Question
IN C#: Below I have the code that I did for this program and the instructions. I am not sure if my code is how
IN C#:
Below I have the code that I did for this program and the instructions. I am not sure if my code is how it should be for this program. I know that we were given 4 or 5 .txt files, I am not sure if I have to incorporate them into my program or what. Also, the sudoku puzzle don't let me keep doing different games. Can you please help me figure out how my code is suppose to actually look. It says something at the bottom of the instructions that for a Grade of 'B' I need to report all invalid Rows and Columns and for a Grade of 'A' I also need to report All Invalid Block. My code is not doing that. Please Help me.
INSTRUCTIONS:
A few years ago, a style of puzzle became pretty popular. The name most people know it by is Sudoku. If youve played it, you know the rules. Ill give them here in a nutshell. The user starts with a 9x9 grid. That grid is split into nine 3x3 blocks. There are a handful of cells filled in. The goal of the game is to fill in all the cells such that the following three rules are true:
each column uses each digit (1-9) exactly once
each row uses each digit exactly once
each of the 3x3 blocks uses each digit exactly once
Here are examples of a starting grid and a solution (lifted from Wikipedia).
"Sudoku-by-L2G-20050714 solution" by en:User:Cburnett
Your mission, should you choose to accept it, is to determine whether a given Sudoku answer is correct. This means that you will need to validate the three rules above for the given puzzle answer.
The puzzle will be in a .txt file. It will have nine rows of nine digits. The digits will be separated by commas. You will need to place the digits into a 9x9 two dimensional array. Then you will need to check each of the rules on the array. The numbers used will be 1-9. You do not need to worry about numbers outside the range.
At the end, you need to output VALID or INVALID. If the answer is invalid, you should write where you found it to be invalid. You must continue on to find and report all invalid locations, e.g.
ROW 0
COL 4
BLK 1
The rows are numbered left to right, the columns top to bottom, the blocks left-to-right, top to bottom, all starting with zero,
To be clear the blocks are numbered:
0 | 1 | 2 |
3 | 4 | 5 |
6 | 7 | 8 |
There are a number of txt files attached to the assignment, one good solution and a number of bad ones. There are notes in the file that give the expected errors. You may find these useful for testing your program. You are encouraged to create other good (and bad) solutions for further testing. Also, feel free to use any data structures in addition to a two-dimensional array that will help you in your validation.
Use good programming practices as you solve this problem. As always, you will be graded on efficiency, maintainability, etc. as well as correctness. Do not do this all in one big loop. Write methods to test each portion, e.g. one to test rows, one to test columns, etc. If you do not, you will find it difficult to think through your program logically; it will also be harder to maintain when your employer wants to add a killer Sudoku checker.
As always, you will be graded on good programming practices, efficiency, clarity, etc.
For a grade of B:
