemListAsString(items); System.out.println(listStr); } else System.out.println("Could not find "+genre+" in the list."); } else System.out.println("Unrecognized search type."); } else if (userStr.equalsIgnoreCase("P")){ System.out.println("Your media: "); MediaItem[] items = mediaList.getItemList(); String listStr = getItemListAsString(items); System.out.println(listStr); } else if(userStr.equalsIgnoreCase("X")) keepGoing = false; else System.out.println("Unrecognized input."); } System.out.println("Bye for now."); scan.close(); } /* This method returns a String which consists of the String * representations of all MediaItems in the array passed in. * Assume the itemList does not contain NULL values. * Assume the itemList is not null, and length >=0. */ public static String getItemListAsString(MediaItem[] itemList){ StringBuilder sb = new StringBuilder(); if(itemList.length>0){ for(int i=0; i ---------------------------------------------------------------------------------------------------------------------------- /** * This class encapsulates the data required to represent a MediaItem. * The attributes common to all MediaItems are: title, author and genre. * Subclasses should override the toString method. **/ public class MediaItem { private String title; private String author; private String genre; /* Subclasses may add specific parameters to their constructor's * parameter lists. */ public MediaItem(String title, String author, String genre){ this.title = title; this.author = author; this.genre = genre; } // get method for the title public String getTitle(){ return title; } // get method for the author public String getAuthor(){ return author; } // get method for the genre public String getGenre(){ return genre; } // Subclasses should override. public String toString(){ return title+", "+author+", "+genre; } } ------------------------------------------------------------------------------------------------------------------------------- /* This class encapsulates a list of media items in a user's collection. * The list is implemented as an array of type MediaItem. * Media items are either a book (electronic format), movie, or song. * Each type of media item is represented by an instance of the Book, Movie, or Song class. * These three classes are subclasses of MediaItem. The array stores media items as * references of type MediaItem. * * It is recommended that you maintain an int variable to keep track of the number of media items * on the array. */ public class MediaList { //Class member variable declarations: must include at least an array of type MediaItem. /* Constructor that initializes the member variables. The array is * created using the initial length passed in to the constructor. * Any other member variables are initialized as well. */ public MediaList(int initialLen){ } /* Add the newItem passed in to the next available cell in the itemList. * Available cells have the vaue NULL. The numItems variable may be used * to keep track of the index of the next available cell. * For example, if the list contained: item1, item2, NULL, NULL, * the next available cell is at index 2. */ public void addItem(MediaItem newItem){ } /* This method returns an array that contains only MediaItem objects whose * title matches the targetTitle passed in. * The array returned does not contain any NULL values. * This method returns an array of length 0 if there are no matches. * This method may call the getOnlyItems method. */ public MediaItem[] getItemsByTitle(String targetTitle){ } /* This method returns an array that contains only MediaItem objects whose * genre matches the targetGenre passed in. * The array returned does not contain any NULL values. * This method returns an array of length 0 if there are no matches. * This method may call the getOnlyItems method. */ public MediaItem[] getItemsByGenre(String targetGenre){ } /* This method returns an array of all of the MediaItem objects that are * stored in the itemList. The array returned does not contain any NULL * values. This method returns an array of length 0 if the itemList is empty. * This method may call the getOnlyItems metho */ public MediaItem[] getItemList(){ } /* Returns the number of items stored in the itemList. */ public int getNumItems(){ } /* Returns true if the itemList contains no MediaItems, false otherwise. */ public boolean isEmpty(){ } /****** Private, "helper" method section ******/ /* Creates a new array that is double the size of the array passed in, copies the data * from that array to the new array, and returns the new array. * Note that the new array will contain the items from the previous array followed by NULL values. */ private MediaItem[] expandList(MediaItem[] inputList){ } /* A full item list is an array where all cells contain