Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In C++, create a main.cpp file from the code below that includes the following classes and instantiates them. The main class should not create any
In C++, create a main.cpp file from the code below that includes the following classes and instantiates them.
- The main class should not create any custom data types.
- The main class should create an object that returns animal blood type and type of animal
- The comments include the details of the class. If anything else is needed, please add.
Here is the code:
Animals.hpp
#ifndef CPP_ANIMALS #define CPP_ANIMALS #include using namespace std; class Animals { public: virtual string getBloodType() = 0; virtual string getMovementType() = 0; virtual string getConcreteType() = 0; }; #endif
Animals.cpp
#include "./Animals.hpp" /* * Since "Animals" only has pure virtual functions, so this file is empty */
ColdBlooded.hpp
#ifndef CPP_COLDBLOODED #define CPP_COLDBLOODED #include "./Animals.hpp" class ColdBlooded : public Animals { private: virtual string getBloodType(); }; #endif
ColdBlooded.cpp
#include "./ColdBlooded.hpp" string ColdBlooded::getBloodType() { return "ColdBlooded"; }
WarmBlooded.hpp
#include "./Animals.hpp" class WarmBlooded : public Animals { private: virtual string getBloodType(); };
WarmBlooded.cpp
#include "./WarmBlooded.hpp" string WarmBlooded::getBloodType() { return "WarmBlooded"; }
Reptiles.hpp
#ifndef CPP_REPTILES #define CPP_REPTILES #include "./ColdBlooded.hpp" class Reptiles : public ColdBlooded { private: virtual string getMovementType(); }; #endif
Reptiles.cpp
#include "./Reptiles.hpp" string Reptiles::getMovementType() { return "Reptiles"; }
Mammals.hpp
#ifndef CPP_MAMMALS #define CPP_MAMMALS #include "./WarmBlooded.hpp" class Mammals : public WarmBlooded { private: virtual string getMovementType(); }; #endif
Mammals.cpp
#include "./Mammals.hpp" string Mammals::getMovementType() { return "Mammals"; }
Lizards.hpp
#include "./Reptiles.hpp" class Lizards : public Reptiles { private: string getConcreteType(); };
Lizards.cpp
#include "./Lizards.hpp" string Lizards::getConcreteType() { return "Lizards"; }
Snakes.hpp
#include "./Reptiles.hpp" class Snakes : public Reptiles { private: string getConcreteType(); };
Snakes.cpp
#include "./Snakes.hpp" string Snakes::getConcreteType() { return "Snakes"; }
Humans.hpp
#include "./Mammals.hpp" class Humans : public Mammals { private: string getConcreteType(); };
Humans.cpp
#include "./Humans.hpp" string Humans::getConcreteType() { return "Humans"; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
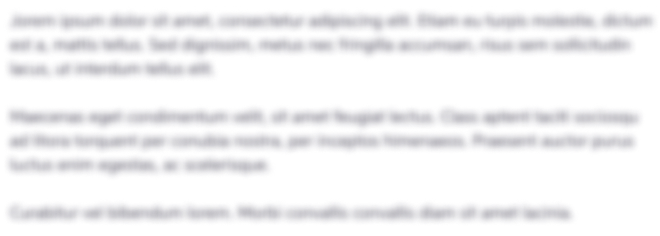
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started