Question
In C only please Project 4 simulation with conway's rules for life - revisited due before 4/30 11:59pm, autograded by project 4 zylab, limited to
In C only please
Project 4
simulation with conway's rules for life - revisited
due before 4/30 11:59pm, autograded by project 4 zylab, limited to 10 submissions.
We will be using the concepts from project 2 to test the more recent concepts of linked lists, pointers, and file I/O. The initial data points will be stored in a file (specification of the layout of the file below), each grid of values will be dynamically allocated and then referenced via a pointer in a linked list.
More details are:
input file
first line will list the number of time steps to simulate
second line will include number of rows followed by the number of columns in the grid to be simulated
remaining lines in the file will have the positions of the initial cells that are "alive"
dynamically create nodes that use the following struct
struct node { int rows; int columns; int * grid; // a pointer to the grid that should be of size rows*columns struct node *next_step; // a pointer to the node that holds the grid for the next time step };
you will create a linked list of nodes where each node is a single time step
the begin pointer should point to the initial grid, and each time step should follow.
no array notation is to be used, so no []'s should appear in your code.
You MUST create the following functions
struct node *createInitialNode(FILE *input, int *numsteps); // read data from file pointer input and return a dynamically created node with the data and the number of time steps to simulate in numsteps void nextStep(struct node *begin); // add a struct node to the end of the list pointed to by begin for the next time step, so use data in the last node in the list to create the new timestep. void printList(struct node *begin); // print out all the grids contained in the list pointed to by begin.
You should use your code from project 2. If you had issues with the functions from project 2, you should see the professor or a TA ASAP!!!!!!
The autograder will test the three functions above (as well as the functionality of your new array creation) and produce a score of 65 points. The remaining 35 points will be for style per @7
Notes:
by using the linked list there is no limit to the number of time steps to simulate, recall project 2 had a max number of time steps.
by using dynamically allocated memory there is no limit to the grid size, recall project 2 had a max grid size.
when grading the style points, if any []'s are seen in the code, a 0 will be given for the autograded test cases.
Example input file sampleinput.txt
contains:
3 10 12 7 7 7 8 7 9 8 7 9 8
So your code should display the following:
Welcome to Connor's game of Life Please enter filename 000000000000 000000000000 000000000000 000000000000 000000000000 000000000000 000000000000 000000011100 000000010000 000000001000 000000000000 000000000000 000000000000 000000000000 000000000000 000000000000 000000001000 000000011000 000000010100 000000000000 000000000000 000000000000 000000000000 000000000000 000000000000 000000000000 000000011000 000000010100 000000010000 000000000000 000000000000 000000000000 000000000000 000000000000 000000000000 000000000000 000000011000 000000110000 000000001000 000000000000We will be using the concepts from project 2 to test the more recent concepts of linked lists, pointers, and file lI/O. The initial data points will be stored in a file (specification of the layout of the file below), each grid of values will be dynamically allocated and then referenced via a pointer in a linked list More details are: input file first line will list the number of time steps to simulate second line will include number of rows followed by the number of columns in the grid to be simulated remaining lines in the file will have the positions of the initial cells that are "alive" dynamically create nodes that use the following struct struct node ( int rows; int columns; int grid; // a pointer to the grid that should be of size rows*columns struct node *next_step; // a pointer to the node that holds the grid for the next time step you will create a linked list of nodes where each node is a single time step the begin pointer should point to the initial grid, and each time step should follow no array notation is to be used, so no I's should appear in your code You MUST create the following functions struct node *createInitialNode (FILE *input, int *numsteps); // read data from file pointer input and return a dynamically created node with the data and the number of time steps to simulate in numsteps void nextStep(struct node *begin); // add a struct node to the end of the list pointed to by begin for the next time step, so use data in the last node in the list to create the new timestep. void printList (struct node *begin) // print out all the grids contained in the list pointed to by begin You should use your code from project 2. If you had issues with the functions from project 2, you should see the professor or a TA ASAP!! The autograder will test the three functions above (as well as the functionality of your new array creation) and produce a score of 65 points. The remaining 35 points will be for style per @7 Notes: by using the linked list there is no limit to the number of time steps to simulate, recall project 2 had a max number of time steps by using dynamically allocated memory there is no limit to the grid size, recall project 2 had a max grid size when grading the style points, if any I's are seen in the code, a 0 will be given for the autograded test cases Example input file sampleinput.txt contains 10 12 7 8 7 9 8 7 98 So your code should display the following Welcome to Connor's game of Life Please enter filename 000000011100 000000010000 000000001000 000000001000 000000011000 000000010100 000000011000 000000010100 000000010000 000000011000 000000110000 000000001000 We will be using the concepts from project 2 to test the more recent concepts of linked lists, pointers, and file lI/O. The initial data points will be stored in a file (specification of the layout of the file below), each grid of values will be dynamically allocated and then referenced via a pointer in a linked list More details are: input file first line will list the number of time steps to simulate second line will include number of rows followed by the number of columns in the grid to be simulated remaining lines in the file will have the positions of the initial cells that are "alive" dynamically create nodes that use the following struct struct node ( int rows; int columns; int grid; // a pointer to the grid that should be of size rows*columns struct node *next_step; // a pointer to the node that holds the grid for the next time step you will create a linked list of nodes where each node is a single time step the begin pointer should point to the initial grid, and each time step should follow no array notation is to be used, so no I's should appear in your code You MUST create the following functions struct node *createInitialNode (FILE *input, int *numsteps); // read data from file pointer input and return a dynamically created node with the data and the number of time steps to simulate in numsteps void nextStep(struct node *begin); // add a struct node to the end of the list pointed to by begin for the next time step, so use data in the last node in the list to create the new timestep. void printList (struct node *begin) // print out all the grids contained in the list pointed to by begin You should use your code from project 2. If you had issues with the functions from project 2, you should see the professor or a TA ASAP!! The autograder will test the three functions above (as well as the functionality of your new array creation) and produce a score of 65 points. The remaining 35 points will be for style per @7 Notes: by using the linked list there is no limit to the number of time steps to simulate, recall project 2 had a max number of time steps by using dynamically allocated memory there is no limit to the grid size, recall project 2 had a max grid size when grading the style points, if any I's are seen in the code, a 0 will be given for the autograded test cases Example input file sampleinput.txt contains 10 12 7 8 7 9 8 7 98 So your code should display the following Welcome to Connor's game of Life Please enter filename 000000011100 000000010000 000000001000 000000001000 000000011000 000000010100 000000011000 000000010100 000000010000 000000011000 000000110000 000000001000
Step by Step Solution
There are 3 Steps involved in it
Step: 1
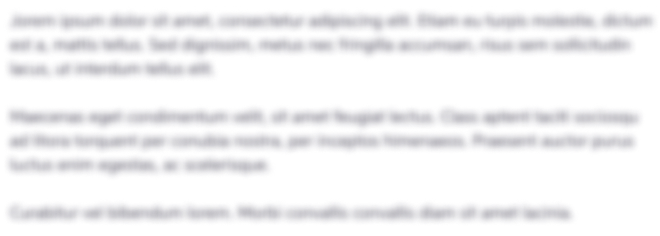
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started