Question
In C++ Using this code and functions go at the bottom: #include #include #include #include #include #include #include // PLACE CODE PROTOTYPES HERE FOR TASK
In C++ Using this code and functions go at the bottom:
#include
// PLACE CODE PROTOTYPES HERE FOR TASK A // *************************************************
class myString { public: myString(); // Default construction; the default value shall be the empty string.
myString(const char* ); //for initializing with a string //Pre: pointer must not be a null pointer //Post: will initialize an object to a string literal
myString(const char*, std::size_t); //initialization by part of a string literal //Pre: c-string must not be null and have size charAmount //Post: will initialize object to the charAmount of characters in a string literal
myString(const myString &); //copy constructor initializer //Pre: must accepts an object of the same type //Post: The initialized object will be equal to the original
myString(myString &&); //move constructor
bool empty() const; //empty function to tell if a string is empty or not //Pre: none //Post: returns 1 for true and 0 for false and does not change string (1 is empty, 0 is not empty)
std::size_t size() const; //size() function to return the length of a string //Pre: none //Post: return the lenght of a string not including the null terminator (does not change the string)
char* data() const; //returns a raw c-string pointer //Pre: none //Post: returns a char pointer that holds the c-string
std::size_t find(char ) const; //returns the index of the first occurrence of the target char //Pre: accepts a valid char for the target the user wants to find //Post: returns index where the target first occurs or npos if not found
char* substr(std::size_t, std::size_t) const; //makes a string out of an existing string //Pre: accepts a integer for the index to start at and charAmount for the amount of characters //Post: returns a char pointer for the new substring
friend std::ostream& operator(myString &) const; //overloading > comparison operator bool operator>(const char *); //overloading > comparison operator for string literal bool operator>=(myString &) const; //overloading >= comparison operator bool operator>=(const char *); //overloading >= comparison operator for string literal myString operator+(myString &) const; //overloading + operator myString& operator=(myString &&); //move assignment operator // add a test case for move construction and assignment and EARN B O N U S points!!!
~myString() //deconstructor { delete [] stringVar; stringVar = nullptr; }
// Defines the npos value. static constexpr std::size_t npos = -1; private: char* stringVar; short strLength; };
using std::cout; using std::cin; using std::endl; using std::setw; using std::left; using std::right;
int main() { // Check the default contsructor (ctor). { myString s; assert(s.empty()); } // A member function to return the length of the string. // Returns a std::size_t (unsigned integer) value. The size() function // shall not modify the object s1. // // This operation shall be constant time (i.e., you cannot traverse the // underlying string to compute the length). { myString s1 = "hola"; std::size_t n = s1.size(); }
// string ctor { char const* str = "hello"; myString s = str; assert(s.data() != str); assert(strcmp(s.data(), str) == 0); }
// Raw C-string access, which returns the underlying array of // characters as a pointer. This member function shall not modify its // object. { myString s1 = "hola"; char const* p1 = s1.data(); }
// copy ctor // Initialization by a string literal. The initialized object (s1) // shall be equal to the string literal after initialization. // The string literal shall not be a null pointer. You must assert // this property. // Copy construction. The initialized object (s2) shall be equal to // the original (s1) after initialization. { myString s1 = "hello"; myString s2 = s1; assert(strcmp(s1.data(), s2.data()) == 0); }
// Initialization by a bounded C-string. The initialized object (s2) // shall be equal to the first n characters of the given C-string. // The C-string shall not be null and shall have at least length n. // You must assert this property. Hint: use std::strnlen and be aware // that it may not insert a null-terminator. { myString s2("yolo", 2); // s2 is equal to "yo". assert(!strcmp(s2.data(), "yo")); }
