Question
In C++ please, using a header and cpp file. For this assignment, you will complete the Turing machine you began to develop in major assignment
In C++ please, using a header and cpp file. For this assignment, you will complete the Turing machine you began to develop in major assignment 2. In that assignment, you developed code to represent the memory of the Turing Machine (the infinite tape) and the read head (the thing that selects the current square). Now you will implement the state table, and provide functions that allow your Turing machine to actually run. When finished your program will be as precisely as functional as the online turing machine simulator, although its user interface will be somewhat more primitive. (Links to an external site.)
Here's what you need to do:
- Extend your previous program to include a new constructor: TuringMachine(string tapeFile, string stateFile). This constructor specifies the names of two files, one specifying the initial state of the tape, the other specifying the turing machine states.
- You will need to use some sort of data structure (a vector, an array, whatever) to store the states of the machine. I leave it to you to determine what this data structure should be.
- The format of the tape file is as follows:
2:-3,1,3
- There is exactly one number before :. This number specifies the initial position of the tape head (the initial value of currentSquare)
- The numbers after the : represent the currently marked square. In the line above, you should consider the squares -3, 1, and 3 as containing 1s, and everything else as containing 0s
- I recommend using the getline() function (discussed in lecture on day 10) for this.
- The format of the state file is as follows:
1,R,2:1,L,2 1,L,1:0,L,3 1,L,0:1,L,4 1,R,4:0,R,1
The state file above specifies the Turing machine pictured below. Each line represents one state. The portions before the colon on each line represent the command to be executed if the current square is unmarked. The portions after the colon represent the command to be executed if the current square is marked. The three components of a command indicate:
* Whether the current square should be marked (0 for no mark, 1 for mark) * The direction the tape head should move (L for left, R for right) * The state that should be active the next time the machine updates
0 1
1 1R2 1L2
2 1L1 0L3
3 1L0 1L4
4 1R4 0R1
Here is what the program above looks like. When run, this program leaves six marks on the tape, at positions -1, 0, 1, 2, 3, 4
- Include a function in your TuringMachine called update(). This function executes the next instruction. For example, if we were using the program above, if the current square were 0 and the current state were 1, the update function would make a mark, move the head to the right, and then set the current state to 2.
- (notice that to implement this, you will need to include an instance variable in your TuringMachine to keep track of the current state)
- Include a function in your TuringMachine called run(). This function repeatedly calls update until the current state is 0.
I recommend testing your machine with the program given above. If your machine produces the following output:
Tape: [-1, 0, 1, 2, 3, 4] Current square: 0
Then your program is likely correct.
The details of how to implement your machine are up to you. My requirements are that you have the following functions, and that they all work appropriately:
A constructor that initializes your machine from two files: TuringMachine::TuringMachine(string tapeFile, string stateFile)
A function that makes your machine advance one step: void TuringMachine::update() A function that makes your machine run until it is set to state 0: void TuringMachine::run()
Currently, the code I have is as follows:
[TuringMachine.h]
#ifndef TURINGMACHINE_H
#define TURINGMACHINE_H
#include
using namespace std;
class TuringMachine {
public:
// Mutators
void moveLeft();
void moveRight();
void makeMark(bool mark);
// Accessors
bool readSquare();
void printMachineInfo();
// Default constructor with no arguments
TuringMachine();
private:
vector turingTape;
int currSquare;
};
#endif
[TuringMachine.cpp]
#include
#include "TuringMachine.h"
#include
#include
using namespace std;
TuringMachine::TuringMachine() { // Constructor that sets the current square position to 0
currSquare = 0;
}
void TuringMachine::moveLeft() { // Moves the current square position to the left
currSquare = currSquare - 1;
}
void TuringMachine::moveRight() { // Moves the current square position to the right
currSquare = currSquare + 1;
}
void TuringMachine::makeMark(bool mark) { // Changes the currently selected square to either 0 or 1, based on whether the bool passed in as a parameter is false (0) or true (1)
if (mark) {
if (!readSquare()) // When readSquare() returns false
turingTape.push_back(currSquare);
}
else { // When readSquare() returns true
if (readSquare()) {
for (int i = 0; i < turingTape.size(); i++) {
if (currSquare == turingTape.at(i)) {
turingTape.erase(turingTape.begin() + i);
break;
}
}
}
}
}
bool TuringMachine::readSquare() { // Indicates the current state of the square
for (int i = 0; i < turingTape.size(); i++) {
if (currSquare == turingTape.at(i))
return true;
}
return false;
}
void TuringMachine::printMachineInfo() { // Prints out the whole tape (indexes) and current square position
sort(turingTape.begin(), turingTape.end());
cout << "turingTape: [";
int size = turingTape.size();
for (int i = 0; i < size; i++) {
if (i != size - 1)
cout << turingTape.at(i) << ", ";
else
cout << turingTape.at(i);
}
cout << "]";
cout << " Current Square: " << currSquare << endl;
}
int main () { // Driver program to display expected format
TuringMachine t1;
t1.makeMark(true);
t1.moveRight();
t1.makeMark(true);
t1.moveRight();
t1.makeMark(true);
t1.moveLeft();
t1.moveLeft();
t1.moveLeft();
t1.makeMark(true);
t1.moveLeft();
t1.makeMark(true);
t1.moveLeft();
t1.makeMark(true);
t1.moveRight();
t1.moveRight();
t1.printMachineInfo();
return 0;
}
Output:
Turing Tape: [-3, -2, -1, 0, 1, 2] Current Square: -1
Help would be much appreciated, thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
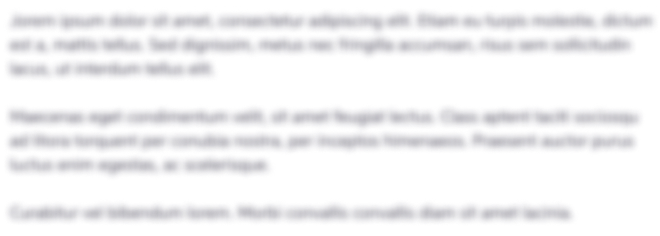
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started