Question
In c++ Milestone 1 Create a class called Words . In Words , Create two private int variables minlen, maxlen. Create an overloaded constructor to
In c++
Milestone 1
Create a class called Words.
In Words,
Create two private int variables minlen, maxlen. Create an overloaded constructor to set these variables.
Create a private int variable called count
Create a private pointer to a string: string * choices
Create a function int count_candidates(). This function will open the file enable1.txt and count all the words in the file whose lengths are between minlen and maxlen. This function will return the resulting count.
Create a function void load_words().
In this function, create a dynamic string array of size count. Have choices point to this newly created array.
This function will open the file enable1.txt again. Similar to count_candidates(), this function will go through the entire file identifying all the words whose lengths are between minlen and maxlen. Add the qualifying words into the choices array as they are identified.
Create a function string pick_word(). This function will return a single random string out of the choices array.
In the constructor, call count_candidates() and load_words().
You might want to consider disposing of choices in a destructor.
For this milestone, use main to instantiate an instance of Words. Ask the user for two integer values as min and max. Pass these two values into your Words instances constructor. Also, test to see if you can display a single word by calling pick_word().
Sample main() for Milestone 1
int main() { int min, max; cout << "Enter min: "; cin >> min; cout << "Enter max; "; cin >> max; Words words(min, max); cout << words.pick_word() << endl; }
Sample Interaction for Milestone 1
[Run your program] Enter min: 27 Enter max: 29 electroencephalographically
Milestone 2
Create a class called Hangman. In this class,Create the following private variables: char word[40], progress[40], int word_length.
word will be used to store the current word
progress will be a dash representation of the the current word. It will be the same length as the current word. If word is set to apple, then progress should be at the beginning of the game. As the user guesses letters, progress will be updated with the correct guesses. For example, if the user guesses p then progress will look like pp
Create a private function void clear_progress(int length). This function will set progress to contain all dashes of length length. If this function is called as clear_progress(10), this will set progress to . Remember that progress is a character array; dont forget your null terminator.
Create the following protected data members: int matches, char last_guess, string chars_guessed, int wrong_guesses, int remaining.
Create a public function void initialize(string start). This function will initialize chars_guessed, wrong_guesses. Set remaining to 6. Use strcpy() to set word to the starting word passed as start. Set word_length to the length of word. Call clear_progress in this function.
Create a constructor which passes in a string parameter. Call initialize() from within the constructor
Create getter functions for word, progress, and remaining.
Create a function bool is_word_complete(). This function calls the strcmp() function to compare if progress equals word.
Create a protected function bool check(char user_guess). This function will accept a single character (this character is what the user will guess). This function will return true if user_guess is found in word and return false if the user_guess is not in word. This function will update progress with the correct letters updating the appropriate positions with all occurrences of user_guess. If user_guess is not found in the word, do not update progress. Also, be sure to update chars_guessed (use the += operator), wrong_guesses, and remaining. Make sure you detect within chars_guessed if user_guess has previously been entered, and make sure not to penalize the user for guessing the same non-matching letter multiple times. In other words, dont update chars_guessed, wrong_guesses and remaining for repeated user guesses of the same letter. Only update chars_guessed with the letters not found in the word.
Create a class HangmanConsole which publicly inherits from Hangman.
Write an overloaded constructor passing in a single string variable start. This constructor will call Hangmans overloaded constructor in its initializer list.
Write a function void show_status(int stage). This function implements a switch statement. See the start of this below you will have to fill in the rest of the cases.
switch (stage) { case 0: cout << " +----- "; cout << " | "; cout << " | "; cout << " | "; cout << " | "; cout << " ----- "; break; }
Write a function void show_info(). This function will call get_progress(), show_status() and text showing:
how many matches were found on the users last guessed character (last_guess and matches)
how many wrong guesses were made (wrong_guesses)
how many attempts are remaining (remaining)
What letters have been guessed so far (chars_guessed)
Overload the insertion operator (>>). In this overload, ask the user to enter a single character. Call the check() function for the HangmanConsole object, passing in the character entered by the user
See the sample main below for Milestone 2
Sample main() for Milestone 2
int main() { Words words(7,10); // words between 7 and 10 chars long HangmanConsole game(words.pick_word()); cout << "HANGMAN" << endl << "-------" << endl << endl; cout << "Your word is: " << game.get_progress() << endl; while (!game.is_word_complete() && game.get_remaining() > 0) { cout << endl; cout << "Enter your guess: "; cin >> game; system("clear"); // NON-PORTABLE, ONLY WORKS ON LINUX game.show_info(); } return 0; }
Milestone 3
Modify the Words class and add a public function void reset(int min, int max). This function will set the value of minlen and maxlen using min and max values from the functions parameters. reset() will also check to see if choices is NULL; if it is not NULL, then it will delete it (similar to your destructor). Then, this function will call count_candidates() and load_words(). This function will effectively allow you to change the range of the word lengths and reload the qualifying words.
Create a global, non-class function int show_menu(). This will show a menu to the user as below (or something similar).
1. Play the computer (auto select random range) 2. Play the computer (min: 7, max: 12) 3. Play the computer (user selects/changes range) 4. Play the computer (use same range stored in 3) 5. Two player mode (user1 enters the word, user2 guesses) Enter choice:
Create a function void get_range_num(string prompt, int min, int max, int & number). This function will prompt the user to enter an integer. If the integer entered by the user falls outside of min and max, keep prompting the user. Values within the range of min and max will be accepted and returned via the reference parameter, number.
This function will be used when prompting the user to enter the length range for option 3 in the menu. DEFENSIVE PROGRAMMING (OPTIONAL but RECOMMENDED): Should you allow the user to enter values for min that are larger than max?
What happens if menu option 4 is selected before menu option 3 is called? Should it also ask for user input first?
You will need to call the reset() function you created in step 1 of Milestone 3 anytime you change the range of word lengths. Realize that Option 5 of the menu above does not use the Words class or any instantiated objects of the Words class.
Create as many functions and variables as you need for Milestone 3. Not all of them have been specified above you can create your own functions as you see fit.
Takeaways
Classes Words and Hangman have no relationship. Hangman can be used without Words you can simply pass into its constructor any word.
Hangman contains all the functionality to implement the game taking a word, comparing user guesses, keeping count of attempts, etc without implementing where the word comes from, or where the users guessed character comes from or how the games progress is displayed to the user.
HangmanConsole inheriting from Hangman gives it the playability of the game but also provides the input and output to make the game visibly playable from the console. In theory, you can create other child classes HangmanGUI, HangmanIOS, HangmanAndroid which will provide the functionality needed for those various platforms. Keeping the guts of the gameplay in the parent Hangman allows it to be usable in various implementations.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
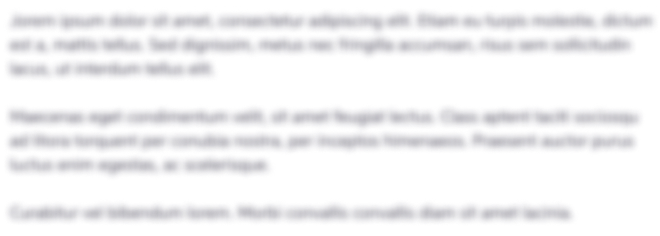
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started