Question
In C write a program that reads its input into an array and then uses selection sort to sort the array. In selection sort, we
In C write a program that reads its input into an array and then uses selection sort to sort the array. In selection sort, we first find the smallest element in the array and exchange it with the first array elements value; then we find the next smallest element in the array and exchange it with the second array elements value; and so on, until the array is sorted.
Have your program include four functions, besides main, which perform the following functions:
1. Read in an undetermined number of array elements
2. Print the array elements (call this function both before and after the elements are sorted)
3. Sort the array elements
4. Swap two elements of an array (this function will be called by your sort function)
Do not use any jump statements, e.g., the break statement.
Declare the maximum array size as a constant MAXSIZE with a value of 10.
Run your program using the following sets of input:
1. 5 12 -7 3 0
2. 1 2 3 4 5 7 6
3. Some input that will trigger each of your error checks, e.g., what happens when you enter more values than your declared array size or when invalid (nonnumeric) data is entered. Flush the invalid data and continue reading until end-of-file or the array is full. Print appropriate error messages for invalid character data and for too many values. Data items should still be sorted, even if invalid character data is encountered or too many values are entered.
The code should use the following:
1. meaningful variable names
2. comments on the following:
- program description
- function descriptions
- all variable and constant declarations
- ambiguous or complex sections of code
3. the correct use of local variables, local prototypes, and parameter passing
Selection Sort
algorithm in pseudocode
for i = 0 to (n - 2)
min = ai
min_index = i
for j = (i + 1) to (n - 1)
if aj < min
min = aj
min_index = j
endif
endfor
swap(a, i, min_index)
endfor
Step by Step Solution
There are 3 Steps involved in it
Step: 1
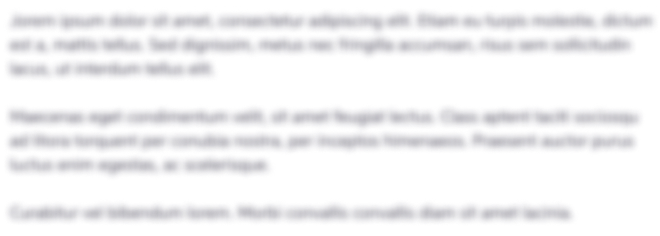
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started