Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import static java.lang.Math.abs; public class HashTableOpenAddressing extends Dictionary { private int capacity; // size of the table private int size; // number of elements in
import static java.lang.Math.abs; public class HashTableOpenAddressingextends Dictionary { private int capacity; // size of the table private int size; // number of elements in the table private int previousPrime; //store prev prime so that it is not calculated again and again in double hashing. private int mode; public static int LINEARPROBING = 1; public static int QUADRATICPROBING = 2; public static int DOUBLEHASHING = 3; private double loadFactor; private Entry [] table; public HashTableOpenAddressing() { this(DOUBLEHASHING, 11, 0.75); // default initial capacity of 10 } public HashTableOpenAddressing(int mode) { this( mode, 11, 0.75); } public HashTableOpenAddressing(int capacity, double loadFactor) { this(DOUBLEHASHING, capacity, loadFactor); } /* TODO: This constructor takes a mode, capacity, loadFactor, and sets those variables + relevant variables according to such. Additionally, it will set up the table according to the capacity. If the mode is DOUBLEHASHING, please calculate the previousPrime and set it. */ public HashTableOpenAddressing(int mode, int capacity, double loadFactor) { } private int previousPrime(int number) { while( true ) { if( isPrime( number ) ) { return number; } number--; } } // TODO: // second hash should be prevPrime - (key % prevPrime)...shouldn't be negative private int hash2(K key) { return 0; } // TODO: gets the next index given the index and the offset. Please take into account the mode. private int getNextIndex(K key, int offset) { return 0; } // TODO: // Put a key, value pair into the table. // If the key already exists/inactive, override it. Else, put it into the table. // Throw a RuntimeException if there is an infinite loops. public void put(K key, V value) { } // TODO: // Retrieves the value of a key in the table. // If there is an infinite loop, throw a RuntimeException. // Return null if not there. public V get(K key) { return null; } // TODO: Searches the table to see if the key exists or not. public boolean containsKey(K key) { return false; } // TODO: // Set the key as inactive if it exists in the table. Return true. // If there is no key, return false. // If there's an infinite loop, throw a RuntimeException. public boolean remove(K key) { return false; } public int size() { return size; } public boolean isEmpty() { return size == 0; } // TODO: // Calculate the absolute hash of the key. Do not overthink this. private int hash(K key) { return 0; } private boolean isPrime(int number) { for( int i = 2; i <= number / 2; i++ ) { if( number % i == 0 ) { return false; } } return true; } private int nextPrime(int number) { while( true ) { if( isPrime( number ) ) { return number; } number++; } } // TODO: // Set the capacity to the nextPrime of the capacity doubled. // Calculate the previousPrime and set up the new table with the old tables' // contents now hashed to the new. private void resize() { } @Override public String toString() { StringBuilder sb = new StringBuilder(); sb.append("{"); int index = 0; for (Entry entry : table) { sb.append(index + ": "); index++; if (entry != null) { sb.append(entry.getKey() + "=" + entry.getValue() + ","); } sb.append(";"); } if (sb.length() > 1) { sb.setLength(sb.length() - 2); } sb.append("}"); return sb.toString(); } private class Entry { private K key; private V value; private boolean isActive; public Entry(K key, V value) { this.key = key; this.value = value; isActive = true; } public K getKey() { return key; } public V getValue() { return value; } public void setValue(V value) { this.value = value; } public boolean getActive() { return isActive; } public void setActive(boolean active) { isActive = active; } } public static void main(String []args ) { HashTableOpenAddressing hashTable = new HashTableOpenAddressing<>(QUADRATICPROBING, 10, 1); hashTable.put(2,2); System.out.println(hashTable); for (int i = 0; i < 280; i += 10) { hashTable.put(i, i); hashTable.remove(0); System.out.println(hashTable.get(i)); } } }
import java.util.ArrayList; import java.util.LinkedList; import java.util.List; public class HashTableWithChainingextends Dictionary { private int capacity; // size of the table private int size; // number of elements in the table private double loadFactor; private List >> table; // hash table // Entry class to hold key-value pairs private class Entry { private K key; private V value; public Entry(K key, V value) { this.key = key; this.value = value; } public K getKey() { return key; } public V getValue() { return value; } public void setValue(V value) { this.value = value; } } public HashTableWithChaining() { this(11, 0.75); // default initial capacity of 10 } public HashTableWithChaining(int capacity) { this( capacity, 0.75); } /* TODO: This constructor takes a capacity and loadFactor, and sets those variables + relevant variables according to such. Additionally, it will set up the table according to the capacity. */ public HashTableWithChaining(int capacity, double loadFactor) { } // TODO: // Put a key, value pair into the table. // If the key already exists, update it with the new value. // If there