Question
In android studio using kotlin please, For this assignment you are going to create a card game called Monty. Initially show a stack of 3
In android studio using kotlin please,
For this assignment you are going to create a card game called Monty. Initially show a stack of 3 cards face down and centered on the screen. Once the play button is tapped, animate the left and right cards to their respective positions. Once the player taps on a card, perform a flip animation for the reveal and show a superimposed ImageView indicating win or loss. The player wins if they pick the ace. You have to:
- Make this a landscape orientation-only app. Where the play button is enabled initially and disabled once tapped. The reset button starts its life in a disabled state and is enabled once the play button is tapped. Back and forth.
- Use ValueAnimators to animate cards to their left and right positions and back on reset. An ImageView's intrinsic width may come in handy: width = centerCardImageView.drawable.intrinsicWidth.toFloat() and use ObjectAnimators and AnimatorSet for a 2 stage approach to animate a card flip for the reveal.
- Use a ValueAnimator to animate the alpha value of an ImageView to announce winner or loser on reveal. On reset this ImageView is hidden.
- Add a fragment CardsFragment to your project. Then move your existing code and UI to the new fragment. In CardsFragment override onViewCreated and place your initialization code there. You can use findViewById in a fragment but have to prefix it with view: playBtn = view.findViewById(R.id.play_button) When done your app should compile, but cards, buttons, etc. will not be visible.
- Update activity_main as shown. The large rectangle is a FrameLayout that will host your fragment and needs an id. Use SupportFragmentManager in your activity to replace the contents of the FrameLayout with an instance of your CardsFragment. When done cards and buttons should show up again and the game work as before.
- Create an Interface called GameInterface with a single function onGameResults that returns the amount a player wins or loses. The amount is always 50, or -50 if the player loses. In CardFragment instantiate this callback in onAttach. You can now broadcast after each round whether the player won or lost 50 bucks: callback.onGameResults(amount) Your MainActivity has to implement GameInterface which in turn means that it has to implement onGameResults. Each time CardFragment reports a new result this function is now called in MainActivity and it's time to update the ui. When done you should be able to update the amount TextView with test values when onGameResults is called.
- To account for balance, wins and losses create a data class called Account. Instantiate and use it in MainActivity's onGameResults method to update your UI. When done your app should update player balance and number of wins/losses after each round.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
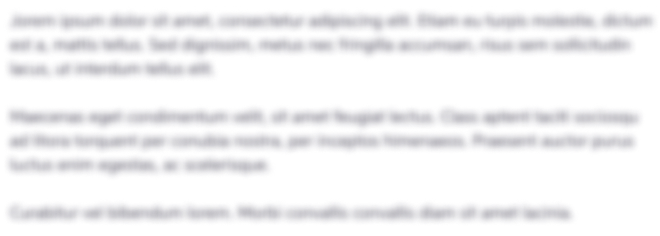
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started