Question
In a loop, the user will be presented with a random number between 1 and 100. They can choose to Keep the number, Discard the
In a loop, the user will be presented with a random number between 1 and 100. They can choose to Keep the number, Discard the number, or Quit. (when quitting the last number is not considered)
The user has two lists: a discard list and a keep list. Each list is kept in order that they were added.
When the user quits the loop, each list is displayed (if any items) as well as the total and average for each list (see sample runs)
Technical Items / Assumptions
You MUST use arrays (not ArrayList) for this program
Allocate 20 for the size of each array.
You can assume that the user will not overfill the array
Honors Option
Do not let the user overfill either list
Generate no more than 25 numbers total (meaning loop will end either when user keeps/discards the 25th number, or once user selects quit)
Not only display the total and average for each list, also display what the total and average for all numbers generated
Grading Rubric
Must have two arrays of size 20 for discarded and kept #s (10 points)
Loop that exits on Q for quit (10 points)
Top of loop generate and display a random number in the range 1-100 (5 points)
allow user to discard [ add to discarded array ] (10 points)
allow user to keep [ add to kept array ] (10 points)
On quit
display discarded list (if any) (5 points)
Display discarded total and average (10 points)
display Kept list (if any) (5 points)
Display kept total and average (10 points)
Your name in code at top (2 points)
Class name following guidelines (begin capital) (5 points)
Variable names following guidelines (begin small letter, descriptive) (10 points)
Proper indenting [ Body of class indented / Body of method indented / Conditionally executed code indented ] (8 points)
Sample Run (regular):
New number to consider is 20
Enter Option: (K, D, Q): d
New number to consider is 96
Enter Option: (K, D, Q): k
New number to consider is 58
Enter Option: (K, D, Q): k
New number to consider is 41
Enter Option: (K, D, Q): d
New number to consider is 62
Enter Option: (K, D, Q): k
New number to consider is 73
Enter Option: (K, D, Q): k
New number to consider is 17
Enter Option: (K, D, Q): d
New number to consider is 17
Enter Option: (K, D, Q): d
New number to consider is 8
Enter Option: (K, D, Q): d
New number to consider is 16
Enter Option: (K, D, Q): d
New number to consider is 83
Enter Option: (K, D, Q): k
New number to consider is 6
Enter Option: (K, D, Q): q
You discarded:
20 41 17 17 8 16
Total 119 - Average 19.8
You kept:
96 58 62 73 83
Total 372 - Average 74.4
Press any key to continue . . .
Another Sample Run(regular):
New number to consider is 27
Enter Option: (K, D, Q): d
New number to consider is 28
Enter Option: (K, D, Q): d
New number to consider is 60
Enter Option: (K, D, Q): d
New number to consider is 93
Enter Option: (K, D, Q): q
You discarded:
27 28 60
Total 115 - Average 38.3
You kept:
None
Press any key to continue . . .
Another Sample Run(regular):
New number to consider is 88
Enter Option: (K, D, Q): k
New number to consider is 72
Enter Option: (K, D, Q): k
New number to consider is 100
Enter Option: (K, D, Q): k
New number to consider is 60
Enter Option: (K, D, Q): q
You discarded:
None
You kept:
88 72 100
Total 260 - Average 86.7
Press any key to continue . . .
Another Sample Run (honors option):
New number to consider is 97
Enter Option: (K, D, Q): k
New number to consider is 67
Enter Option: (K, D, Q): d
New number to consider is 63
Enter Option: (K, D, Q): d
New number to consider is 56
Enter Option: (K, D, Q): d
New number to consider is 69
Enter Option: (K, D, Q): d
New number to consider is 1
Enter Option: (K, D, Q): d
New number to consider is 41
Enter Option: (K, D, Q): d
New number to consider is 28
Enter Option: (K, D, Q): d
New number to consider is 25
Enter Option: (K, D, Q): d
New number to consider is 76
Enter Option: (K, D, Q): k
New number to consider is 89
Enter Option: (K, D, Q): k
New number to consider is 74
Enter Option: (K, D, Q): q
You discarded:
67 63 56 69 1 41 28 25
Total 350 - Average 43.8
You kept:
97 76 89
Total 262 - Average 87.3
All Numbers:
Total 612 - Average 51.0
Press any key to continue . . .
Another Sample Run (honors option maximum # of numbers generated reached ):
New number to consider is 27
Enter Option: (K, D, Q): d
New number to consider is 81
Enter Option: (K, D, Q): k
New number to consider is 94
Enter Option: (K, D, Q): k
New number to consider is 78
Enter Option: (K, D, Q): k
New number to consider is 17
Enter Option: (K, D, Q): d
New number to consider is 43
Enter Option: (K, D, Q): d
New number to consider is 66
Enter Option: (K, D, Q): d
New number to consider is 20
Enter Option: (K, D, Q): d
New number to consider is 8
Enter Option: (K, D, Q): d
New number to consider is 94
Enter Option: (K, D, Q): k
New number to consider is 10
Enter Option: (K, D, Q): d
New number to consider is 100
Enter Option: (K, D, Q): k
New number to consider is 11
Enter Option: (K, D, Q): d
New number to consider is 63
Enter Option: (K, D, Q): d
New number to consider is 34
Enter Option: (K, D, Q): d
New number to consider is 78
Enter Option: (K, D, Q): k
New number to consider is 55
Enter Option: (K, D, Q): d
New number to consider is 91
Enter Option: (K, D, Q): k
New number to consider is 24
Enter Option: (K, D, Q): d
New number to consider is 88
Enter Option: (K, D, Q): k
New number to consider is 58
Enter Option: (K, D, Q): d
New number to consider is 42
Enter Option: (K, D, Q): d
New number to consider is 98
Enter Option: (K, D, Q): k
New number to consider is 95
Enter Option: (K, D, Q): k
This is the last Generated Number!
New number to consider is 15
Enter Option: (K, D, Q): d
Can't Generate any more numbers!
You discarded:
27 17 43 66 20 8 10 11 63 34 55 24 58 42 15
Total 493 - Average 32.9
You kept:
81 94 78 94 100 78 91 88 98 95
Total 897 - Average 89.7
All Numbers:
Total 1,390 - Average 55.6
Press any key to continue . . .
Step by Step Solution
There are 3 Steps involved in it
Step: 1
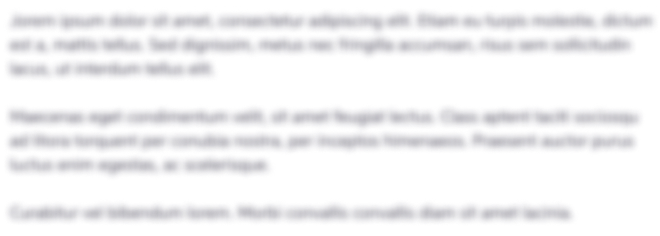
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started