Question
In C++ When running this program it should print which functions you implemented and ask the user which they want to run. I am having
In C++
When running this program it should print which functions you implemented and ask the user which they want to run.
I am having a little bit of trouble trying to put this whole program together to run as one, right now i just added the code i had which worked separate but I am trying to convert it into one project! Explain if possible!
***also having issue IN DICE GAME FUNCTION with running this to get it to say John one i get the other two statements but i never the one about john winning with the total number and their best rolls
#include
#include
#include
#include
#include
using namespace std;
//First Game
int GuessingGame()
{
// Guessing Game
int guesses = 0;
int guess;
int answer;
srand(time(NULL));
answer = rand() % 50 + 1;
cout << "This is the Guess that number game. ";
cout << "Now I am thinking of a number between 1 and 50, can you guess what number it is in "
<< guesses << "tries? ";
for (int i = 0; i < guesses; i++)
{
cout << "Guess number " << i + 1 << "; ";
cin >> guess;
if (guess != answer)
{
if (guess > answer)
cout << "Your answer is too high, try aiming lower. ";
else
cout << "Your answer is too low, try aiming higher. ";
}
else
{
cout << "You win! ";
return 0;
}
}
cout << "You lost: ";
cout << "The correct answer was: " << answer << endl;
return 0;
}
//Reverse Array Game
int ReverseArray()
{
int arr[20], n, temp, i, j;
n = 20;
//populate the array with random numbers (1..100)
for (i = 0; i < n; i++)
{
int r = (rand() % 100) + 1;
arr[i] = r;
}
cout << "Array before reversing : " << endl;
for (i = 0; i < 20; i++)
{
cout << arr[i] << " ";
}
// set i=0,j=19. Iterate till 10 th element
for (i = 0, j = n - 1; i < n / 2; i++, j--)
{
//swap arr[i] and arr[j]
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
cout << " Reverse array" << endl;
for (i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
return 0;
}
// is the Distinct Game
void printDistinct(int n)
{
srand(time(0));
int *arr = new int[n];
cout << "Original Array: ";
for (int i = 0; i < n; i++)
{
arr[i] = rand() % 100;
cout << arr[i] << " ";
}
cout << " Distinct Array: ";
// Pick all elements one by one
for (int i = 0; i < n; i++)
{
// Check if the picked element is already printed
int j;
for (j = 0; j < i; j++)
if (arr[i] == arr[j])
break;
// If not printed earlier, then print it
if (i == j)
cout << arr[i] << " ";
}
}
//Maximum-sum subsequence
int maxSum(int arr[], int n, int k)
{
// Compute sum of first window of size k
int res = 0;
for (int i = 0; i < k; i++)
res += arr[i];
int current = res;
for (int i = k; i < n; i++)
{
current += arr[i] - arr[i - k]; //moving the window to the right
res = max(res, current);
}
return res;
}
int main()
{
int arr[100];
for (int i = 0; i < 100; i++)
{
arr[i] = -50 + (std::rand() % (50 + 50 + 1));
}
int k;
cout << "enter sub array size:";
cin >> k;
cout << k << endl;
int n = sizeof(arr) / sizeof(arr[0]);
cout << " array is: " << endl;
for (int i = 0; i < 100; i++)
{
cout << arr[i] << " ";
}
cout << " maximum sum is : " << maxSum(arr, n, k);
return 0;
}
int main(){
printDistinct(15);
return 0;
}
//DiceGame Functions
int main()
{
int numThrows = 10; // Variable which defines number of throws
int diceSizeP = 6, diceSizeJ = 4; // Variables whch defines the number of sides of Pete dice and John size
int resultsP, resultsJ; // Result of each throw of John dice/Pete dice which is stored in resultsJ/resultsP respectively.
int totalP = 0; // Total varaibles which are used to store the sum of all the throws i.e 10 throws stored in respective variables, i.e sum of 10 throws for Pete dice
int totalJ = 0; // Total varaibles which are used to store the sum of all the throws i.e 10 throws stored in respective variables, i.e sum of 10 throws for John dice
srand(time(NULL)); // It should be run exactly once to generate different random number when we call rand() or else the output will be same set of sequence.
for (int i = 0; i < numThrows; i++) // Starting the game now with beginning for loop with 0 and bbreaking the loop when it reaches 10, here 10 means number of times the dice was thrown
{
resultsP = rand() % 31 + 6; // Here number of dice are 6, so minimum sum of 6 dice is 6 and maximum sum of six dice with 6 sided is 36(6*6), so the output will be between 6 and 36
// Formula will be minimum + rand()%(maximum-minimum+1)= 6+ rand()%31
totalP += resultsP; // Summation of each throw for Pete dice
cout << "Pete rolled a " << resultsP << endl; // Printing each throw sum for Pete
resultsJ = rand() % 28 + 9; // Here number of dice are 9, so minimum sum of 9 dice is 9 and maximum sum of nine dice with 4 side is 36(9*4), so the output will be between 9 and 36
// Formula will be minimum + rand()%(maximum-minimum+1)= 9+ rand()%28
totalJ += resultsJ; // Summation of each throw for Pete dice
cout << "John rolled a " << resultsJ << endl; // Printing each throw sum for Pete
if (resultsP != resultsJ)
{ // When each throw sum of John's dice and Pete's dice are not equal
if (resultsP > resultsJ) // Result of each throw comparision and display who is the winner
cout << "Pete answer is higher than John, Pete wins. ";
else if (resultsP < resultsJ) // Result of each throw comparision and display who is the winner
cout << "John answer is higher than Pete, John wins. ";
}
else
cout << "Draw both numbers are equal"; // When the results were same
}
if (totalP > totalJ) {
// Overall total of Pete is greater than John check
cout << "The total for Pete is " << totalP << " and total for John " << totalJ <<
", Pete won the overall game" << endl;
}
else if (totalJ < totalP) {
// Overall total of John is greater than Pete check
cout << "The total for Pete is " << totalP << " and total for John " << totalJ <<
", John won the overall game" << endl;
}
else {
cout << "The total for Pete is " << totalP << " and total for John " << totalJ <<
", the game is drawn between John and Pete" << endl; // Draw match
}
return 0;
}
int main() {
return
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
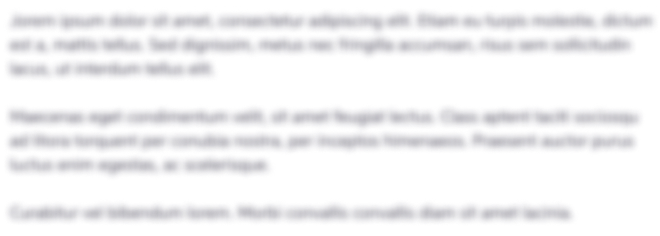
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started