Question
In a previous project, I created an address book that utilized a Vector Class. This week, I must modify the Vector address book program by
In a previous project, I created an address book that utilized a Vector Class.
This week, I must modify the Vector address book program by creating:
1. Person Class
2. Employer Class
3. Personal Info Class
These classes must utilize a HAS-A Relationship
Then, I must use the Employer and Personal Info classes as member variables of the Person class.
Modify the Vector class to hold People objects instead of Record objects.
Modify the menu interface and input algorithms to accept data for the Employer and Personal Info classes via functions in the Person class.
Modify the Output to print all the information in each Person object, associated Employer object, and associated Personal Info object.
Make sure to separate the implementation of classes from the header files.
Have a .cpp file and a .h file for each class I create.
Here is the code from the Vector Address Book that must be modified. It has a main.cpp, Record.h, Record.cpp, VectorClass.h, and VecotrClass.cpp:
/*main.cpp*/
/***** main.cpp *****/
#include
#include "Record.h"
#include "VectorClass.h"
using namespace std;
int main() {
VectorClass
int op, age, telephone;
string firstName, lastName;
while (1) {
cout << "1. Input information into a record." << endl;
cout << "2. Display all information in all records." << endl;
cout << "3. Exit the program" << endl;
cout << "Enter option: ";
cin >> op;
switch (op) {
case 1:
try {
cout << "Enter first name: ";
cin >> firstName;
cout << "Enter last name: ";
cin >> lastName;
cout << "Enter age: ";
cin >> age;
cout << "Enter telephone number: ";
cin >> telephone;
Record newRecord(recVector.size() + 1, firstName, lastName, age, telephone);
recVector.push_back(newRecord);
} catch (const exception& e) {
cerr << "Error: " << e.what() << endl;
}
break;
case 2:
cout << "Record Number FirstName LastName Age Telephone-Number" << endl;
cout << "-----------------------------------------------------" << endl;
for (int i = 0; i < recVector.size(); i++) {
recVector[i].print();
}
break;
case 3:
cout << "Exit the program." << endl;
return 0;
}
cout << endl;
}
}
/***** Record.h *****/
#ifndef RECORD_H
#define RECORD_H
#include
//Record class
class Record
{
private:
//private data member
int recordNumber;
string firstName;
string lastName;
int age;
int telephone;
public:
//default constructor
Record();
//parameterized constructor
Record(int, string, string, int, int);
//getter functions
int getRecordNumber();
string getFirstName();
string getLastName();
int getAge();
int getTelephone();
//setter functions
void setRecordNumber(int);
void setFirstName(string);
void setLastName(string);
void setAge(int);
void setTelephone(int);
void print();
};
#endif // RECORD_H
/***Record.cpp***/
#include
#include
#include"Record.h"
using namespace std;
//default constructor
Record::Record()
{
recordNumber = 0;
firstName = "";
lastName = "";
age = 0;
telephone = 0;
}
//parameterized constructor
Record::Record(int rno, string fn, string ln, int ag, int tno)
{
recordNumber = rno;
firstName = fn;
lastName = ln;
age = ag;
telephone = tno;
}
//getter functions
int Record::getRecordNumber() //returns the recordNumber
{
return recordNumber;
}
string Record::getFirstName() //returns the firstname
{
return firstName;
}
string Record::getLastName() //returns the lastname
{
return lastName;
}
int Record::getAge() //returns the age
{
return age;
}
int Record::getTelephone() //returns the telephone
{
return telephone;
}
//setter functions
void Record::setRecordNumber(int rno) //set the recordNumber
{
recordNumber = rno;
}
void Record::setFirstName(string fn) //set the firstname
{
firstName = fn;
}
void Record::setLastName(string ln) //set the lastname
{
lastName = ln;
}
void Record::setAge(int ag) //set the age
{
age = ag;
}
void Record::setTelephone(int tno) // //set the telephone
{
telephone = tno;
}
void Record::print() //print a record
{
cout<
/***VectorClass.h***/
#ifndef VECTOR_CLASS_H
#define VECTOR_CLASS_H
#include
#include
#include
template
class VectorClass {
private:
std::vector
public:
void push_back(const T& value);
T& operator[](int index);
int size() const;
};
#include "VectorClass.cpp"
#endif // VECTOR_CLASS_H
/***VectorClass.cpp***/
template
void VectorClass
vec.push_back(value);
}
template
T& VectorClass
if (index < 0 || index >= vec.size()) {
throw std::out_of_range("Index out of range");
}
return vec[index];
}
template
int VectorClass
return vec.size();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
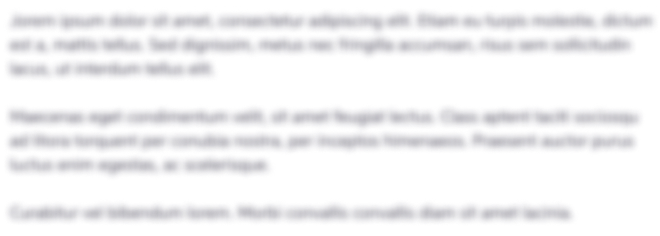
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started