Question
In C++, what is the Main.cpp for this program to run the tournament? Thank you. In this project, using C++, you will write a program
In C++, what is the Main.cpp for this program to run the tournament? Thank you.
In this project, using C++, you will write a program to run a tournament. Start with creature header and inplementation files listed below. This is a one-user-two-players game. A user will enter the number of fighters both players will use. The user should also enter the type of creatures and fighter names. The first player supplies a lineup of creatures in order. The second player then supplies his lineup of creatures in order. By lineup I mean something like a batting order in baseball or softball. The winner gets put at the back of her/his teams lineup; the loser goes to the container for those who lost. The lineup order cannot be changed once the players are entered. Similarly, the loser pile should have the last loser at the top and the first loser at the bottom. Even if a creature wins, she/he may have taken damage. You should restore some percentage of the damage when they get back in line. Make this simple as you are adding a new member function to the parent class. Some examples are: generate a random integer in some ranges, e.g., on a roll of 5 they recover 50% of the damage they lost, or some scheme of your own. If you want to use a different recovery function for each character, that is fine. Remember to use polymorphism if the recovery is different for different creatures! After each round, you will display which type of creatures fought and which won on screen (For example, Round 1: Team A Blue man No.1 VS. Team B Harry Potter No.1, Harry Potter No.1 won!). At the end of the tournament (when one team or both run out of fighters in the lineup), your program will display final total points for each team (you can determine how to score each round of fight, for example, winner team +2, loser team -1, tie +1). You must display which team won the tournament. This is another element you must define in the analysis and design stage. You must have a method to determine the winner and you can determine it by yourself. To help you testing and that of the graders, provide an option to display the winner and the updated scores for each round. Also provide an option at the end of the tournament to display the contents of the loser pile, i.e. print them out in order with the loser of the first round displayed last. You should be using polymorphism and all creatures will inherit from the Creature class. Each object should be instantiated and put into their lineup. You will only use pointers to creatures in your program. A creature pointer can then be used to indicate an object of a subclass and polymorphism will ensure the correct function is called. NOTE: Depending upon your implementation, you could have ties. How does that tie change your end of match logic? What do you do if the final match is a tie? Specifically, where do you put the fighters? For this program, you should use stack-like or queue-like structures to hold the lineups and the loser pile. Which will you use for the lineup? Which will you use for the loser pile? This is a programming assignment and not a complete game! Other than entering the lineup, the players just wait for the results. They should take no further actions. Make sure you test your program with instances of all creature types youve created. You may not be able to do an exhaustive test, but make it as extensive as you can.
Here are header and inplementation files. Ask if you need other files. These are files started and used for another program.
barbarian.cpp
#include
using namespace std;
void Barbarian::setData(){ fightName = "Barbarian"; fighterArmor = 0; strength = 12; death = false; }
int Barbarian::fightAttack(){ int damage = rollForAttack(); cout << "Barbarian attacked for a total of " << damage<< " damage." << endl; return damage; }
void Barbarian::defend(int d){ int defend = rollForDefense(); int damage; if(d != 999){ cout << "Barbarian defends for " << defend<< " damage." << endl; damage = d - defend; }else{ damage = d; }
