Question
In C++ Requirement 1: You are required to create a dynamic array and display all elements of array. The array size will be given by
In C++
Requirement 1: You are required to create a dynamic array and display all elements of array.
The array size will be given by reading argv[1].You are allowed to use ARRAY Syntax (e.g. int
arr[], accessing elements by index using arr[i]) to create an array.
After creating the array, you need to assign value into an array through keyboard (using cin).
You are allowed to use standard ARRAY Syntax (e.g. int arr[]) to assign a value to each element
in the array.
Finally, you MUST use a pointer to print out all elements in the array (using cout). Each of
elements should be separated by a space. And the last element will have to end up with end line
character (endl). You will receive 0 points if you use standard array syntax.
Requirment 2: You are required to create a dynamic array and display all elements of array.
The array size will be given by reading argv[2].You are NOT allowed to use ARRAY Syntax to create an array. You MUST use pointer(s) to create the dynamic array.
After creating the array, you need to fill up the array through keyboard (using cin). You are NOT allowed to use standard ARRAY Syntax to assign value to each element. You MUST use a pointer to do.
To print out all elements in the array on screen (using cout), you MUST use a pointer. When printing, each of elements should be separated by a single space, and there should be an endl following the last element. Finally, you MUST delete your dynamic array/clear your allocated memory after printing out the entire of array.
Requierment 3: You will use both pointers and class. Using the included class Car, you are required to
create a dynamic array of car objects and display attributes of each object. (45 Points)
The array size will be initialized by reading argv[3].
To fill up the attributes of each car object in the array, you should prompt the user to enter each
attribute separately (model, color, mileage).
You can use cout to display prompt and use cin to read values from the user.
You can use the overloaded constructor to set custom attributes of each object of type Car at
time of creation.
After filling out the entire car objects, you need to print out all three attributes for each car
object in the array (using cout). Three attributes for each object MUST be separated by space.
And each object MUST be printed on its own line.
You should print the attributes in the following order: Model Color Mileage. If your output
doesn't match this, you will get 0 credit.
Finally, you MUST delete your dynamic array when done. And again, in this problem, you are NOT allowed to use standard ARRAY Syntax. You MUST use Pointer.
You will be reading your arguments from the command line. The size of the dynamic array in the Requierment 1 will be passed into your program as argument 1, argv[1]. The size of the dynamic array in
the Requierment 2 will be passed into your program as argument 2, argv[2]. The size of the dynamic array in the Requierment 3 will be passed into your program as argument 3, argv[3].
Here is the main file structure that you MUST use. You may NOT add or remove, or change the parameters or variables.
#include#include "Car.h" using namespace std;
// pointers void problemOne(int arraySize) {
int numbers[arraySize]; for (int i = 0; i < arraySize; ++i) {
cout << "Enter next number" << endl;
cin >> numbers[i]; }
int *p = numbers; // Print out the array 'numbers' by using the pointer p // YOU MAY NOT ACCESS ELEMENTS USING THE 'numbers[i]' STYLE SYNTAX!!! // using that method will result in a ZERO!!! /*
* Your code goes here
*/ }
// Dynamic Arrays - void problemTwo(int arraySize) {
cout << "Problem 2" << endl; int* numbers = new int[arraySize];
// 1. Fill array from cin (WITHOUT using standard array syntax! You must // use the pointer). // 2. Print the newly filled array with cout // 3. Delete the pointer 'numbers' with the 'delete' keyword.
// Remember, using the standard array syntax will get you no credit. /*
* Your code goes here
*/ }
// Dynamic array, pointer, and struct void problemThree(int arraySize) {
// 1. Using the included class "Car", create a dynamic array using the // parameter arraySize. // 2. Fill it with cars that you create by accepting // user input from the keyboard (You should prompt the user to enter each // attribute separately - model, color, mileage).
// 3. Use those values to call the overloaded constructor and fill the // array dynamically. // // Hint: Use another pointer to move through the array.
// // 4. After you fill the array, print out all three attributes for each car. // 5. Finally, delete your dynamic array. // Again, you may NOT use the standard array syntax, or you will receive // a 0 for this section.
} int main(int argc, char* argv[]) {
problemOne(atoi(argv[1])); problemTwo(atoi(argv[2])); problemThree(atoi(argv[3])); return 0;
}
We will provide the class Car as shown below. You are NOT allowed to modify it. Car.h:
#ifndef CAR_H #define CAR_H
#includeusing namespace std;
class Car { int mileage;
public: string model; string color;
Car(); Car(string, string, int);
int getMileage();
void setMileage(int); };
#endif //CAR_H
Car.cpp:
#include "Car.h"
Car::Car() { color = "tan";
model = "civic";
mileage = 100000; }
Car::Car(string model, string color, int mileage) { this->color = color; this->model = model; this->mileage = mileage;
}
int Car::getMileage() { return mileage;
}
void Car::setMileage(int newMileage) { mileage = newMileage;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
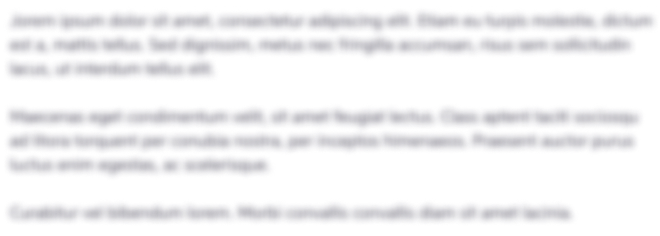
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started