Question
In C++ Language: CS 161 Assignment #5 User Defined Functions Program requirements: You must use the function prototypes given below. Copy and paste them above
In C++ Language:
CS 161 Assignment #5 User Defined Functions
Program requirements:
- You must use the function prototypes given below. Copy and paste them above the main function of your source code.
- In the main function, create a menu system to test the functions.
- Assume perfect input.
- Do not use infinite loops with break statements to get out of them. If you naturally program using infinite loops then come up with a Boolean flag mechanism instead.
- Use exactly one return statement in main the last statement.
Function 1
Write a function that reads one or more positive numbers from the user, outputs only the count of positive numbers entered, and then returns the largest number. Once the value is returned the calling function needs to output the answer. The function prototype should be as follows:
int largest();
This function does not take any parameters, and it returns the largest integer in the common input stream (i.e., that means you must use cin in this function). Use a while loop to read in integers one at a time and keep track of the largest number. Do not use an array in this function we have not covered that yet. The function should exit whenever it encounters either zero or a negative number in the input.
Function 2
Write a function that reads one number from the user and returns the number of odd and even digits within that number. In order to test if it is working correctly after the calling this function needs output the results. This function will be a void function in order to effectively return multiple values. Here is the function prototype:
void numberCount(int &oddCount, int &evenCount);
For example, when the user enters 382045, the number of odd digits is 2 and even digits is 4 (treating zero as an even number since that is how mathematicians view it). You must use integer math in this problem (hint review integer division and integer remainder operations).
Function main
The main function must display a simple menu as follows:
Welcome to my program! Please enter a-c to choose one of the following:
(a) Find the largest of a list of positive numbers (type 0 to quit).
(b) Count the odd and even digits in a number.
(c) Quit this program.
If the user picks something other than a-c then display a friendly error message and continue the program. Based on what character the user picks, use either a switch or an if statement and output the corresponding answer. This menu should be in a loop so it continues to display after each turn until the user enters c to quit.
Program template:
Copy the code below and fill in the blanks. If you like extend these comments listing all variables in the program.
/*CS 161 Assignment 5: User Defined Functions
Filename: a05.cpp
Date: ___
Summary: (describe the programs purpose, then describe how the input is transformed into the output)
Variables (data type, name, purpose):
___
Equations (if any):
___
*/
Design Considerations:
- Please name your file a05.cpp.
- No global variables. All variables must be inside main().
- Above main() write code comments describing the assignment.
- Your application must be data driven meaning your answer should be built from user input.
- Points will be awarded for the following attributes of your solution.
- If your program does not compile you will get at most 30% of the maximum points irrespective of what the error is.
- If your program compiles, then points are awarded for correctness, readability, and documentation. Your source code needs to have good form. In particular, pay attention to your indentation, capitalization, and so forth. Be sure to read the class C++ style guide before working on this problem. However, if you follow the style used in the book you will be fine. Having good documentation requires that you have comments delineating your name, the date, assignment name/number, the assignment description (see above), and outside people or Internet sources that helped you complete this assignment.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
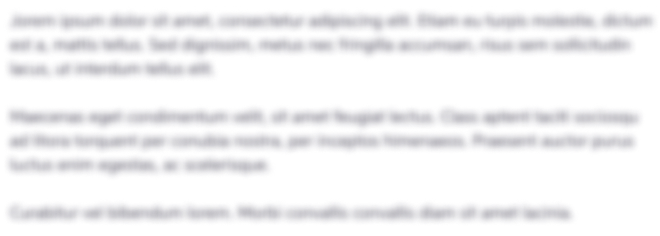
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started