if(damage <= 0){ cout << "Barbarian defended himself." << endl; cout << "Armor at: " << fighterArmor<< endl; cout << "Strength left: " << strength<< endl; }else if(damage = 999){ cout << "Barbarian has died. He failed to divert his eyes from Medusa." << endl; cout << "Armor at: 0" << endl; cout << "Strength left: 0" << endl; death = true; }else if(damage > fighterArmor){ damage = damage - fighterArmor; strength = strength - damage; fighterArmor = 0; if(strength > 0){ cout << "Barbarian wounded." << endl; cout << "Armor at: " << endl; cout << "Strength at: " << endl; }else if(strength <= 0){ cout << "Barbarian died. :(" << endl; cout << "Armor at: " << endl; cout << "Strength at: " << endl; death = true; //barbarian has died } } }
string Barbarian::getUserName(){ return fightName; }
bool Barbarian::checkDeath(){ return death; }
int Barbarian::rollForAttack(){ int diceRoll = rand()%6 + 1; //generate random number within 6 for die roll diceRoll = diceRoll + (rand()%6 +1); //2nd dice added to get full attack return diceRoll; }
int Barbarian::rollForDefense(){ int diceRoll = rand()%6 + 1; //generate ramdom number for defense diceRoll = diceRoll + (rand()%6 + 1); return diceRoll; }
int Barbarian::getFighterArmor(){ return fighterArmor; }
int Barbarian::getFighterStrength(){ return strength; }
barbarian.hpp
#ifndef BARBARIAN_HPP #define BARBARIAN_HPP
#include "creature.hpp"
using namespace std;
class Barbarian:public Creature{ protected:
public: void setData(); int fightAttack(); void defend(int d); string getUserName(); bool checkDeath(); int rollForAttack(); int rollForDefense(); int getFighterArmor(); int getFighterStrength(); ~Barbarian(){}; };
#endif
bluemen.cpp
#include
void BlueMen::setData(){ fightName = "Bleu Men"; fighterArmor = 3; strength = 12; death = false; lossDie = 0; //special ability }
int BlueMen::fightAttack(){ int damage = rollForAttack(); cout << "Blue Men attacked for " << damage << "damage." << endl; return damage; }
void BlueMen::defend(int d){ int defend = rollForDefense(); int damage; if(d != 999){ cout << "Blue Men defended for " << defend<< " points." << endl; damage = d - defend; }else{ damage = d;
if(damage <= 0){ cout << "Blue Men defened." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(damage > 0 && damage <= fighterArmor){ fighterArmor = fighterArmor - damage; cout << "Blue Men defended with minor damage." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(damage == 999){ cout << "Blue Men has turned to stone after looking at Medusa." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: 0" << endl; death = true; //Blue men died }else if(damage > fighterArmor){ damage = damage - fighterArmor; strength = strength - damage; fighterArmor = 0; if(strength > 0){ cout << "Blue men have been wounded."<< endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(strength <= 0){ cout << "Blue Men have died. :("<< endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; death = true; //blue men died } } if(strength <= 8 && strength > 4){//special ability for mob attack from blue men - every 4 pts of demage they lose one defensive roll lossDie = 1; //lose 1 die }else if(strength <= 4 && strength > 0){ lossDie = 2; //lose 2 die } } }
string BlueMen::getUserName(){ return fightName; }
bool BlueMen::checkDeath(){ return death; }
int BlueMen::rollForAttack(){ int diceRoll = rand()%10 + 1; //generates a number within in 10 diceRoll = diceRoll + (rand()%10 + 1); //2nd dice roll added to first return diceRoll; }
int BlueMen::rollForDefense(){ int diceRoll;
if(lossDie == 0){ diceRoll = rand()%6 + 1; //defensive roll within 6 diceRoll = diceRoll + (rand()%6 + 1); diceRoll = diceRoll + (rand()%6 + 1); }else if(lossDie == 1){ diceRoll = rand()%6 + 1; diceRoll = diceRoll + (rand()%6 + 1); }else if(lossDie == 2){ diceRoll = rand()%6 + 1; }
return diceRoll; }
int BlueMen::getFighterArmor(){ return fighterArmor; }
int BlueMen::getFighterStrength(){ return strength; }
bluemen.hpp
#ifndef BLUEMEN_HPP #define BLUEMEN_HPP
#include "creature.hpp" #include
using namespace std;
