Question
In C++ Please alter testProgGraph.cpp so it displays requested output. Prompt: Write a program that outputs the nodes of a graph in a depth-first traversal
In C++
Please alter testProgGraph.cpp so it displays requested output.
Prompt: Write a program that outputs the nodes of a graph in a depth-first traversal and in a breadth-first traversal
same question and similar code to https://www.chegg.com/homework-help/questions-and-answers/hello-code-everything-implemented-done-read-file-answer-look-like-picture-tia-maincpp-file-q29164053
Input:
11 0 1 5 -999 1 2 3 5 -999 2 4 -999 3 -999 4 3 -999 5 6 -999 6 8 -999 7 3 8 -999 8 10 -999 9 4 7 10 -999 10 -999
Output:
Enter input filename: in.txt
Depth First Traversal: 0 1 4 3 2 5 7 8 6 9
Breadth First Traversal: 0 1 3 4 2 5 7 8 6 9
testProgGraph.cpp
#include
#include
#include "graphType.h"
using namespace std;
int main()
{
cout <<"Depth First Traversal:"<< ???????
<< endl;
cout << "Breath First Traversal:"<< ???????
<< endl;
return 0;
}
Here are the rest of the files that the program uses.
graphType.h
#ifndef H_graph
#define H_graph
#include
#include
#include
#include "linkedList.h"
#include "unorderedLinkedList.h"
#include "linkedQueue.h"
using namespace std;
class graphType
{
public:
bool isEmpty() const;
//Function to determine whether the graph is empty.
//Postcondition: Returns true if the graph is empty;
// otherwise, returns false.
void createGraph();
//Function to create a graph.
//Postcondition: The graph is created using the
// adjacency list representation.
void clearGraph();
//Function to clear graph.
//Postcondition: The memory occupied by each vertex
// is deallocated.
void printGraph() const;
//Function to print graph.
//Postcondition: The graph is printed.
void depthFirstTraversal();
//Function to perform the depth first traversal of
//the entire graph.
//Postcondition: The vertices of the graph are printed
// using the depth first traversal algorithm.
void dftAtVertex(int vertex);
//Function to perform the depth first traversal of
//the graph at a node specified by the parameter vertex.
//Postcondition: Starting at vertex, the vertices are
// printed using the depth first traversal algorithm.
void breadthFirstTraversal();
//Function to perform the breadth first traversal of
//the entire graph.
//Postcondition: The vertices of the graph are printed
// using the breadth first traversal algorithm.
graphType(int size = 0);
//Constructor
//Postcondition: gSize = 0; maxSize = size;
// graph is an array of pointers to linked lists.
~graphType();
//Destructor
//The storage occupied by the vertices is deallocated.
protected:
int maxSize; //maximum number of vertices
int gSize; //current number of vertices
unorderedLinkedList
//adjacency lists
private:
void dft(int v, bool visited[]);
//Function to perform the depth first traversal of
//the graph at a node specified by the parameter vertex.
//This function is used by the public member functions
//depthFirstTraversal and dftAtVertex.
//Postcondition: Starting at vertex, the vertices are
// printed using the depth first traversal algorithm.
};
bool graphType::isEmpty() const
{
return (gSize == 0);
}
void graphType::createGraph()
{
ifstream infile;
char fileName[50];
int vertex;
int adjacentVertex;
if (gSize != 0) //if the graph is not empty, make it empty
clearGraph();
cout << "Enter input file name: ";
cin >> fileName;
cout << endl;
infile.open(fileName);
if (!infile)
{
cout << "Cannot open input file." << endl;
return;
}
infile >> gSize; //get the number of vertices
for (int index = 0; index < gSize; index++)
{
infile >> vertex;
infile >> adjacentVertex;
while (adjacentVertex != -999)
{
graph[vertex].insertLast(adjacentVertex);
infile >> adjacentVertex;
} //end while
} // end for
infile.close();
} //end createGraph
void graphType::clearGraph()
{
for (int index = 0; index < gSize; index++)
graph[index].destroyList();
gSize = 0;
} //end clearGraph
void graphType::printGraph() const
{
for (int index = 0; index < gSize; index++)
{
cout << index << " ";
graph[index].print();
cout << endl;
}
cout << endl;
} //end printGraph
void graphType::depthFirstTraversal()
{
bool *visited; //pointer to create the array to keep
//track of the visited vertices
visited = new bool[gSize];
for (int index = 0; index < gSize; index++)
visited[index] = false;
//For each vertex that is not visited, do a depth
//first traverssal
for (int index = 0; index < gSize; index++)
if (!visited[index])
dft(index,visited);
delete [] visited;
} //end depthFirstTraversal
void graphType::dft(int v, bool visited[])
{
visited[v] = true;
cout << " " << v << " "; //visit the vertex
linkedListIterator
//for each vertex adjacent to v
for (graphIt = graph[v].begin(); graphIt != graph[v].end();
++graphIt)
{
int w = *graphIt;
if (!visited[w])
dft(w, visited);
} //end while
} //end dft
void graphType::dftAtVertex(int vertex)
{
bool *visited;
visited = new bool[gSize];
for (int index = 0; index < gSize; index++)
visited[index] = false;
dft(vertex, visited);
delete [] visited;
} // end dftAtVertex
void graphType::breadthFirstTraversal()
{
linkedQueueType
bool *visited;
visited = new bool[gSize];
for (int ind = 0; ind < gSize; ind++)
visited[ind] = false; //initialize the array
//visited to false
linkedListIterator
for (int index = 0; index < gSize; index++)
if (!visited[index])
{
queue.addQueue(index);
visited[index] = true;
cout << " " << index << " ";
while (!queue.isEmptyQueue())
{
int u = queue.front();
queue.deleteQueue();
for (graphIt = graph[u].begin();
graphIt != graph[u].end(); ++graphIt)
{
int w = *graphIt;
if (!visited[w])
{
queue.addQueue(w);
visited[w] = true;
cout << " " << w << " ";
}
}
} //end while
}
delete [] visited;
} //end breadthFirstTraversal
//Constructor
graphType::graphType(int size)
{
maxSize = size;
gSize = 0;
graph = new unorderedLinkedList
}
//Destructor
graphType::~graphType()
{
clearGraph();
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
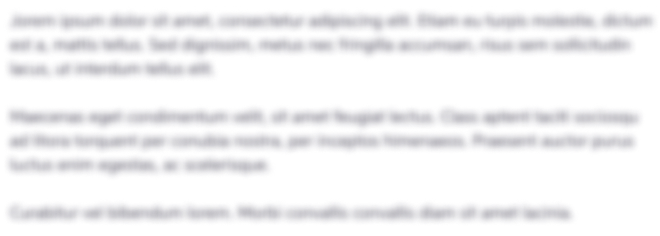
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started