// Copy assignment. After assignment, the assigned object on the left (s1) // shall be equal to the value on the right (s2). { myString s1 = "hello"; myString s2; s2 = s1; assert(strcmp(s1.data(), s2.data()) == 0); // Compound addition/assignment (concatenation). Appends the string s2 // to s1. s1 += s2; }
// Assignment to a string. After assignment, the object on the left (s1) // shall be equal to the string literal on the right. { myString s1; s1 = "hello"; assert(!strcmp(s1.data(), "hello")); } // self assign { myString s1 = "hello"; s1 = s1; }
// A member function to determine if a string is empty. Returns true // if s1 is the empty string. The empty() function shall not modify // the object s1 (i.e., the member function must be const). { myString const s1; assert(s1.empty()); myString const s2 = ""; assert(s2.empty()); }
// Character access. Support reading and writing of characters using // the subscript operator. Both operators take a std::size_t argument n, // such that n >= 0 and n
myString const s2 = "test"; assert(s2[0] == 't');
assert(s1[-1]); assert(s2[-1]); }
// A member function that returns the index of the first character // in the string. This shall return a std::size_t value. If no such // character exists, return npos. Hint: see std::strchr. // // Note that npos is already defined within your class. // // This function shall not modify its object. { myString const s1 = "abcdef"; assert(s1.find('c') == 2); assert(s1.find('z') == s1.npos); }
// A member function that creates a substring comprising all of the // characters starting at an index i and containing n characters. The // index i shall be a valid position in the string. You must assert // this condition. If n is larger than the number of characters past i // then all characters after i are copied to the the output. // Hint: use your bounded C-string constructor. // // This function shall not modify its object. { myString const s1 = "abcdef"; myString s2 = s1.substr(0, 3); myString s3 = s1.substr(3, 3); assert (s2 == "abc"); assert (s3 == "def"); }
// Equality comparisons. Two strings compare equal when they have the // same sequence of characters. Hint: see std::strcmp. { myString const s1 = "hello"; myString const s2 = "goodbye"; assert(s1 == s1); assert(s1 != s2); }
// ordering. One string compares less than another when it lexicographically // precedes it. Hint: see std::strcmp { myString s1 = "abc"; myString s2 = "def"; assert(s1 s1); assert(s1 = s1); }
// concatenation { myString s1 = "abc"; myString s2 = "def"; myString s3 = s1 + s2; myString s4 = "abcdef"; assert(s3 == s4); }
// self concatenation { myString s1 = "abc"; s1 += s1; myString s2 = "abcabc"; assert(s1 == s2); }
cout
// PLACE CODE HERE FOR TASK A // ************************************************* // TASK A CODE // the ostream overload is provided std::ostream& operator This is an inefficient string, but it will provide us with the practice at creating a dynamically allocated string, writing methods to support the string, and other goodies such as copy semantics. You should use as much from the C-String library as possible, and you should not have anything referencing the standard string - std::string. If there is then it will be difficult to get any points for this task, so be careful. We will write a class called myString. Implementing myString requires you to provide many, many functions, discovering the joy in operator overloading. You will find the defined myString class and all associated functions' declarations in myCodeDos cpp. Download it. Most of these functions and overloaded operators are relatively straightforward in the specifications. You will write all of those functions in the task A coding area. Review the code in the driver and the class definition before starting. You must make the test cases, which are also given to you, properly work as described in the comments. For task A you need to: Create the many supporting functions for the myString class Provide overloads as described Naturally, you will also be required to provide a destructor that releases or deletes any memory allocated to the string in a constructor or assignment operator Overloads of streaming operators ('
Step by Step Solution
There are 3 Steps involved in it
Step: 1
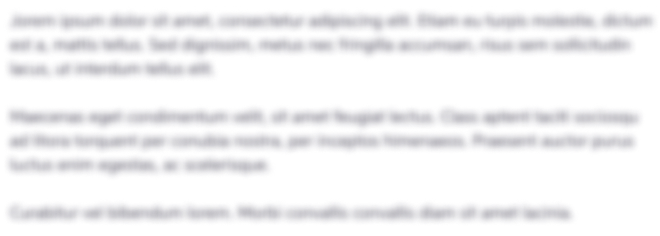
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started