Question
- IN C++ PLEASE AND ALL IN ONE FILE Homework Assignment 1 In this assignment, you are going to design a directory of restaurants that
- IN C++ PLEASE AND ALL IN ONE FILE
Homework Assignment 1
In this assignment, you are going to design a directory of restaurants that helps restaurant owners advertise their business and users find great local restaurants. Each restaurant creates a profile with basic listing information such as restaurant name, location, contact information, type of food it serves, rating, and number of reviewers. Users can look up this directory to find places of interest and can submit a review to a restaurant service using a one to five-star rating system. The program allows several functionalities such as: adding a new restaurant listing to the directory, deleting a listing if the restaurant closed, displaying restaurants details, finding specific restaurant(s), and rating a restaurant.
To do that, you need to create a structure, Restaurant, that holds individual restaurants details. The Restaurant struct contains the following fields:
struct Restaurant {
string name; string address; string city; string state; int zipcode; string type; string website; float rating; int reviewers;
};
Then, in your main program, you need to:
- declare a vector of Restaurant structs, named restaurants, to hold the data for local restaurants.
- process a transaction file, RestaurantsTrans.txt. Each line in the file contains a specific commandsuch as AddListing, RemoveListing, DisplayAll, FindByName, FindByCriteria, Rate, and FindTopRated. The line also contains all necessary data to process the command. Each
command must be implemented in a separate function. The commands are:
AddListing RestaurantName RestaurantAddress RestaurantCity RestaurantState RestaurantZipCode RestaurantType RestaurantWebsite
-This command creates a new Restaurant record (object) and adds it to the restaurants vector. You should read the restaurant details from the transaction file (RestaurantName, RestaurantAddress, RestaurantCity, RestaurantState, RestaurantZipCode, RestaurnatType, RestaurantWebsite). You can simply use the push_back function to add a Restaurant object to the end of the vector. Make sure to set rating and number of reviewers initially to zeros to indicate that the restaurant has not been rated yet. The Rate function allows users to set the rating and number of reviewers later.
DisplayAll
-This command displays summary details of all restaurants listed in the system in a neat table format. Details include a restaurants name, city, type of food it serves, rating, and number of reviewers. Note that rating is a float and should be printed with 2 digits of precision. A sample display is shown below:
Restaurant City Type Rating Reviewers
---------------------------------------------------------------------
LaPizzeria SanDiego italian 0.00 0
...
FindByName RestaurantName
This command finds and prints the details of all restaurants whose names either match the keyword, RestaurantName, or contain that keyword in their names. For example, ifRestaurantName is Ristorante, you should display the following restaurants in the format below:
FindByName Ristorante OlivioRistorante, italian 4320CaminoDr, Carlsbad CA 92008 www.olivio.com rating: 4.08 (6 reviews)
MariaRistorante, italian 6534SpringburstDr, Carlsbad CA 92008 www.mariaristorante.com rating: 3.00 (3 reviews)
If no matching entry is found, you should display an error message. For example:
FindByName BuffaloWings No such restaurant exists
-FindByCriteria RestaurantType RestaurantCity
This command searches and prints summary records of all restaurants in a specific city,RestaurantCity, and of a specific type, RestaurantType. For example, if RestaurantCity isSanDiego and RestaurantType is italian, you should display the following restaurants:
FindByCriteria italian SanDiego LaPizzeria 4356NobelDr, SanDiego CA 92130 www.pizerria.com rating: 4.40 (5 reviews)
Civico 8765RegentsRd, SanDiego CA 92101 www.civico.com rating: 5.00 (9 reviews)
If no matching entry is found, you should display an error message. For example:
FindByCriteria Chinese Carlsbad No such restaurant exists
- RemoveListing RestaurantName
