Question
In C++, write one program that implements 4 classes with these requirements Person : Private : string name string address int age Public: void readPersonInfo()
In C++, write one program that implements 4 classes with these requirements
Person:
Private:
string name
string address
int age
Public:
void readPersonInfo() //asks for keyboard inputs of the person's 3 private attributes
void printPersonInfo() // displays the person's three private attributes
int personAGE() // returns the person's age
string personName() //returns the person's name
Student - derived from base class Person
Private:
string ID
string major
float gpa
Public:
void readStudentInfo() //frist calls the base class function readPersonInfo, then asks for keyboard inputs of the student's 3 private attributes
void printStudentInfo() //first invokes the base class function printPersonInfo, then prints the student's three private attributes
float studentGPA() //returns the student's GPA
Course
Private
string ID
string title
int credits
public
void printCourseInfo() //displays the Course's 3 private attributes
Class Constructor:
-constructor that is used to fill the data of the Course's 3 private attributes
CourseSelection derived class of base class Course
Private:
int sectionSize //number of students enrolled in the section
Student *roster //to store a list of the students' objects enrolled in the section
Public:
void fillRosterInfo() //read the info of all students in the section and store them in the roster
void printSectionInfo() //prints the section info starting with its course info followed by printing the info of all students in the section
void printTopGPAStudent() //prints the info of student with the highest GPA (assume there is only one student with a highest GPA)
Class Constructor and Destructor:
-Constructor takes as argument the 3 attributes of course to which the section belongs as well as the section size. First, this constructor invokes the base class (Course) constructor using the course 3 attributes. Then it dynamically creates the array needed for the roster attribute with the size sectionSize
-Destructor to delete any allocated memory
Dont need to write int main(). Just the 4 classes with the descriptions above.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
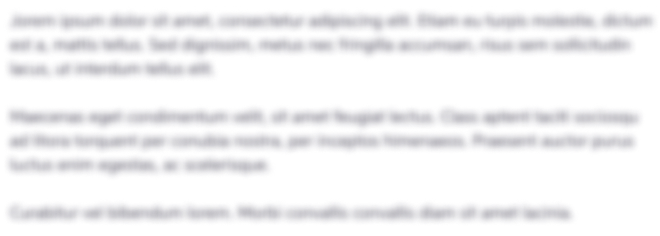
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started