Question
In c++ please. I need help implementing these three functions. enum class MagicNumber { kRawbits, kAscii, kFail }; struct Pixel { int red; int green;
In c++ please. I need help implementing these three functions.
enum class MagicNumber { kRawbits, kAscii, kFail };
struct Pixel {
int red;
int green;
int blue;
};
struct Image {
MagicNumber magic; // PPM format
std::vector comments; // All comments
int width; // image width
int height; // image height
int max_color; // maximum color value
Pixel **pixels; // Acts as 2D array of pixels
};
Part 1: Read the magic number and return enum
MagicNumber ReadMagic(std::ifstream &fppm);
PPM has multiple variants. The first two bytes of the file are called the magic number, and they tell us how to read the rest of the file.
The header file ppm.h defines a MagicNumber enum class that contains three entries.
kRawbits indicates that the PPM file is using a binary format. This is described in the Simple Image Formats reading. This corresponds to magic number P6. kAscii indicates that the PPM file is using the ASCII text format. This is described at ppm. Part 2 of this lab allows for some assumptions to make parsing easier. kFail indicates an unknown file type. Implement the ReadMagic function in ppm.cpp.
Do not assume that the file pointer is at the beginning of the file. Use seekg to seek to the beginning of the file. Read the first character by using read to read 1 char. Read the second character with read. Return the correct enum value based on the characters that you read. You can test this function using sled_dog.ppm which is in the rawbits format, and sled_dog_ascii.ppm which is in the text format. You can test failure by attempting to read any other file that is not a PPM image.
Part 2: Parse a PPM in text mode
Image ParseAsciiImage(std::ifstream &fppm);
In text mode, the pixel RGB values are written in ASCII text, creating a human-readable format.
There are a few assumptions you can make to simplify this process.
Comments in the PPM file are assumed to only start at the beginning of the line. Comments only exist after the magic number and before the width and height of the image. They cannot be put in the image data section. If your program receives a malformed PPM of any type, that is malformed for any reason (including violations of the assumptions above), then it should exit gracefully with an error message. Your program should not crash for any reason. You program should not leak memory for any reason.
Read the magic number again inside the parsing function. This serves two purposes: to advance the file cursor, and to verify that the correct function was called. Create a new Image struct. Read any comments that are present in the file. Comments are lines that begin with the # character. Append these comments to the comments field of the Image struct. Heap allocate (new) enough memory for the pixels, relying on the width and height of the image as defined in the file. Sanity check these values against some maximum bound. Feel free to reject images that are too large and would result in allocating an unreasonable amount of memory. Use a nested for loop to read three lines at time and create a Pixel struct. Copy this Pixel struct into the 2D pixels array of the image. Return the final image from the function. Part 3: Implement FreeImage
void FreeImage(const Image &img); Our image structures contain heap-allocated memory to store the pixels. Implement the FreeImage function in such a way that it frees this memory.
A part of the ppm file
P3 # Created by ... 630 458 255 119 119 131 126 126 138 131 131 141
Step by Step Solution
There are 3 Steps involved in it
Step: 1
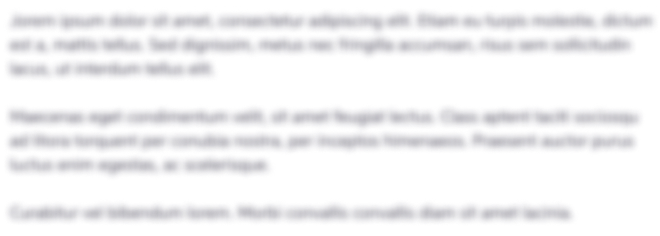
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started