an item. That * means there is no cell that contains NULL. * This method returns true if all cells in the array contain a String * object, false otherwise. */ private boolean isFull(){ } /* * This method takes an array of MediaItems as an input as well as * the number of MediaItems on that array. The input array may contain * some NULL values. * This method returns an array that contains only the MediaItems in * the input array and no NULL values. * It returns an array of length 0 if there are no MediaItems in the input array. */ private MediaItem[] getOnlyItems(MediaItem[] inputArray, int size){ } } ------------------------------------------------------------------------------------------------------------------------ import org.junit.Assert; import static org.junit.Assert.*; import org.junit.Before; import org.junit.Test; import java.lang.reflect.*; public class MediaListTest { private MediaList mediaList; /** Fixture initialization (common initialization * for all tests). **/ @Before public void setUp() { mediaList = new MediaList(6); } @Test public void itemListNameTest() { Field[] fields = MediaList.class.getDeclaredFields(); boolean wasDeclared = false; for(int i=0;i if(fields[i].getName().equals("itemList")){ wasDeclared = true; } } assertEquals("Test 1: The instance variable itemList has not been declared.", true, wasDeclared); } /** Test for required instance variable initializations in constructor. **/ @Test public void itemListTypeTest() { Field[] fields = MediaList.class.getDeclaredFields(); String varType = null; for(int i=0;i if(fields[i].getName().equals("itemList")){ varType = fields[i].getType().getName(); } } assertEquals("Test 2: The instance variable itemList has not been declared as an int.", "[LMediaItem;", varType); } /** Test that the list has been created. **/ @Test public void initListTest() { MediaItem[] items = mediaList.getItemList(); assertNotEquals("Test 3: The array is null- list may not have been initialized.", null, items); } /** Test the addItem method. **/ @Test public void addOneItemTest() { MediaItem item = new Movie("Black Panther", "Coogler", "fantasy", 134, "Chadwick Boseman", "2018"); mediaList.addItem(item); MediaItem[] items = mediaList.getItemList(); assertEquals("Test 4: The item was not added.", item.toString(), items[0].toString()); } /** Test the addItem method-length of array. **/ @Test public void addOneItemLenOneTest() { mediaList.addItem(new Movie("Black Panther", "Coogler", "fantasy", 134, "Chadwick Boseman", "2018")); MediaItem[] items = mediaList.getItemList(); assertEquals("Test 5: Call to addItem- the list should contain one item.", 1, items.length); } /** Test the getNumberOfItems method on an empty list. **/ @Test public void initListSizeTest() { int len = mediaList.getNumItems(); assertEquals("Test 6: The getNumItems method should return 0 on an empty list.", 0, len); } /** Test the isEmpty method on a list with one item. **/ @Test public void getItemListEmptyTest() { MediaItem[] items = mediaList.getItemList(); assertEquals("Test 7: The getItemList method should return a zero length array on an empty itemList.", 0, items.length); } @Test public void initListIsEmptyTest() { boolean empty = mediaList.isEmpty(); assertEquals("Test 8: The isEmpty method should return true on an empty list.", true, empty); } @Test public void oneItemListSizeTest() { mediaList.addItem(new Movie("Black Panther", "Coogler", "fantasy", 134, "Chadwick Boseman", "2018")); int len = mediaList.getNumItems(); assertEquals("Test 9: The getNumItems method should return 1 on list with 1 item.", 1, len); } @Test public void listOneItemIsEmptyTest() { mediaList.addItem(new Movie("Black Panther", "Coogler", "fantasy", 134, "Chadwick Boseman", "2018")); boolean empty = mediaList.isEmpty(); assertEquals("Test 10: The isEmpty method should return false on a list with one item.", false, empty); } /* Test private method getOnlyItems. */ @Test public void expandListTest4()throws Exception { Class classToCall = Class.forName("MediaList"); MediaItem[] argu = {null, new Book("Goosebumps","R.L.Stine", "fiction",128,3.5), null, new Song("Humble","Kendrick Lamar","hip hop", 2.57,"Damn","Top Dawg"), null}; Method methodToExecute = classToCall.getDeclaredMethod("getOnlyItems", new Class[]{MediaItem[].class, Integer.TYPE}); methodToExecute.setAccessible(true); Object resultListObj = methodToExecute.invoke(mediaList, new Object[]{argu, 2}); MediaItem[] resultList = (MediaItem[])resultListObj; assertEquals("Test 11: The expandList method did not correctly copy the data from the array passed in.", 2, resultList.length); } /** Test the getItemsByTitle method with zero matches. **/ @Test public void getItemsByTitleZeroMatchesTest() { addSixMixedItems(mediaList); MediaItem[] items = mediaList.getItemsByTitle("No