class BlueMen:public Creature{ protected: int lossDie; public: void setData(); int fightAttack(); void defend(int d); string getUserName(); bool checkDeath(); int rollForAttack(); int rollForDefense(); int getFighterArmor(); int getFighterStrength(); ~BlueMen(){} };
#endif
harrypotter.cpp
#include
void HarryPotter::setData(){ fightName = "Harry Potter"; fighterArmor = 0; strength = 10; death = false; hogwarts = 1; //special ability for HP }
int HarryPotter::fightAttack(){ int damage = rollForAttack(); //calling attack function cout << "Harry Potter attacks and generated " << damage<< " damage points." << endl; return damage; }
void HarryPotter::defend(int d){ int defend = rollForDefense(); //calling defense function int damage; if(d != 999){ cout << "Harry Potter defended for " << defend << " defense points." << endl; damage = d - defend; }else{ damage = d; }
if(damage <= 0){ cout << "Harry Potter defended himself." << endl; cout << "Armor points: " << fighterArmor<< endl; cout << "Strength points: " << strength<< endl; }else if(damage = 999 && hogwarts == 1){//special ability cout << "Harry Potter died by looking at Medusa"<< endl; cout << "*At Hogwarts* and Harry Potter has recovered with 20 strength points." << endl; hogwarts = 0; strength = 20; cout << "Armor points: " << fighterArmor<< endl; cout << "Strength points: " << strength<< endl; }else if(damage == 999 && hogwarts == 0){ cout << "Harry Potter died by looking at Medusa. :(" << endl; strength = 0; cout << "Armor points: " << fighterArmor<< endl; cout << "Strength points: " << strength<< endl; death = true; //HP died }else if(damage > fighterArmor){ damage = damage - fighterArmor; strength = strength - damage; fighterArmor = 0; if(strength > 0){ cout << "Potter is hurt." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(strength <= 0 && hogwarts == 1){ //special ability cout << "Potter died." << endl; cout << "*Hogwarts* saves HP." << endl; hogwarts = 0; strength = 20; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(strength <= 0 && hogwarts == 0){ cout << "Potter has died. :(" << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; death = true; //HP died } } }
string HarryPotter::getUserName(){ return fightName; }
bool HarryPotter::checkDeath(){ return death; }
int HarryPotter::rollForAttack(){ int diceRoll = rand()%6 + 1; diceRoll = diceRoll + (rand()%6 + 1); return diceRoll; }
int HarryPotter::rollForDefense(){ int diceRoll = rand()%6 + 1; diceRoll = diceRoll + (rand()%6 + 1); return diceRoll; }
int HarryPotter::getFighterArmor(){ return fighterArmor; }
int HarryPotter::getFighterStrength(){ return strength; } harrypotter.hpp
#ifndef HARRYPOTTER_HPP #define HARRYPOTTER_HPP
#include "creature.hpp"
class HarryPotter:public Creature{ protected: int hogwarts; //special ability indicated in instructions
public: void setData(); int fightAttack(); void defend(int d); string getUserName(); bool checkDeath(); int rollForAttack(); int rollForDefense(); int getFighterArmor(); int getFighterStrength(); ~HarryPotter() {} };
#endif
medusa.cpp
#include
void Medusa::setData(){ fightName = "Medusa"; fighterArmor = 3; strength = 8; death = false; }
int Medusa::fightAttack(){ int damage = rollForAttack(); if(damage == 12){ //call special ability cout << "Enemy has stared into Medusa and is now stone." << endl; damage = 999; }else if(damage != 12) { cout << "Medusa attacked for " << damage<< " damage points." << endl; }
return damage; }
void Medusa::defend(int d){ int defend = rollForDefense(); int damage;
if(d != 999){ cout << "Medusa defened for " << defend<< " points." << endl; damage = d - defend; }else{ damage = d; }
if(damage < 0){ cout << "Medusa defended herself." << endl; cout << "Armor points: " << fighterArmor<< endl; cout << "Strength points: " << strength<< endl; }else if(damage > 0 && damage <= fighterArmor){ fighterArmor = fighterArmor - damage; cout << "Medusa's armor absorbed attack."<< endl; cout << "Armor points: " << fighterArmor<< endl; cout << "Strength points: " << strength<< endl; }else if(damage = 999){ cout << "Medusa has died by seeing her reflection. :(" << endl; cout << "Armor points: 0" << endl; cout << "Strength points: 0" << endl; death = true; //medusa died }else if(damage > fighterArmor){ damage = damage - fighterArmor; strength = strength - damage; fighterArmor = 0; if(strength > 0){ cout << "Medusa hurt." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(strength <= 0){ cout << "Medusa died." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; death = true; //medusa died } } }
