Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.Stack; /** * Lab 4: Practice with Stack operations * * See comments for assignment instructions. * * @author Your Name Goes Here *
import java.util.Stack; /** * Lab 4: Practice with Stack operations * * See comments for assignment instructions. * * @author Your Name Goes Here * */ public class StackCopy { /** * Make an identical copy of a Stack. * post-condition: s is the same as it was before the method was called. * @param s The Stack to copy. * @return An identical copy of the Stack s. */ public static Stack copy(Stack s) { // (1) Complete this method. Although Java's Stack class provides methods // for access by an index, you are not allowed to use those methods in // this lab assignment. You are also not allowed to use the clone method // of Java's Stack class. You are allowed to use only the following // methods of Java's Stack class: empty, peek, pop, and push (and also // the constructor since you will need to construct a couple Stacks). // Stack Copy Algorithm: Assumes the Stack to copy is named s (as it is in the parameter of this method. // Let copy be an initially empty Stack. // Let temp be an initially empty Stack. // while s is not empty // Pop s and store the result in element. // Push element onto temp. // end while // while temp is not empty // Pop temp and store the result in element. // Push element onto copy. // Push element onto s. // end while // return copy return null; } /** * Make a reverse copy of a Stack. * post-condition: s is the same as it was before the method was called. * @param s The Stack to copy. * @return A reverse copy of the Stack s. */ public static Stack reverseCopy(Stack s) { // (2) Complete this method. Although Java's Stack class provides methods // for access by an index, you are not allowed to use those methods in // this lab assignment. You are also not allowed to use the clone method // of Java's Stack class. You are allowed to use only the following // methods of Java's Stack class: empty, peek, pop, and push (and also // the constructor since you will need to construct a couple Stacks). // Stack Reverse Copy Algorithm: Assumes the Stack to copy is named s (as it is in the parameter of this method. // Let reverse be an initially empty Stack. // Let temp be an initially empty Stack. // while s is not empty // Pop s and store the result in element. // Push element onto temp. // Push element onto reverse. // end while // while temp is not empty // Pop temp and store the result in element. // Push element onto s. // end while // return reverse return null; } public static void main(String[] args) { // (3) Complete this main method to test your methods. // Create a Stack of whatever type of element you want, such as Strings, // Integers, etc, and put at least 5 different elements in it. // Use the copy method to make a duplicate Stack System.out.println("Original Stack"); // Use a for-each style loop to print the original Stack // This type of loop will work with Java's Stack class since it implements // the Iterable interface, although you don't typically iterate over a Stack // like this. System.out.println("Copy Stack"); // Use a for-each style loop to print the copy // Use the reverseCopy method to make a copy of the original Stack in reverse order System.out.println("Original Stack"); // Use a for-each style loop to print the original Stack System.out.println("Reverse copy Stack"); // Use a for-each style loop to print the reverse copy } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
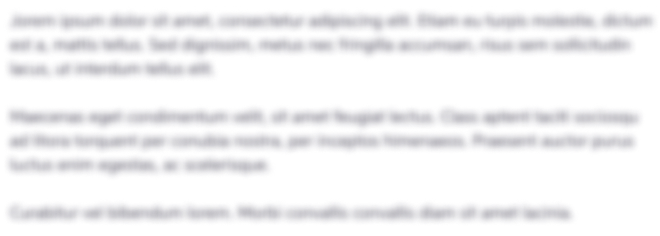
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started