Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import numpy as np def loss ( w , b , xTr , yTr , C ) : INPUT: w : d
import numpy as np
def lossw b xTr yTr C:
INPUT:
w : d dimensional weight vector
b : scalar bias
xTr : nxd dimensional matrix each row is an input vector
yTr : n dimensional vector each entry is a label
C : scalar constant that controls the tradeoff between lregularizer and hingeloss
OUTPUTS:
loss : the total loss obtained with w b on xTr and yTr scalar
Tests:
xTrtest, yTrtest generatedata
n d xTrtest.shape
# Check whether your loss returns a scalar
def losstest:
w nprandom.randd
b nprandom.rand
lossval lossw b xTrtest, yTrtest,
return npisscalarlossval
# Check whether your loss returns a nonnegative scalar
def losstest:
w nprandom.randd
b nprandom.rand
lossval lossw b xTrtest, yTrtest,
return lossval
# Check whether you implement lregularizer correctly
def losstest:
w nprandom.randd
b nprandom.rand
lossval lossw b xTrtest, yTrtest,
lossvalgrader lossgraderw b xTrtest, yTrtest,
return nplinalg.normlossval lossvalgradere
# Check whether you implemented the squared hinge loss and not the standard hinge loss
# Note, lossgraderwrong is the wrong implementation of the standard hingeloss,
# so the results should NOT match.
def losstest:
w nprandom.randnd
b nprandom.rand
lossval lossw b xTrtest, yTrtest,
badloss lossgraderwrongw b xTrtest, yTrtest,
return notnplinalg.normlossval badlosse
# Check whether you implement square hinge loss correctly
def losstest:
w nprandom.randnd
b nprandom.rand
lossval lossw b xTrtest, yTrtest,
lossvalgrader lossgraderw b xTrtest, yTrtest,
return nplinalg.normlossval lossvalgradere
# Check whether you implement loss correctly
def losstest:
w nprandom.randnd
b nprandom.rand
lossval lossw b xTrtest, yTrtest,
lossvalgrader lossgraderw b xTrtest, yTrtest,
return nplinalg.normlossval lossvalgradere
runtestlosstest'losstest
runtestlosstest'losstest
runtestlosstest'losstest
runtestlosstest'losstest
runtestlosstest'losstest
runtestlosstest'losstest
Step by Step Solution
There are 3 Steps involved in it
Step: 1
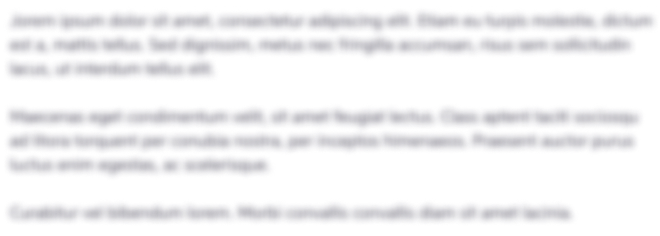
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started