string Medusa::getUserName(){ return fightName; }
bool Medusa::checkDeath(){ return death; }
int Medusa::rollForAttack(){ int diceRoll = rand()%6 + 1; diceRoll = diceRoll + (rand()%6 + 1); return diceRoll; }
int Medusa::rollForDefense(){ int diceRoll = rand()%6 + 1; return diceRoll; }
int Medusa::getFighterArmor(){ return fighterArmor; }
int Medusa::getFighterStrength(){ return strength; }
medusa.hpp
#ifndef MEDUSA_HPP #define MEDUSA_HPP
#include "creature.hpp"
class Medusa:public Creature{ protected: public: void setData(); int fightAttack(); void defend(int d); string getUserName(); bool checkDeath(); int rollForAttack(); int rollForDefense(); int getFighterArmor(); int getFighterStrength(); ~Medusa() {} };
#endif
vampire.cpp
#include
void Vampire::setData(){ fightName = "Vampire"; fighterArmor = 1; strength = 18; death = false; }
int Vampire::fightAttack(){ int damage = rollForAttack(); cout << "Vampire attacked for " << damage << "damage points." << endl; return damage; }
void Vampire::defend(int d){ int charm = rand()%2 + 1;//special ability, sees if vampire charmed other fighter out of attack if(charm == 1){ cout << "Vampire charmed opponent out of attack."<< endl; cout << "Armor points: " << fighterArmor<< endl; cout << "Strength points: " << strength<< endl; }else{ int defend = rollForDefense(); int damage; if(d != 999){ cout << "Vampire defended for " << defend << " defensive points." << endl; damage = d - defend; } else{ damage = d; }
if(damage <= 0){ cout << "Vampire defended." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(damage == fighterArmor){ fighterArmor = fighterArmor - damage; cout << "Vampire armor absorbed attack."<< endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; } else if(damage == 999){ cout << "Vampire has died by looking at Medusa." << endl; cout << "Armor points: 0" << endl; cout << "Strength points: 0" << endl; death = true; //vampire died }else if(damage > fighterArmor){ damage = damage - fighterArmor; strength = strength - damage; fighterArmor = 0; if(strength > 0){ cout << "Vampire damaged but still lives." << endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; }else if(strength <= 0){ cout << "Vampire has died. :("<< endl; cout << "Armor points: " << fighterArmor << endl; cout << "Strength points: " << strength << endl; death = true; //vampire died } } } }
string Vampire::getUserName(){ return fightName; }
bool Vampire::checkDeath(){ return death; }
int Vampire::rollForAttack(){ int diceRoll = rand()%12 + 1; return diceRoll; }
int Vampire::rollForDefense(){ int diceRoll = rand()%6 + 1; return diceRoll; }
int Vampire::getFighterArmor(){ return fighterArmor; }
int Vampire::getFighterStrength(){ return strength; }
vampire.hpp
#ifndef VAMPIRE_HPP #define VAMPIRE_HPP
#include "creature.hpp" #include
class Vampire:public Creature{ protected:
public: void setData(); int fightAttack(); void defend(int d); string getUserName(); bool checkDeath(); int rollForAttack(); int rollForDefense(); int getFighterArmor(); int getFighterStrength(); ~Vampire(){} };
#endif
creature.hpp
#ifndef CREATURE_HPP #define CREATURE_HPP
#include
using namespace std;
class Creature{ protected: string fightName; int fighterArmor; int strength; bool death;
public: //all functions are virtual virtual void setData() = 0; virtual int fightAttack() = 0; virtual void defend(int d) = 0; virtual string getUserName() = 0; virtual bool checkDeath() = 0; virtual int rollForAttack() = 0; virtual int rollForDefense() = 0; virtual int getFighterArmor() = 0; virtual int getFighterStrength() = 0; virtual ~Creature() {} };
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
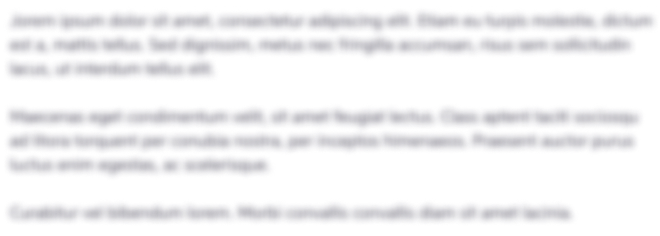
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started