Question
In C Programming: // This function creates an empty list pointer and returns it. List* createList ( ); //This function adds an integer item to
In C Programming:
// This function creates an empty list pointer and returns it. List* createList ( ); //This function adds an integer item to the end of the list by taking a list pointer and an integer item to be added and returns the updated list pointer. List* addItemAtEnd (List*, int); //This function adds an integer item to the beginning of the list by taking a list pointer and an integer item to be added and returns the updated list pointer. List* addItemInBeginning (List*, int); //This function prints out the items in the list passed through the parameter. void printList (List);
Structure given in header file:
#include
#include
typedef struct nodestruct {
int item;
struct nodestruct *next;
} Node;
typedef struct {
int size; // Number of items on users list
Node *head, *tail;
} List;
List* createList ();
List* addItemAtEnd (List*, int);
List* addItemInBeginning (List*, int);
void printList (List);
Node* createNode(int);
In main: First, create a List ADT based on the structure given in the header file. Then, 'generate' 13 random integer numbers between 1 and 13. Print out the 'generated' numbers on the console separated by space. Next, add first 6 of the 13 numbers one by one to the beginning of the list and the remaining 7 numbers one by one to the end of the list. Print out the list on the console. Finally, terminate the program. Example output: mwc-070138:~ $ gcc main.c lab5.c -Wall -Werror mwc-070138:~ $ ./a.out Randomly 'generated' numbers: 1 1 2 4 3 5 13 10 3 11 2 6 5 Items on the linked list: 5 3 4 2 1 1 13 10 3 11 2 6 5 Have a nice day!!!!! mwc-070138:~ $
Step by Step Solution
There are 3 Steps involved in it
Step: 1
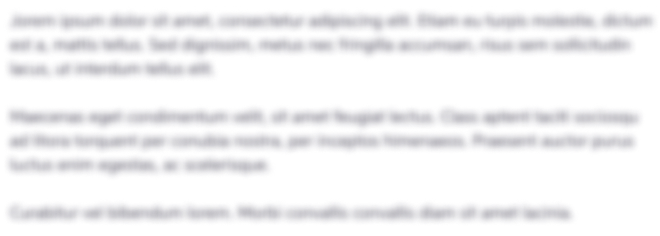
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started