Question
import java.util.Set; public class SetUtilities { /** * Returns a new set representing the union of s and t. * * Does not modify s
import java.util.Set;
public class SetUtilities {
/**
* Returns a new set representing the union of s and t.
*
* Does not modify s or t.
* @param s
* @param t
* @return a new set representing the union of s and t
*/
public static
}
/**
* Returns a new set representing the intersection of s and t.
*
* Does not modify s or t.
* @param s
* @param t
* @return a new set representing the intersection of s and t
*/
public static
}
/**
* Returns a new set representing the set difference s and t,
* that is, s \ t.
*
* Does not modify s or t.
* @param s
* @param t
* @return a new set representing the difference of s and t
*/
public static
}
/**
* Returns the Jaccard index of the two sets s and t.
*
* It is defined as 1 if both sets are empty.
*
* Otherwise, it is defined as the size of the intersection of the sets,
* divided by the size of the union of the sets.
*
* Does not modify s or t.
*
* @param s
* @param t
* @return the Jaccard index of s and t
*/
public static
}
} =======================================================================
import java.util.List;
import java.util.Set;
public class SimilarityUtilities {
/**
* Returns the set of non-empty lines contained in a text, trimmed of
* leading and trailing whitespace.
*
* @param text
* @return the trimmed set of lines
*/
public static Set
return null;
}
/**
* Returns a list of words in the text, in the order they appeared in the text,
* converted to lowercase.
*
* Words are defined as a contiguous sequence of letters and numbers.
*
* @param text
* @return a list of lowercase words
*/
public static List
return null;
}
/**
* Returns the line-based similarity of two texts.
*
* The line-based similarity is the Jaccard index between each text's line
* set.
*
* A text's line set is the set of trimmed lines in that text, as defined by
* trimmedLines.
*
* @param text1
* a text
* @param text2
* another text
* @return
*/
public static double lineSimilarity(String text1, String text2) {
return -1.0;
}
/**
* Returns the line-based similarity of two texts.
*
* The line-based similarity is the Jaccard index between each text's line
* set.
*
* A text's line set is the set of trimmed lines in that text, as defined by
* trimmedLines, less the set of trimmed lines from the templateText. Removes
* the template text from consideration after trimming lines, not before.
*
* @param text1
* a text
* @param text2
* another text
* @param templateText
* a template, representing things the two texts have in common
* @return
*/
public static double lineSimilarity(String text1, String text2, String templateText) {
return -1.0;
}
/**
* Returns a set of strings representing the shingling of the given length
* of a list of words.
*
* A shingling of length k of a list of words is the set of all k-shingles
* of that list.
*
* A k-shingle is the concatenation of k adjacent words.
*
* For example, a 3-shingle of the list: ["a" "very" "fine" "young" "man"
* "I" "know"] is the set: {"averyfine" "veryfineyoung" "fineyoungman"
* "youngmanI" "manIknow"}.
*
* @param words
* @param shingleLength
* @return
*/
public static Set
return null;
}
/**
* Returns the shingled word similarity of two texts.
*
* The shingled word similarity is the Jaccard index between each text's
* shingle set.
*
* A text's shingle set is the set of shingles (of the given length) for the
* entire text, as defined by shingle and asLowercaseWords,
* less the shingle set of the templateText. Removes the templateText
* from consideration after shingling, not before.
*
* @param text1
* @param text2
* @param templateText
* @param shingleLength
* @return
*/
public static double shingleSimilarity(String text1, String text2, String templateText, int shingleLength) {
return -1.0;
}
}
1. Translate written descriptions of behavior into code. 2. String.split 3. implement the shingleSimilarity method, which parallels the second lineSimilarity method
Thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
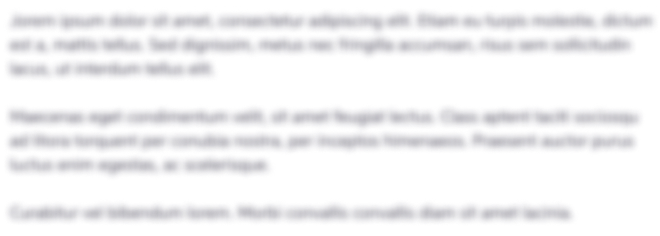
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started