is no key at that index, add it into the table. // Resize when the size is > the loadFactor * capacity. // Remember that multiple keys can exist at the same index. public void put(K key, V value) { } private boolean isPrime(int number) { for( int i = 2; i <= number / 2; i++ ) { if( number % i == 0 ) { return false; } } return true; } private int nextPrime(int number) { while( true ) { if( isPrime( number ) ) { return number; } number++; } } // TODO: // Set the capacity to the nextPrime of the capacity doubled. // Calculate the previousPrime and set up the new table with the old tables' // contents now hashed to the new. private void resize() { //find the next prime number twice that of capacity... } // TODO: // Retrieves the value of a key in the table. // Return null if not there. public V get(K key) { return null; } // TODO: Searches the table to see if the key exists or not. public boolean containsKey(K key) { return false; } // TODO: // Remove the entry under that key. Return true. // If there is no key, return false. public boolean remove(K key) { return false; } public void clear() { for (LinkedList > list : table) { list.clear(); } size = 0; } public boolean isEmpty() { return size == 0; } public int size() { return size; } // TODO: Calculate the absolute hash of the key. Do not overthink this. private int hash(K key) { return 0; } @Override public String toString() { StringBuilder sb = new StringBuilder(); sb.append("{"); int index = 0; for (LinkedList > list : table) { if(list.size() > 0 ) { sb.append(index + ": "); for (Entry entry : list) { sb.append(entry.getKey() + "=" + entry.getValue() + ","); } index++; sb.append(";"); } } if (sb.length() > 1) { sb.setLength(sb.length() - 2); } sb.append("}"); return sb.toString(); } public static void main(String []args ) { HashTableWithChaining hashTable = new HashTableWithChaining<>(); hashTable.put("Hi", 2); hashTable.put("Ih", 2); hashTable.put("Hit", 2); hashTable.put("Him", 20); hashTable.put("His", 1); hashTable.put("Hiasdasd", 2); hashTable.put("Hiasdasds", 1); hashTable.put("Hiasdasadsd", 2); hashTable.put("H12is", 1); hashTable.put("H123iasdasd", 2); hashTable.put("Hita123s1d3asads", 2); System.out.println(hashTable); } }
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Scanner; public class SearchEngine { private int mode; private DictionarynodeTable; // build everything bahahah // TODO: mode 5 = Openaddressing mode 6 = Chaining; build public SearchEngine(int mode) throws IOException { this.mode = mode; } public Dictionary getNodeTree(){ return this.nodeTable; } // TODO: assumes that the file exists already public void buildList() throws IOException { System.out.println("reading"); BufferedReader reader = new BufferedReader(new FileReader("dataset.txt")); String url; while((url = reader.readLine()) != null){ Document doc = Jsoup.connect(url).get(); String text = doc.body().text().toLowerCase(); if(url.equals("https://en.wikipedia.org/wiki/Kamala_Harris")) System.out.println(text); // System.out.println(text); String[] words = text.split("\\s+"); // splits by whitespace int count = 0; for (String word : words) { // TODO: } } reader.close(); System.out.println("Finished reading through all URLs"); } // TODO: return the results from one term public ArrayList search(String term) { System.out.println("Searching for " + term + " using data structure mode " + mode + "..."); return new ArrayList<>(); } public static void main(String[] args) throws IOException{ Scanner input = new Scanner(System.in); System.out.println("Enter mode as in what data structure to use:"); System.out.println(" 5. HashTableOpenAddressing "); System.out.println(" 6. HashTableWithChaining"); int mode = input.nextInt(); System.out.println("Building Search Engine..."); SearchEngine engine = new SearchEngine(mode); String answer = "y"; while (answer.equals("y")) { input.nextLine(); // consume the remaining newline character System.out.print("Search (enter a term to query): "); String term = input.nextLine(); engine.search(term); System.out.print("Would you like to search another term (y/n)? "); answer = input.nextLine(); } input.close(); } }
import java.util.ArrayList; public class Node implements Comparable{ private String keyword; private ArrayList references; public Node(String keyword){ this.keyword = keyword; this.references = new ArrayList (); } public String getKeyword(){ return this.keyword; } public ArrayList getReferences(){ return this.references; } // // public int compareTo(Object o){ // if(o instanceof Node){ // Node other = (Node) o; // return this.keyword.compareTo(other.keyword); // } // return -1; // } public void insertReference(String website){ this.references.add(website); } public boolean equals (Object o) { if (o instanceof Node) { Node other = (Node) o; return this.keyword.equals(other.keyword); } else return false; } public String toString(){ return this.keyword; } public int compareTo(Node o) { return this.keyword.compareTo(o.keyword); } }
abstract class Dictionary{ public abstract boolean remove(K key); public abstract boolean containsKey(K key); public abstract V get(K key); public abstract void put(K key, V value); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
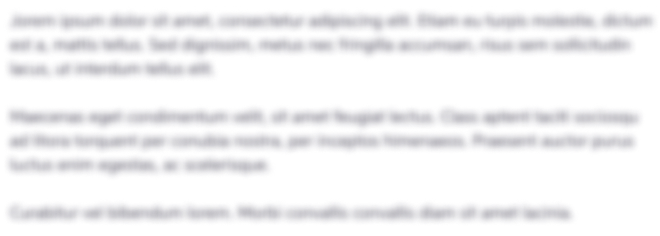
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started