Question
In C programming: This program involves implementing a simple Car Rental System that will allow the user to read, store, and manipulate customer data through
In C programming:
This program involves implementing a simple Car Rental System that will allow the user to read, store, and manipulate customer data through a friendly text-based interface. During execution, the program stores all customer data in a linked list, and upon termination this data is automatically saved into a text file.The function definitions in file "proj-part1.c" are basically empty. Your main task in this project is to fill in the missing code based on the following descriptions of what each function is supposed to do. Empty functions and .txt files shown (cars.txt, customers.txt)
#include
//This function should open and read the given input file which contains information about all rental cars. //It should store each car's data in an element of the given array arr, and return the number of cars it read from the file. //Notice that the file contains extra information which you should just skip/ignore (using * option in fscanf) //The general format of the input file should be obvious from the example file provided "final_project_input1_cars.txt" //Obviously the value of member is_available should be initialized to 1 for all elements of array arr.
int readCarsInfo(char *filename, CarInfo arr[]) { int n = 0;
// ...
return n; }
//This is similar to function readCarsInfo, except it reads customers' information instead of cars information. //It should return the number of customers it read from the input file. //The general format of the input file is indicated in the example file provided "final_project_input2_customers.txt"
int readCustomersInfo(char *filename, CustInfo arr[]) { int n = 0;
// ...
return n; }
//This function should return a pointer to the element in array arr that contains the car whose //identification number is equal to the given value id
CarInfo * findCar(CarInfo arr[], int size, unsigned int id) { CarInfo *p = NULL;
// ...
return p;
}
//This function should return a pointer to the element in array arr that contains the customer whose social security //number is equal to the given value ssn (hint: you should use strncmp with n=4)
CustInfo * findCustomer(CustInfo arr[], int size, char *ssn) { CustInfo *p = NULL;
// ...
return p; }
//This function should first create a new node (dynamic variable) of type RentalInfo that contains rental information //for the given car and the given customer and then insert this node at the beginning of given linked list. // //Guidelines for implementation: // - use the input argument id to find the corresponding car in the cars array arr1 by calling function findCar() // - use the input argument ssn to find the corresponding customer in the customers array arr2 by calling function findCustomer() // - the value of member out_date of the new node should be set by using the standard library time.h to determine the current date and hour. // - the value of member num_days of the new node should be equal to the input argument days // - the value of member is_available of the corresponding car to 0
RentalInfo * rent_a_car(RentalInfo *list, CarInfo arr1[], int size1, CustInfo arr2[], int size2, unsigned int id, char *ssn, unsigned int days) {
// ...
return list; }
//This function display on the screen the information in all elements of the given linked list. //If the list is actually empty, the function should display a simple message such as "No rented cars." //The output should be formatted such that it is elegant and easy to read.
void displayRentals(RentalInfo *list) {
// ...
}
(cars.txt)
Car ID Car Make Car Model Year Unit Price Per Day MPG ---------------------------------------------------------------------------------------------------------------------------------------------------------- 311 Chevrolet Chevelle-Malibu 1 2014 45.99 21 312 Buick Skylark-320 2014 79.95 19 313 Plymouth Satellite 2017 61.75 N/A 314 AMC Rebel-SST 2016 99.99 16 315 Ford Torino 2015 85.99 22.1 316 Ford Galaxie-500 2016 61.95 29.5 317 Chevrolet Impala 2013 42.99 18.7 318 Plymouth Fury-iii 2017 69.85 20.52 319 Pontiac Catalina 2012 29.99 N/A 320 AMC Ambassador-DPL 2015 95.99 16.30 321 Citroen DS-21-Pallas 2016 59.75 19.05
(customers.txt)
SSN First Name Last Name ---------------------------------------------------------------- xxx-xx-3192 Farhad Akbar xxx-xx-0721 Bassam Awad Alanazi xxx-xx-9210 Roger Cungtha Biak xxx-xx-7226 Christian C. Collier xxx-xx-0802 Tanner Durnil xxx-xx-1155 Melissa Gudeman xxx-xx-7530 Daniel Patrick Kobold Jr xxx-xx-8162 Travis Lee Leondis xxx-xx-0011 Cleopatrio Soares Neto xxx-xx-5533 Daniel Biaklian Sang xxx-xx-1100 Bryant Bernard xxx-xx-8123 Joaquin John Garcia xxx-xx-3128 Jacob Austin Shebesh xxx-xx-9011 D'Artagnan S. Engle xxx-xx-1234 You Zhou xxx-xx-4321 Joi Ashlyn Officer
Step by Step Solution
There are 3 Steps involved in it
Step: 1
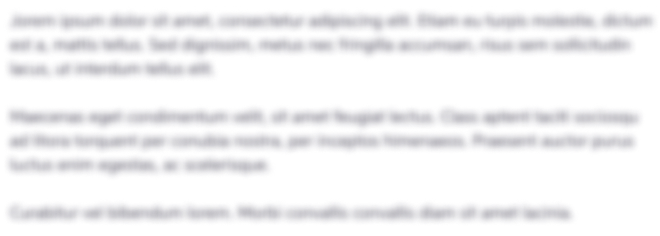
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started