Question
(!) IN C LANGUAGE: I've been working on this for most of the day and can't seem to get much of it working, so help
(!) IN C LANGUAGE: I've been working on this for most of the day and can't seem to get much of it working, so help would be greatly appreciated. This is a program that builds off of a previous program I did, so I will provide that code as well (not including the new functions I've attempted, but can't get to work).
The goal of the program is to read a file, store the input in a linked list, sort it by either first or last name, and then print it out in that order. The information in the file is separated by commas, and the birthday month is written out and not a number. (i.e. "January" instead of "1")
*Please also provide updated driver.c code and do not change the "starter" code I've provided. Thank you! :)
Here's the assignment instructions:
This lab will be built off of your linked list code from Lab 3. While you will not have to check for duplicate entries, your code should be able to read from an input file, store data in a linked list, and print output to a file. We will be adding several additional functions to Lab 3.
Requirements:
Function Pointers: In this lab, you will be sorting a linked list either by first name or last name. You should only have one sortList function, but you will pass a function pointer to one of two compare functions to specify how sortList should sort the linked list.
Date Validation: You will have to validate that the date stored in a node is valid. To do this, you will have to check that the day is not negative and also not higher than the number of days in the month. You will also need to check for leap years.
Sorting: In this lab, you will take in an additional command line argument which will determine whether you should sort your list by first name or by last name. You can use any sorting algorithm (or make up your own!) to sort your list as long as you keep the function specs the same.
Files: For this lab, you will check to make sure that a valid date is being read in from the input file. You are then going to sort the linked list (sort is determined by a command line argument). Your solution will consist of the following files: driver.c This file will have minimal code. Most of your code should be in your functions.c file. You will have to change the number of arguments you check for because you will be taking in an additional command line argument. In this file, you should keep the same code as in lab 3 but add: Take in an additional command line argument, either 1 or 2. If ran with 1, then you should sort the list by first name. If 2, then sort by last name. Call sortList to sort the linked list (different calls depending on last name or first name) sortList should be called after createList, but before printList. Your driver shouldnt have more code than necessary. DO NOT include functions.c in your driver.c file. As a rule, you should not include .c files. In this lab, doing so will screw up the autograder.
functions.h This file will include the definitions for both of your typedef-ed structs. You will also have your function definitions in this file. Add the new functions you are implementing to this file. Header guards are required for this file. You will also need to have a function pointer in this file. The functions pointed to by this function pointer should compare two nodes (take in two node*) and return an int: 0 -- if the nodes are equal positive -- if the first node is "greater" negative -- if the second node is "greater" You will have two functions in functions.c that fit these specifications: compare_by_lastname() and compare_by_firstname().
functions.c In addition to the functions from lab 3, you will have to implement the following functions:
bool checkInvalidDate(node_t *node); This function should take in a node ptr and check that the node being pointed to contains a valid date. We will not give you incorrectly spelled months, but you must check that the day is in the month. To do this, you will also need to check for leap years (I did this in a helper function). If a node has an invalid date, dont add it to the list (or dont print it out). However you chose to implement, it shouldnt be in your output file.
void sortList(node_t **head,
As far as algorithms go, I recommend using bubble sort for those totally new to sorting. Sorting your list is largely a choose your own adventure. As long as you keep the given functions the same, you can use any sorting algorithm and any helper functions you would like. I recommend adding a helper function called swapNode that takes in two node pointers (node*) and switches those two nodes in the linked list. You should use the compare function that you passed in to compare nodes. You should not have a separate sort function for last name and for first name.You can use the same logic and just call a different compare function.
int compare_by_lastname(node_t *a, node_t *b); This function should take in two node pointers and compare the two nodes by last name. You should return 0 if the strings are the same, a positive number if the first node is "greater", and a negative number if the second node is "greater". int compare_by_firstname(node_t *a, node_t *b); This function should take in two node pointers and compare the two nodes by first name. You should return 0 if the strings are the same, a positive number if the first node is "greater", and a negative number if the second node is "greater". The two compare functions should be virtually identical. These functions are required, but you are free to implement helper functions as you see fit. As long as the required functions (with the correct parameters and return values) are present, the implementation is flexible.
My current code is:
driver.c:
functions.c:
functions.h:
Thank you for the help.
\#ifndef FUNCTIONS_H \#define FUNCTIONS_H \#include \#include "stdbool.h" //add birthday struct here typedef struct birthday \{ int day; int year; char month[50]; \} birthday_t; //add node t struct here (this struct will represent a node in the // Iinked list) typedef struct node \{ char firstName[50]Step by Step Solution
There are 3 Steps involved in it
Step: 1
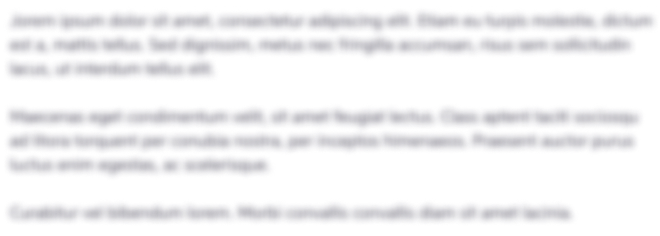
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started