Question
In 32-bit x86 assembly language masm you are going to develop a famous word search puzzle game using 32-bit x86 assembly language. A word search
In 32-bit x86 assembly language masm you are going to develop a famous word search puzzle game using 32-bit x86 assembly language. A word search puzzle consists of the letters of words placed in a grid, which usually has a square shape. Given a list of the hidden words, the objective of this puzzle is to find and mark all the words hidden inside the box. The words may be placed horizontally, vertically, or diagonally. The puzzle that you are developing must meet the following requirements: 1) The puzzle grid is of size 15 x 15 2) Words to be used for the puzzle are read from the file having 500 words in which each line corresponds to a single word and each words length is between 6 to 10 letters 3) Eight words are hidden in the puzzle. 4) Words can be placed in any of the following six directions where each direction is assigned a unique number. 5) Words are placed at random position and at random direction in the puzzle grid 6) The puzzle game must be timed and the player must solve the puzzle in 10 minutes 7) The player must enter the guessed word in the following format: w r c d where w is the word found, r is the row no in the gird from where the word starts, c is the column no in the gird from where the word starts, and d is the direction no. 8) The player earns a score of +10 on correct answer and -10 on wrong answer 9) Found words must be highlighted within the puzzle grid. 10) The program ends if either the player founds all the word in the puzzle within 10 minutes, or player inputs the answer after the 10 minutes time since the start of puzzle. 11) You must make sure that all the exceptions have been handled (e.g. Input provided in wrong format is not processed. Score must not be added if player re-enters the already found word) you may use the following procedure for random number generation. However, you are also welcome to write your own random number generator.
; Generate a random number in the range [0, n-1] ; Expect one parameter that is 'n' in EAX ; returns random number in EAX ; seed is a dword type variable global variable initialized with positive number ; (Its not a good practice though to use global variables inside the procedure) generaterandom proc uses ebx edx mov ebx, eax ; maximum value mov eax, 343FDh imul seed add eax, 269EC3h mov seed, eax ; save the seed for the next call ror eax,8 ; rotate out the lowest digit mov edx,0 div ebx ; divide by max value mov eax, edx ; return the remainder ret generaterandom endp Given below are prototypes of Windows library function that would be helpful for this project: GetTickCount PROTO ; get elapsed milliseconds ; since computer turned on ExitProcess PROTO, ; exit program dwExitCode:DWORD ; return code GetStdHandle PROTO, ; get standard handle nStdHandle:DWORD ; type of console handle CloseHandle PROTO, ; close file handle handle:DWORD GetTickCount PROTO ; get elapsed milliseconds since computer ; turned on 3 ReadConsoleA PROTO, handle:DWORD, ; input handle lpBuffer:PTR BYTE, ; pointer to buffer nNumberOfCharsToRead:DWORD, ; number of chars to read lpNumberOfCharsRead:PTR DWORD, ; number of chars read lpReserved:PTR DWORD ; 0 (not used - reserved) WriteConsoleA PROTO, ; write a buffer to the console handle:DWORD, ; output handle lpBuffer:PTR BYTE, ; pointer to buffer nNumberOfCharsToWrite:DWORD, ; size of buffer lpNumberOfCharsWritten:PTR DWORD, ; number of chars written lpReserved:PTR DWORD ; 0 (not used) CreateFileA PROTO, ; create new file pFilename:PTR BYTE, ; ptr to filename accessMode:DWORD, ; access mode shareMode:DWORD, ; share mode lpSecurity:DWORD, ; can be NULL howToCreate:DWORD, ; how to create the file attributes:DWORD, ; file attributes htemplate:DWORD ; handle to template file ReadFile PROTO, ; read buffer from input file fileHandle:DWORD, ; handle to file pBuffer:PTR BYTE, ; ptr to buffer nBufsize:DWORD, ; number bytes to read pBytesRead:PTR DWORD, ; bytes actually read pOverlapped:PTR DWORD ; ptr to asynchronous info SetConsoleTextAttribute PROTO, nStdHandle:DWORD, ; console output handle nColor:DWORD ; color attribute SetConsoleCursorPosition PROTO, handle:DWORD, pos:DWORD GetTimeFormatA PROTO :DWORD,:DWORD,:DWORD,:DWORD,:DWORD,:DWORD
Step by Step Solution
There are 3 Steps involved in it
Step: 1
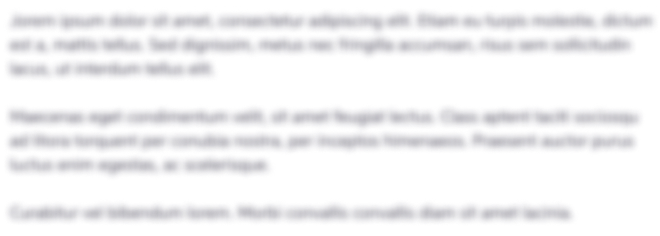
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started