This command removes the entry of a specific restaurant whose name is RestaurantName from the restaurants vector. You should check if that restaurant is listed before trying to delete its entry. Pay attention that deleting could be from any entry in the vector and not necessarily the last record. If no matching entry is found, you should display an error message. For example:
RemoveListing BuffaloWings No such restaurant exists
- Rate RestaurantName ReviewerRating
This command updates the rating score and number of reviewers of a specific restaurant, whose name is RestaurantName. Every time the Rate command is executed, the number of reviewers is incremented by one and the rate is calculated as the new moving average. In other words, it
is the sum of all past ratings and current one, ReviewerRating, divided by the total number of reviewers. Rating should be a positive number between 1 and 5. You should search for the restaurant whose name matches RestaurantName. If that restaurant exists and ReviewerRatingis valid, you should update the restaurants rating and number of reviewers. Otherwise, you should display an error message. For example:
Rate Agave 0 Rating should be a number from 1 to 5
- FindTopRated RestaurantCity This command prints summary information of the top rated restaurant(s) in a specific city
RestaurantCity. That is the restaurant(s) with the highest rating in the city. For example:
FindTopRated SanDiego Civico, italian 8765RegentsRd, SanDiego CA 92101 www.civico.com rating: 5.00 (9 reviews)
- To test your code, you may create and process the following transaction file, RestaurantsTrans.txt:
AddListing LaPizzeria 4356NobelDr SanDiego CA 92130 italian www.pizerria.com
AddListing Civico 8765RegentsRd SanDiego CA 92101 italian www.civico.com
AddListing OnBorder 101DoveSt SanDiego CA 92129 mexican www.border.com
AddListing Ortega 3465RegentsRd SanDiego CA 92130 mexican www.ortegarestaurant.com
AddListing ThePatio 6GenesseeRd SanDiego CA 92129 american www.thepatio.com
AddListing Season23 908BrooksAve SanDiego CA 92101 american www.season23.com
AddListing Cucina 1245ViaLaGitano Poway CA 93064 italian www.lacucina.com
AddListing ElCantina 10876PowayRd Poway CA 93064 mexican www.cantina.com
AddListing Joes 125BridleRd Poway CA 93064 american www.joes.com
AddListing OlivioRistorante 4320CaminoDr Carlsbad CA 92008 italian www.olivio.com
AddListing MariaRistorante 6534SpringburstDr Carlsbad CA 92008 italian www.mariaristorante.com
AddListing Agave 8764CreekViewBlvd Carlsbad CA 92009 mexican www.agave.com
AddListing Barrio 5Broadway Carlsbad CA 92010 mexican www.barrio.com
AddListing Seaside 5432DelSur Carlsbad CA 92009 american www.seaside.com AddListing TheHarbor 324CaminoSouth Carlsbad CA 92009 american www.theharbor.com
DisplayAll Rate ElCentro 5 Rate LaPizzeria 4 Rate LaPizzeria 4.5 Rate LaPizzeria 4.5 Rate LaPizzeria 5 Rate LaPizzeria 4 Rate Civico 5 Rate Civico 5 Rate Civico 5 Rate Civico 5 Rate Civico 5 Rate Civico 5 Rate Civico 5 Rate Civico 5 Rate Civico 5 Rate Cucina 3 Rate Cucina 3 Rate Cucina 3.5 Rate Cucina 3.5 Rate MariaRistorante 2 Rate MariaRistorante 3 Rate MariaRistorante 4 Rate OlivioRistorante 4 Rate OlivioRistorante 3 Rate OlivioRistorante 4 Rate OlivioRistorante 4.5
Rate OlivioRistorante 5 Rate OlivioRistorante 4 Rate OnBorder 5 Rate OnBorder 4.5
Rate Ortega 3 Rate ElCantina 4 Rate ElCantina 5 Rate Barrio 2 Rate ThePatio 4 Rate ThePatio 5 Rate ThePatio 4 Rate ThePatio 3 Rate ThePatio 4 Rate ThePatio 4 Rate ThePatio 5 Rate ThePatio 5 Rate ThePatio 5 Rate ThePatio 3 Rate ThePatio 4 Rate ThePatio 5 Rate Joes 2 Rate Joes 3 Rate Season23 4 Rate TheHarbor 5 Rate TheHarbor 4 Rate TheHarbor 4 Rate TheHarbor 4 Rate TheHarbor 5 Rate TheHarbor 6 Rate Agave 1 Rate Agave 0 Rate Agave 4 RemoveListing Barrio RemoveListing Seaside RemoveListing BuffaloWings DisplayAll FindByName BuffaloWings FindByName Agave FindByName Ortega FindByName Ristorante FindByCriteria italian SanDiego FindByCriteria american Carlsbad FindByCriteria chinese Carlsbad FindTopRated Poway FindTopRated SanDiego FindTopRated Escondido
Step by Step Solution
There are 3 Steps involved in it
Step: 1
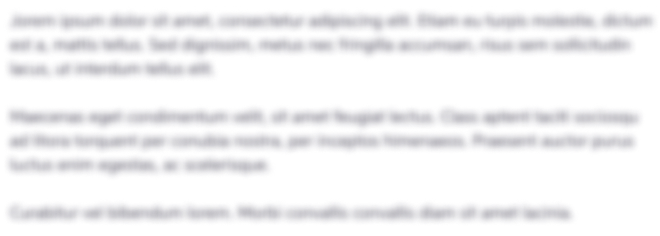
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started