Question
In C Only Reading and printfing from a binary file The schedule will be printed in a format that is similar to a table. It
In C Only
Reading and printfing from a binary file
The schedule will be printed in a format that is similar to a table. It will be printed from 8:00 am to 9:30 pm in half an hour intervals. It will print the schedule in the following format "08:00 am - name". It is assumed there will be no overlapping events. The name of the event will be written beside the time from start to finish and left blank when there are no events. For example, breakfast starts at 8:00 am for 30 minutes and commute starts at 9:00 am for 50 minutes.
/*****
* Self-defined Data Structures
*****/
/* enumerate a Boolean datatype for convenience */
typedef int abstractBoolean;
enum abstractBoolean {
false,
true
};
typedef abstractBoolean boolean;
/* Start time is in hours, duration is in minutes */
typedef struct eventNode {
char name[64];
int startTime;
boolean halfHour;
int duration;
} Event;
/*******************Function prototypes***********************/
/* Writes to a binary file */ void createBinaryFile(char *fileame);
/* Prints a schedule of events from a list of events */ void printSchedule(Event *list, int listLength);
/*******************Main***********************/
int main(int argc, char *argv[]) { char *fileName = "lab08/docs/schedule.bin"; createBinaryFile(fileName); return 0; }
/*******************Functions***********************/
/* Writes to a binary file */ void createBinaryFile(char *name) { int i; FILE *ptr = fopen(name,"wb"); char names[][64] = {"breakfast", "commute", "meeting", "lunch", "seminar", "gym", "commute", "dinner"}; int starts[] = {8, 8, 10, 12, 13, 16, 18, 19}; boolean halfHours[] = {false, true, false, false, false, true, false, false}; int durations[] = {30, 45, 75, 60, 180, 80, 45, 30}; for(i = 0; i < 8; i++) { Event new; sprintf(new.name, "%s", names[i]); new.startTime = starts[i]; new.halfHour = halfHours[i]; new.duration = durations[i]; fwrite(&new, sizeof(Event), 1, ptr); } fclose(ptr); }
void printSchedule(Event *list, int listLength){
/**-----------------------> This is the function that needs to be completed***************/
/* Main will need a few lines of code to all this function */
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
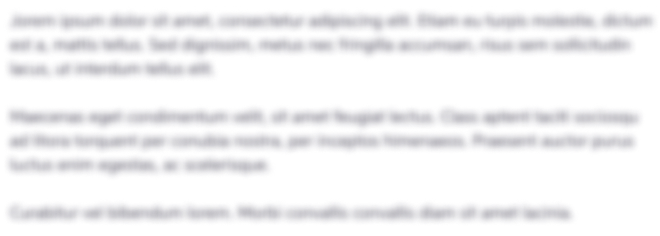
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started