Question
in c++, You have four classes--Polygon, Rectangle, Triangle, and Square. The latter three are subclasses of Polygon. Look through and understand the provided code. Implement
in c++, You have four classes--Polygon, Rectangle, Triangle, and Square. The latter three are subclasses of Polygon. Look through and understand the provided code. Implement the appropriate functions and classes where the comments indicate.
#include
using namespace std;
class Polygon{ protected: vector
class Rectangle : public Polygon { public: Rectangle() { sides.push_back(0.0); sides.push_back(0.0); } Rectangle(double a, double b) { sides.push_back(a); sides.push_back(b); } virtual double area() { // Implement this function double area = 1; for (int i=0; i < sides.size(); i++){ area *= sides[i]; } return area; } virtual double perimeter() { // Implement this function double perimeter = 0; for (int i=0; i < sides.size(); i++){ perimeter += sides[i]; } return perimeter; } };
class Triangle : public Polygon { double base; double height; public: Triangle() { sides.push_back(0.0); sides.push_back(0.0); sides.push_back(0.0); } Triangle(double a, double b, double c) { sides.push_back(a); sides.push_back(b); sides.push_back(c); } // Assume that the base and height will be set correctly externally void setBase(double _base) { base = _base; } void setHeight(double _height) { height = _height; } virtual double area() { // Implement this function double area = (0.5) * base * height; return area; } };
class Square : public Polygon { //Implement this class double side; public: Square() {sides.push_back(0.0);} Square(double s){sides.push_back(s);} void setSide(double_side) {side = _side;} //implement this class virtual double area() { //implement this function double area = side * side; return area; } virtual double perimeter() { //implement this function double perimeter = 4 * side; return perimeter; } };
// You may use main for testing int main(){ return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
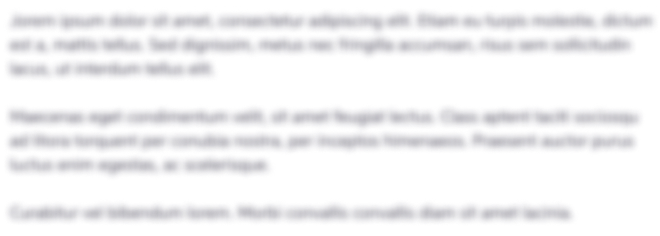
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started