Question
import javax.swing.JOptionPane; public class Pyramid { public static void main(String[] args) { // decalre variables String input; double radius, slant_height, height, volume, lateral_surface_area, base_area, Total_area;
import javax.swing.JOptionPane;
public class Pyramid {
public static void main(String[] args) {
// decalre variables
String input;
double radius, slant_height, height, volume, lateral_surface_area, base_area, Total_area;
input = JOptionPane.showInputDialog("Enter radius");
radius = Double.parseDouble(input);
input = JOptionPane.showInputDialog("Enterheight");
height = Double.parseDouble(input);
if (radius < 0 || height < 0)
JOptionPane.showMessageDialog(null, "Invalid inputs");
else {
//Calculate
volume = (1 / 3.0) * radius * radius * height * Math.PI;
slant_height = Math.sqrt(height * height + radius * radius);
lateral_surface_area = slant_height * radius * Math.PI;
base_area = Math.PI * radius * radius;
Total_area = lateral_surface_area + base_area;
//output
String resultMessage = "Volume : " + String.valueOf(volume) + " Height : " + height + "Slant Height"
+ slant_height + " Lateral Surface Area:" + String.valueOf(lateral_surface_area)
+ "Base Surface Area:" + String.valueOf(base_area) + " Total Surface Area : "
+ String.valueOf(Total_area);
JOptionPane.showMessageDialog(null, resultMessage);
}
}
}
Modify the program I have completed
Step 1: Write four method definitions as follows:
The first method takes the radius and height
as the parameters, then
calculates and returns the
slant height.
The second method takes the radius and height as the parameters, then calculates and returns the volume.
The third method takes the radius and slant height as the parameters, then calculates and returns the total surface area.The fourth method takes the radius, height, slant height, volume, and total surface area as the parameters, then prints the result.
Step 2: In the main method, the program does the following:
It prompts the user to enter the radius and height
.
If the user input is valid, it calls the four methods with appropriate arguments to calculate and
print the result. Otherwise, prints an error message.
Format the result to 2 decimal places.
Sample Run:
Enter radius:
12.34
Enter height:
23.45
********* Result ****************
Slant Height = 26.50
Volume = 3,739.40
Total Surface Area = 1,505.67
Step by Step Solution
There are 3 Steps involved in it
Step: 1
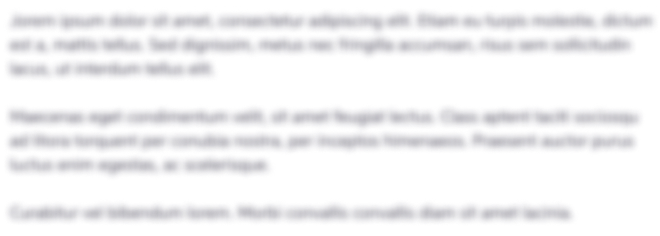
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started