Question
in c++ program Short.h Make the following deletions, changes and additions to Short.h : #ifndef _SHORT_H_DEF_ //replace with your initials #define _SHORT_H_DEF_ #include using namespace
in c++ program
Short.h
Make the following deletions, changes and additions to Short.h:
#ifndef _SHORT_H_DEF_ //replace with your initials
#define _SHORT_H_DEF_
#include
using namespace std;
enum display_type {DEC, BIN, HEX, ERROR};
class Short
// Unary operators
Short operator++ (int); //post
Short operator-- (int); //post
Short& operator++ (); //pre
Short& operator-- (); //pre
Short operator- () const;
Short operator+ () const;
// Just for fun, do this recursively
Short pow( unsigned exp ) const; //returns (*this)exp
void set_display_type( display_type );
};
#endif
this the full question
Please note, you are required to include the following when you use g++ to compile: -pedantic
For example:
g++ -pedantic filename.cpp
This will give a warning if you attempt to use any non-standard feature in g++. You must correct your code so that you do not receive any warnings of this type.
Log on to the Linux server.
Use four files as in the previous assignment:
Short.h
Short.cpp
ShortMain.cpp
Makefile
Make the following deletions, changes and additions to Short.h:
#ifndef _SHORT_H_DEF_ //replace with your initials
#define _SHORT_H_DEF_
#include
using namespace std;
enum display_type {DEC, BIN, HEX, ERROR};
class Short
{
signed char _n;
display_type _dt;
void _display_BIN( ostream& )const;
void _display_HEX( ostream& )const;
//other private member functions as needed
public:
Short( ); // _n == 0 && _dt == DEC
Short( signed char, display_type = DEC );
Short( const char* );
// char* must be well-formed (see definition below)
// if not well-formed: _n == 0 && _dt == DEC
Short ( const Short& );
// Immediate operators
Short& operator= (const Short&);
Short& operator+= (const Short&);
Short& operator-= (const Short&);
Short& operator*= (const Short&);
Short& operator/= (const Short&);
Short& operator%= (const Short&);
// I/O functions
friend ostream& operator<< (ostream&, const Short&);
// Writes the number in either decimal, binary, or hexadecimal form
// This is determined by the value in _dt
// Do not include a gratuitous newline
friend istream& operator>> (istream&, Short&);
// operator>> must read what operator<< writes
// It must be able to read DEC, BIN, or HEX
// Arithmetic operators
Short operator+ (const Short&) const; //[1]
Short operator+ (signed char) const;
friend Short operator+ (signed char, const Short&);
Short operator- (const Short&) const;
Short operator- (signed char) const;
friend Short operator- (signed char, const Short&);
Short operator* (const Short&) const;
Short operator* (signed char) const;
friend Short operator* (signed char, const Short&);
Short operator/ (const Short&) const;
Short operator/ (signed char) const;
friend Short operator/ (signed char, const Short&);
Short operator% (const Short&) const;
Short operator% (signed char) const;
friend Short operator% (signed char, const Short&);
// Comparison operators
bool operator== (const Short&) const;
bool operator== (signed char) const;
friend bool operator== (signed char, const Short&);
bool operator!= (const Short&) const;
bool operator!= (signed char) const;
friend bool operator!= (signed char, const Short&);
bool operator> (const Short&) const;
bool operator> (signed char) const;
friend bool operator> (signed char, const Short&);
bool operator< (const Short&) const;
bool operator< (signed char) const;
friend bool operator< (signed char, const Short&);
bool operator>= (const Short&) const;
bool operator>= (signed char) const;
friend bool operator>= (char, const Short&);
bool operator<= (const Short&) const;
bool operator<= (signed char) const;
friend bool operator<= (signed char, const Short&);
// Unary operators
Short operator++ (int); //post
Short operator-- (int); //post
Short& operator++ (); //pre
Short& operator-- (); //pre
Short operator- () const;
Short operator+ () const;
// Just for fun, do this recursively
Short pow( unsigned exp ) const; //returns (*this)exp
void set_display_type( display_type );
};
#endif
Well-formed For this assignment, we will define a string of characters to be well-formed if it satisfies one of the following requirements:
Contains three, two, or one base-ten symbols[2] and nothing else
Meets the first requirement and begins with either the minus sign- or the plus sign +
Contains two base-16[3] symbols followed by h and nothing else
Contains four base-two symbols[4], followed by a space character, followed by four more base-two symbols, followed by b and nothing else.
Note: Overflow can lead to interesting results. For example, if you use either the number 128 or the character string 128, your Short should contain the value -128. This is not an error in your code; it is the result of overflow.
In Short.cpp, implement these member functions and the following helper function:
//determines and returns the type of a char*, either DEC, BIN, HEX. It returns ERROR if the char* is not well-formed
display_type type ( const char* );
Write other helper-functions as needed. I have several. Place the prototypes for helper functions at the top of your Short.cpp file and the implementations at the bottom of the file. These are not member functions and should not be in your Short.h file.
You must not use any built-in functions
In ShortMain.cpp, write a test main to test your code. Remember: code that does not compile will not be graded and will receive a grade of ZERO. You must thoroughly test ALL of your code to ensure that it compiles and works correctly.
Submit these four files via the Canvas drop-box (append .txt to change Makefile to Makefile.txt)
[1] Implement all of the arithmetic operators in terms on the immediate operators. For example, use operator+= to implement operator+.
[2] Base-ten symbols: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
[3] Base-16 symbols: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E, F
[4] Base-two symbols: 0, 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
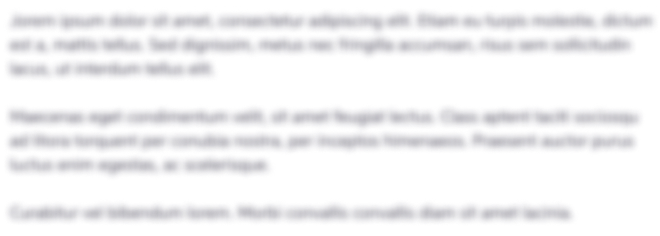
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started