Question
In C language. #includelab5-q2.h #include #include #include #include #include /* Initialize the tree with a root #1 */ /* Create an empty BTree first */
In C language.
#include"lab5-q2.h"
#include
#include
#include
#include
#include
/* Initialize the tree with a root #1 */
/* Create an empty BTree first */
/* The root (key, value) is stored at (key[0], value[0]) */
/* If key is unused, set key = INT_MAX, value = -2100 */
void tree_init(BTree **T, int key, double value, int max_size){
// You code here
}
/* Initialize the tree with arrays #2 */
/* Create an empty BTree first */
/* n defines the number of (key,value) pairs of the array */
/* If key is unused, set key = INT_MAX, value = -2100 */
void tree_init_with_array(BTree **T, int *key, double *value, int n, int max_size){
// You code here
}
/* Initialize the empty tree with height of root #3 */
/* Based on the height, calculate the max_size */
/* Create an empty BTree with max_size */
/* If key is unused, set key = INT_MAX, value = -2100 */
void tree_init_with_height(BTree **T, int height){
// You code here
}
/* Get the index of parent #4 */
/* Return the index of the parent */
/* Return -2100 if the parent index is invalid or unused */
int tree_parent(BTree *T, int child_index){
// You code here
return 0;
}
/* Get the index of left child #5 */
/* Return the index of the left child */
/* Return -2100 if the left child index is invalid or unused */
int tree_lchild(BTree *T, int parent_index) {
// You code here
return 0;
}
/* Get the index of right child #6 */
/* Return the index of the right child */
/* Return -2100 if the right child index is invalid or unused */
int tree_rchild(BTree *T, int parent_index){
// You code here
}
/* Insert or update a node #7 */
/* If key exists in T, update the value */
/* If key not in T, insert (key, double) as left child of parent_index*/
/* If the left child cannot be inserted, then do nothing */
/* If the left child is used by other key, then do nothing */
void tree_insert_lchild(BTree *T, int parent_index, int key, double value){
// You code here
}
/* Insert or update a node #8 */
/* If key exists in T, update the value */
/* If key not in T, insert (key,double) as right child of parent_index*/
/* If the right child cannot be inserted, then do nothing */
/* If the right child is used by other key, then do nothing */
void tree_insert_rchild(BTree *T, int parent_index, int key, double value){
// You code here
}
/* Find the value based on the key in the tree T or not #9 */
/* If key in T, return the corresponding value */
/* If key not in T, return -2100.00 */
double tree_key_find(BTree *T, int key){
// You code here
return 0.;
}
/* Find the value based on the index in the tree T or not #10 */
/* If index is used, return the corresponding value */
/* If index is unused or exceeding the max_size, return -2100.00 */
double tree_index_find(BTree *T, int index){
// You code here
return 0.;
}
/* Free the tree *T, if *T is not NULL #11 */
/* Assign NULL to *T after free */
void tree_free(BTree **T){
// You code here
}
/* The print function print the tree in an output string using postorder traversal #12 */
/* The number in the bracket is key, number after bracket is value */
/* If the tree looks like */
/* (0)1.7 */
/* | \ */
/* (1)1.2 (2)1.8 */
/* | | \ */
/* (4)0 (3)2 (6)5 */
/* Then, the output string is "(4)0.00 (1)1.20 (3)2.00 (6)5.00 (2)1.80 (0)1.70 " */
/* You can assume the number of nodes is not more than 100 */
char *tree_print(BTree *T){
char *output = malloc(sizeof(char)*3000);
// You code here
return output;
}
Question 2: Binary Tree - Array Implementation (30\%) You are going to implement the binary tree abstract data type using array implementation. The header file "lab5-q2.h" and the description of the functions are as follows. Your program should not contain main(). Name your program as "lab5-q2.cStep by Step Solution
There are 3 Steps involved in it
Step: 1
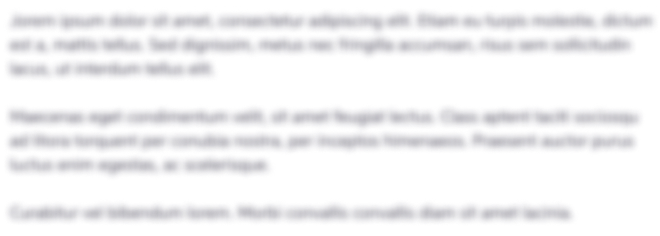
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started