Report all invalid Rows and Columns.
For a grade of A:
Also report all the invalid Blocks.
*******HERE IS MY CODE FOR THE PROGRAM:**********
using System; using System.Collections.Generic; using System.Linq; using System.Text;
namespace Program2 { class Program { static int[,] grid = new int[9, 9]; static string s; static void Init(ref int[,] grid) { for (int i = 0; i < 9; i++) { for (int j = 0; j < 9; j++) { grid[i, j] = (i * 3 + i / 3 + j) % 9 + 1; } } } static void Draw (ref int [,] grid, out string _s) { for (int x = 0; x < 9; x++) { for (int y = 0; y < 9; y++) { s += grid[x, y].ToString() + " "; } s += " "; } Console.WriteLine(s); _s = s; s = " "; } static void ChangeTwoCell(ref int [,] grid, int findValue1, int findValue2) { int xParam1, yParam1, xParam2, yParam2; xParam1 = yParam1 = xParam2 = yParam2 = 0; for (int i = 0; i < 9; i+=3) { for (int k = 0; k < 9; k+=3) { for (int j = 0; j < 3; j++) { for (int z = 0; z < 3; z++) { if (grid [i + j, k + z] == findValue1) { xParam1 = i + j; yParam1 = k + z; } if (grid [i + j, k + z] == findValue2) { xParam2 = i + j; yParam2 = k + z; } } } grid[xParam1, yParam1] = findValue2; grid[xParam2, yParam2] = findValue1; } } } static void Update (ref int[,] grid, int shuffleLevel) { for (int repeat = 0; repeat < shuffleLevel; repeat++) { Random rand = new Random(Guid.NewGuid().GetHashCode()); Random rand2 = new Random(Guid.NewGuid().GetHashCode()); ChangeTwoCell(ref grid, rand.Next(1, 9), rand2.Next(1, 9)); } } [STAThread] static void Main(string[] args) { Console.BackgroundColor = ConsoleColor.Blue; s = " "; string num; Init(ref grid); Update(ref grid, 10); Draw(ref grid, out num); Console.ReadKey(); } } }
THE .TXT FILES JUST HAVE A NAME OF :
sudoku-bad-1.txt(I am including below each .txt file the information in those .txt files)
2,1,5,6,4,7,3,9,8 3,6,8,9,5,2,1,7,4 7,9,4,3,8,1,6,5,2 5,8,6,2,7,4,9,3,1 1,4,2,5,8,3,8,6,7 9,7,3,8,1,6,4,2,5 8,2,1,7,3,9,5,4,6 6,5,9,4,2,8,7,1,3 4,3,7,1,6,5,2,8,9
INVALID ROW: 4 COL: 4 BLK: 4
sudoku-bad-2.txt
2,1,5,6,4,7,3,9,8 3,6,8,9,5,2,1,7,4 7,9,4,3,8,1,6,5,2 5,8,6,2,7,4,9,3,1 1,4,2,5,9,3,8,6,7 9,7,3,8,1,6,4,2,5 8,2,1,7,3,9,5,4,6 6,5,9,4,2,8,7,1,3 4,3,7,6,1,5,2,8,9
INVALID COL: 3 COL: 4
sudoku-bad-3.txt
2,1,5,6,4,3,7,9,8 3,6,8,9,5,1,2,7,4 7,9,4,3,8,6,1,5,2 5,8,6,2,7,9,4,3,1 1,4,2,5,9,8,3,6,7 9,7,3,8,1,4,6,2,5 8,2,1,7,3,5,9,4,6 6,5,9,4,2,7,8,1,3 4,3,7,1,6,2,5,8,9
INVALID BLK: 1 BLK: 2 BLK: 4 BLK: 5 BLK: 7 BLK: 8
sudoku-bad-4.txt
2,1,5,6,4,7,3,9,8 3,6,8,9,5,2,1,7,4 7,9,4,3,8,1,6,5,2 5,8,6,2,7,4,9,3,1 1,4,2,5,9,3,8,6,7 9,7,3,8,1,6,4,2,5 8,2,1,7,3,9,5,4,6 6,5,9,5,2,8,7,1,3 4,3,7,1,6,4,2,8,9
INVALID ROW: 7 ROW: 8 COL: 3 COL: 5
sudoku-good.txt
2,1,5,6,4,7,3,9,8 3,6,8,9,5,2,1,7,4 7,9,4,3,8,1,6,5,2 5,8,6,2,7,4,9,3,1 1,4,2,5,9,3,8,6,7 9,7,3,8,1,6,4,2,5 8,2,1,7,3,9,5,4,6 6,5,9,4,2,8,7,1,3 4,3,7,1,6,5,2,8,9
PLEASE NOTE THAT IN ALL OF THE SUDOKU-BAD TEXT FILES, IT SHOWS ALL THE INVALID INFORMATION IT SHOULD AND IN THE SUDOKU-GOOD TEXT FILE, IT JUST SHOWS THE SUDOKU PUZZLE BECAUSE IT RAN CORRECTLY. THIS IS HOW I NEED MY PROGRAM TO OUTPUT. PLEASE JUST LOOK AT THE INSTRUCTIONS AND HELP ME FIX MY CODE, I REALLY NEED TO GET A GOOD GRADE ON THIS!!!!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
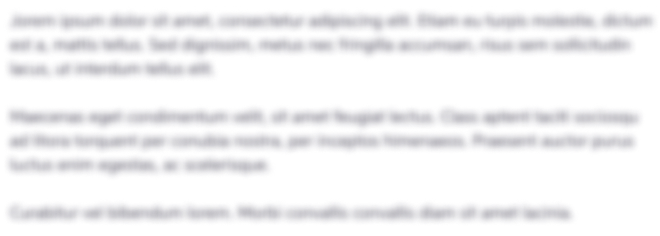
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started