Title Matches This"); assertEquals("Test 12: Test the getItemsByTitle method with zero matches.", 0, items.length); } /** Test the getItemsByTitle method with two matches. **/ @Test public void getItemsByTitleTwoMatchesTest() { addSixMixedItems(mediaList); MediaItem[] items = mediaList.getItemsByTitle("Goosebumps"); assertEquals("Test 13: Test the getItemsByTitle method with 2 matches.", 2, items.length); } /** Test the getItemsByGenre method with zero matches. **/ @Test // @GradedTest(name = "Test 10: The isEmpty method should return true on an empty list.", max_score = 4.0) public void getItemsByGenreZeroMatchesTest() { addSixMixedItems(mediaList); MediaItem[] items = mediaList.getItemsByGenre("No Genre Matches This"); assertEquals("Test 14: Test the getItemsByGenre method with zero matches.", 0, items.length); } /** Test the getItemsByGenre method with two matches. **/ @Test public void getItemsByGenreTwoMatchesTest() { addSixMixedItems(mediaList); MediaItem[] items = mediaList.getItemsByGenre("sci fi"); assertEquals("Test 15: Test the getItemsByGenre method with 2 matches.", 2, items.length); } /* Test private method expandList on empty list of length 5. */ @Test public void expandListTest()throws Exception { Class classToCall = Class.forName("MediaList"); Method methodToExecute = classToCall.getDeclaredMethod("expandList", new Class[]{MediaItem[].class}); methodToExecute.setAccessible(true); MediaItem[] args = new MediaItem[5]; Object expandedListObj = methodToExecute.invoke(mediaList, new Object[]{args}); MediaItem[] expandedList = (MediaItem[])expandedListObj; int result = expandedList.length; assertEquals("Test 16: The expandList method did not create an array of double the length of the array passed in.", 10, result); } /* Test private method isFull on default list. */ @Test public void isFullTest1()throws Exception { Class classToCall = Class.forName("MediaList"); Method methodToExecute = classToCall.getDeclaredMethod("isFull"); methodToExecute.setAccessible(true); Boolean boolObj = (Boolean)methodToExecute.invoke(mediaList); boolean result = boolObj.booleanValue(); assertEquals("Test 17: The isFull method should return false on an array with NULL values.", false, result); } /** Test the getItemList method after expansion. **/ @Test public void getItemListAfterExpansionTest() { mediaList = new MediaList(2); addSixMixedItems(mediaList); MediaItem[] items = mediaList.getItemList(); assertEquals("Test 18: Test the getItemList method after expansion.", 6, items.length); } @Test // // @GradedTest(name = "Test 1: The instance variable initialLength has not been declared.", max_score = 4.0) public void getTitleOnEmptyListTest() { MediaItem[] titleItems = mediaList.getItemsByTitle("Goosebumps"); assertEquals("Test 19: getItemsByTitle on empty list should return array length 0.", 0, titleItems.length); } @Test // // @GradedTest(name = "Test 1: The instance variable initialLength has not been declared.", max_score = 4.0) public void getGenreOnEmptyListTest() { MediaItem[] genreItems = mediaList.getItemsByGenre("sci fi"); assertEquals("Test 20: getItemsByGenre on empty list should return array length 0.", 0, genreItems.length); } @Test // // @GradedTest(name = "Test 1: The instance variable initialLength has not been declared.", max_score = 4.0) public void getOneMatchTitleTest() { mediaList.addItem(new Song("Humble","Kendrick Lamar","hip hop", 2.57,"Damn","Top Dawg")); MediaItem[] genreItems = mediaList.getItemsByTitle("Humble"); assertEquals("Test 21: getItemsByTitle should return array length 1 with one match.", 1, genreItems.length); } @Test // // @GradedTest(name = "Test 1: The instance variable initialLength has not been declared.", max_score = 4.0) public void getOneMatchGenreTest() { mediaList.addItem(new Song("Humble","Kendrick Lamar","hip hop", 2.57,"Damn","Top Dawg")); MediaItem[] genreItems = mediaList.getItemsByGenre("hip hop"); assertEquals("Test 22: getItemsByGenre should return array length 1 with one match.", 1, genreItems.length); } // populate the array with 6 mixed types. private void addSixMixedItems(MediaList list){ list.addItem(new Movie("2001; A Space Odyssey","Kubrick","sci fi",142,"Keir Dullea","1968")); list.addItem(new Book("Snow Crash","Stephenson","sci fi",480,3.5)); list.addItem(new Book("Goosebumps","R.L.Stine", "fiction",128,3.5)); list.addItem(new Song("Humble","Kendrick Lamar","hip hop", 2.57,"Damn","Top Dawg")); list.addItem(new Song("Goosebumps","Travis Scott","trap",4.03,"Birds in the Trap Sing McKnight","Epic")); list.addItem(new Movie("Black Panther", "Coogler", "fantasy", 134, "Chadwick Boseman", "2018")); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
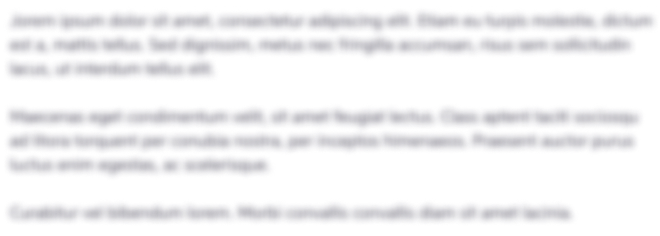
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started