Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In a hacking competition, our team breaks into the adversary s computer and finds the following files. $ ls encrypt.c encrypted - message 2 encrypted
In a hacking competition, our team breaks into the adversarys computer and finds the following files.
$ ls
encrypt.c encryptedmessage encryptedmessage encryptedmessage
encryptedmessage encryptedmessage encryptedmessage encryptedmessage
We cannot see the content of the seven encrypted files but we are able to see the content of file encrypt.c
#include
#include
#include
#include
#include
#include
#define MAX
encrypt the message and write the result to file fd
void encryptchar message unsigned char key, unsigned char shift, int fd
int len strlenmessage;
printflen d
len;
int msglen;
forint i ; i ;
note how we open the file
fd openargv OWRONLY OTRUNC OCREAT, ;
iffd
printfCannot open the file
;
return ;
int key atoiargv;
int shift atoiargv;
assertkey && key ;
assertshift && shift ;
encryptmessage key, shift, fd;
remember to close the file
closefd;
return ;
We believe these seven files are encrypted using the above code. Now we need to break the code: decrypt
the seven files to see their original content.
From the code we can see that each character in the original message is converted to an integer by applying
bit shifting and then an exclusive or operation with a key. The number of shifts and the key are
not known, since they are passed as command line arguments. We will use a bruteforce method to try all
combinations of shift and key values and see which combination reveals the original message.
To break the code, we use a dictionary to check the decrypted text. For example, we can count the number
of words in the decrypted text, according to the dictionary. We can even be more creative and come up with
better measures.
The content of the first few lines of the dictionary file dict.txt are the following.
aardvark
aardwolf
aaron
aback
abacus
abaft
abalone
abandon
abandoned
abandonment
abandons
abase
Note the dictionary file is sorted alphabetically. We can take advantage of this fact in our code.
Below is the main function for our program.
Do not change the main function
int mainint argc, char argv
ifargc
printfs encryptedmessage
argv;
return ;
readfiletoarraydicttxt;
int encryptedMAX;
int len readencryptedargv encrypted;
char messageMAX;
strcpymessage;
searchencrypted len, message;
printfs
message;
return ;
The function search is already implemented as following.
search using all the key shift combinations
to find the original message
result is saved in message
void searchconst int encrypted int len, char message
char decryptedMAX;
int maxscore ;
strcpymessage;
forunsigned char k ; k ; k
forunsigned char shift ; shift ; shift
decryptionk shift, encrypted, len, decrypted;
int score messagescoredecrypted;
ifscore maxscore
maxscore score;
strcpymessage decrypted;
We need to implement the following functions.
TODO
Test wether a string word is in the dictionary
Return if word is in the dictionary
Return otherwise
Be efficient in implementing this function
Efficiency is needed to pass test cases in limited time
int indictchar word
TODO
Use key and shift to decrypt the encrypted message
len is the length of the encrypted message
Note the encrypted message is stored as an array of integers not chars
The result is in decrypted
void decryptionunsigned char key, unsigned char shift, const int encrypted int len, char dec
rypted
TODO
calculate a score for a message msg
the score is used to determine whether msg is the original message
int messagescoreconst char msg
TODO
read the encrypted message from the file to encrypted
return number of bytes read
int readencryptedchar filename int encrypted
starter code
#include
#include
#include
#include
#include
#include
#include
#define MAX
#define MAXWORDCOUNT we have less than words
#define MAXWORDLENGTH each word is less than letters
Using these two global variables can be justified :
char wordsMAXWORDCOUNTMAXWORDLENGTH; d array to hold all the words
int wordcount ; number of words, initilized to
Note the words in the dictionary file are sorted
This fact could be useful
void readfiletoarraychar filename
FILE fp;
open the file for reading
fp fopenfilenamer;
iffpNULL
printfCannot open file s
filename;
exit;
make sure when each word is saved in the array words,
it does not ends with a
see how this is done using fscanf
whilefeoffp
fscanffps
words
Step by Step Solution
There are 3 Steps involved in it
Step: 1
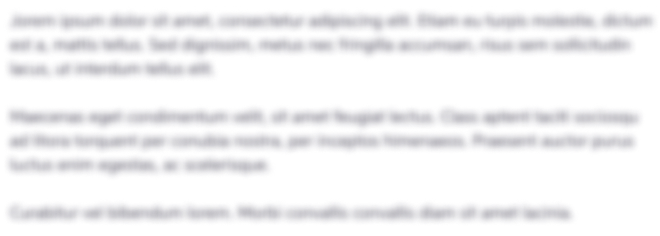
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started