Question
In C#, and please add comments. This is what it has to do. This program must prompt the user to enter their full name, gender
In C#, and please add comments. This is what it has to do.
This program must prompt the user to enter their full name, gender (as M or F), age (as an integer), and salary (as a double, with no dollar signs or commas). It needs to store the information into variables of the appropriate types, converting as necessary. Once all the data is gathered, you will open a file for writing and saving all the data to a file, one piece of data per line. Once the writing is complete, close the file for writing, open it for reading, and read in all the information again, storing it in a second set of variables to test that the data was properly written.
1.)Display the first welcome/thanks messages. 2.)Prompt for each piece of information, pausing for user input after each and storing the value in the appropriate variables (a string for name, char for gender, int for age, and double for salary). 3.)Tell the user that you are going to save their data. 4.)Open a file for writing. 5.)Write the data (all four pieces) to the file. Use WriteLine, not Write, as reading will be more difficult with the latter. 6.)Close the file. 7.)Tell the user that saving is complete and that you are going to do a test read. 8.)Open the file for reading. 9.)Read in the file, storing it in new variables of the appropriate types. 10.)Close the file. 11.)Output the values of the new variables to confirm that the information was correct. 12.)End the program.
This is what I have so far, it creates the file and stores the info, but doesn't let me add more than 1 entry, or read it, also when I re-run the program, it overrides the current data instead of adding it.
using System; using System.Collections; using System.Collections.Generic; using System.IO;
namespace MPeralez_Lab3 { class Employee { private string Name { get; set; } private char Gender { get; set; } private int Age { get; set; } private double Salary { get; set; }
public Employee(string name, char gender, int age, double salary) { this.Name = name; this.Gender = gender; this.Age = age; this.Salary = salary; }
public Employee() { this.Name = null; this.Gender = 'M'; this.Age = 0; this.Salary = 0.0; }
public override string ToString() { return this.Name + " " + this.Gender + " " + this.Age + " " + this.Salary;
}
}
class Program { static void Main(string[] args) { List
// Accepting input from the user do {
Console.WriteLine("Enter name: "); string name = Console.ReadLine(); Console.WriteLine("Enter gender: "); char gender = Console.ReadLine()[0]; Console.WriteLine("Enter age: "); int age = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("Enter salary: "); double sal = Double.Parse(Console.ReadLine()); Employee emp = new Employee(name, gender, age, sal); el.Add(emp); Console.WriteLine("Press 1 to add more records: "); Convert.ToInt32(Console.ReadLine());
} while (ch == 1);
// Write the records to the file using (System.IO.TextWriter writer = File.CreateText(@"D:\Program Stuff\Form.txt")) { foreach (Employee emp in el) { writer.WriteLine(emp.ToString()); } } Console.WriteLine(" Data written to the file. ");
// Read records from file and display them on the screen Console.WriteLine(" Displaying the data after reading from the file: "); TextReader readFile = new StreamReader(@"D:\Program Stuff\Form.txt"); while (true) {
string name = readFile.ReadLine(); if (name == null) { break; } char gender = readFile.ReadLine()[0]; int age = Convert.ToInt32(readFile.ReadLine()); double sal = Double.Parse(readFile.ReadLine()); Employee emp = new Employee(name, gender, age, sal); Console.WriteLine(" Name: " + name); Console.WriteLine("Gender: " + gender); Console.WriteLine("Age: " + age); Console.WriteLine("Salary: " + sal); }
//Close the file readFile.Close(); readFile = null;
Console.WriteLine(" Thank you !!!!"); } } }
Thank You!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
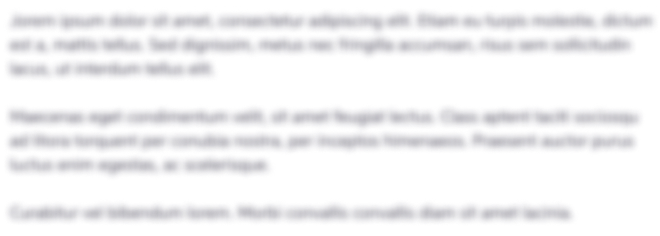
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started