Question
IN C++ Step 1: Write a class called Administrator which is to be derived from the class SalariedEmp which is derived from Employee. Use these
IN C++
Step 1: Write a class called Administrator which is to be derived from the class SalariedEmp which is derived from Employee.
Use these files:
employee.h
employee.cpp
salariedEmp.h
salariedEmp.cpp
empTest.cpp
Your Administrator class should have the following additional members:
A data member of type string that contains the administrator's title (such as Director or Vice President).
A data member of type string that contains the company area of responsibility (such as Production, Accounting, or Personnel).
A data member of type string that contains the name of this administrator's immediate supervisor.
A default constructor that initializes ALL of the data members to empty strings, as well as a constructor that allows the client programmer to provide values for ALL of the data members.
A member function called changeSupervisor which changes the supervisor name.
A member function for reading in the administrator's information from the keyboard. The user should be prompted for each piece of information. This should include information to fill in the data members derived from other classes, such as the name of the administrator.
A member function called print which outputs the administrator's data to the screen.
A redefinition of the member function printCheck() with appropriate notations on the check. This function will look a lot like the print member function, except that the output will look like a check. Base this output on the printCheck() function in the base class. The check itself will not change from how it looks in the base class. The check stub, however, should include information such as the administrator's title, area of responsibility, and supervisor.
// this is the file "employee.h" #ifndef EMPLOYEE_H #define EMPLOYEE_H #includeusing namespace std; class employee { public: employee(); employee(const string& newName, const string& newSsn); string getName() const; string getSsn() const; void changeName(const string& newName); void changeSsn(const string& newSsn); private: string name; string ssn; double netPay; }; #endif
// this is the file "employee.cpp" #include "employee.h" #includeusing namespace std; employee::employee() { netPay = 0; } employee::employee(const string& newName, const string& newSsn) { name = newName; ssn = newSsn; netPay = 0; } void employee::changeName(const string& newName) { name = newName; } void employee::changeSsn(const string& newSsn) { ssn = newSsn; } string employee::getName() const { return name; } string employee::getSsn() const { return ssn; }
// this is the file "salariedemp.h" #ifndef SALARIEDEMP_H #define SALARIEDEMP_H #include "employee.h" #includeusing namespace std; class salariedEmp : public employee { public: salariedEmp(); salariedEmp(const string& newName, const string& newSsn, double newWeeklySalary); void printCheck() const; void giveRaise(double amount); protected: double salary; }; #endif
// this is the file "salariedemp.cpp #include "salariedemp.h" #include "employee.h" #includeusing namespace std; void salariedEmp::giveRaise(double amount) { salary += amount; netPay += amount; } salariedEmp::salariedEmp() { salary = 0; netPay = 0; } salariedEmp::salariedEmp(const string& newName, const string& newSsn, double newWeeklySalary) : employee(newName, newSsn) { salary = newWeeklySalary; netPay = salary; } void salariedEmp::printCheck() const { cout << "pay " << name << endl; cout << "the sum of " << netPay << " Dollars." << endl; cout << "Check Stub: " << endl; cout << "Employee number: " << ssn << endl; cout << "This is a salaried employee. Regular pay: " << salary << endl; }
// this is the file emptest.cpp #include#include "hourlyemp.h" #include "salariedemp.h" using namespace std; int main() { hourlyEmp arnold("Arnonld Jones","23456664",13,20); arnold.setHours(10); cout << "Check for " << arnold.getName() << " for " << arnold.getHours() << " hours:" << endl; arnold.printCheck(); cout << endl << endl << endl; arnold.giveRaise(5); cout << "Check for " << arnold.getName() << " for " << arnold.getHours() << " hours:" << endl; arnold.printCheck(); cout << endl << endl << endl; salariedEmp sally("Sally Wu", "345123456", 1234.45); cout << "Check for " << sally.getName() << endl; sally.printCheck(); cout << endl << endl << endl; sally.giveRaise(10); cout << endl << endl << endl; cout << "after a raise of 10, Sally's check:" << endl; sally.printCheck(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
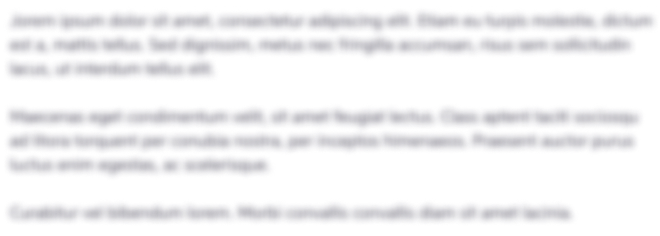
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started