Question
In C++ StackCalculator. Please complete the TO DOs in the porvided code below from the StackCalculator.cpp and driver.cpp files CODE TO COPY: ------------------------------------------------------------ StackCalculator.hpp -------------------------------------------------------------
In C++ StackCalculator. Please complete the TO DOs in the porvided code below from the StackCalculator.cpp and driver.cpp files
CODE TO COPY:
------------------------------------------------------------
StackCalculator.hpp -------------------------------------------------------------
#pragma once
#include
struct Operand
{
float number;
Operand* next;
};
class StackCalculator
{
private:
Operand* stackHead; // pointer to the top of the stack
public:
StackCalculator();
~StackCalculator();
bool isEmpty();
void push(float num);
void pop();
Operand* peek();
Operand* getStackHead() { return stackHead; }
bool evaluate(std::string* s, int size);
};
--------------------------------------------------------------
StackCalculator.cpp
--------------------------------------------------------------
#include
#include "StackCalculator.hpp"
using namespace std;
/*
* Purpose: Determine whether some user input string is a
* valid floating point number
* @param none
* @return true if the string s is a number
*/
bool isNumber(string s)
{
if(s.size() == 1 && s == "-")
return false;
else if(s.size() > 1 && s[0] == '-')
s = s.substr(1);
bool point = false;
for(int i = 0; i
if(!isdigit(s[i]) && s[i] != '.')
return false;
if(s[i]=='.' and !point) point = true;
else if(s[i]=='.' and point) return false;
}
return true;
}
StackCalculator::StackCalculator()
{
//TODO:
}
StackCalculator::~StackCalculator()
{
//TODO:
}
bool StackCalculator::isEmpty()
{
//TODO:
}
void StackCalculator::push(float number)
{
//TODO:
}
void StackCalculator::pop()
{
//TODO:
}
Operand* StackCalculator::peek()
{
//TODO:
return nullptr;// remove this line if you want
}
bool StackCalculator:: evaluate(string* s, int size)
{
/*TODO: 1.scan the array from the end
2.Use isNumber function to check if the input is an operand
3.push all operands to the stack
4.If operator take two element of from the stack,
compute and put the result back in stack
5.Handle the boundery cases as required.
6.Read the writeup for more details
*/
return true;
}
------------------------------------------------------------------------------ driver.cpp
------------------------------------------------------------------------------
#include
#include
#include
#include "StackCalculator.hpp"
using namespace std;
int main()
{
// stack to hold the operands
StackCalculator stack;
int numElement = 0;
string* inparr = new string[50];
// enter a number
string number;
cout
while(true)
{
cout ";
getline(cin, number);
/* TODO
1. Read input (operators and operands) until you encounter a "="
2. store them in inparr
*/
}
/* TODO - If the inparr is empty then print "No operands: Nothing to evaluate"
else call the evaluate function
*/
/* TODO - Validate the expression
1. If valid then print the result cout
2. Else, print "Invalid expression"*/
return 0;
}
OBJECTIVES 1. Create, add to delete from, and work with a stack implemented as a linked list 2. Create, add to delete from, and work with a queue implemented as an array Overview Stacks and Queues are both data structures that can be implemented using either an array or a linked list. You will gain practice with each of these in the following two mini-projects. The first is to build a simple calculator using a linked list stack and the second is to simulate a job scheduling system using a circular array based queue. Stack calculator In this assignment we will implement a linked list based stack. We will use this stack implementation to evaluate prefix expressions. In the following section we will discuss what is a prefix expression and how we can evaluate them using stack. Your task will be to implement the logic into c++ code. We will consider only two binary operators "+" and "" for prefix notations. Prefix Notation In general the way we express a binary operator in mathematical expression is infix. For example "4 + 3". In this expression the order is "
Step by Step Solution
There are 3 Steps involved in it
Step: 1
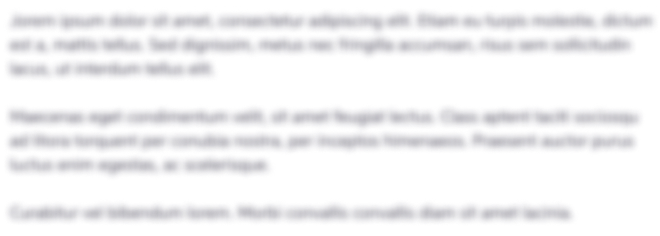
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started