Question
In C++, you will practice using mutexes and condition variables by implementing two versions of the readers and writers problem. The first version will favor
In C++, you will practice using mutexes and condition variables by implementing two versions of the readers and writers problem. The first version will favor readers and the second version will favor writers.
Version 1 Write a multi-threaded C++ program that gives readers priority over writers in accessing a shared variable. If any readers are waiting, they have priority over writer threads. Writers can only write when there are no readers waiting. It is acceptable to have multiple processes reading the shared variable at the same time. However, if one process is updating (writing) the shared variable, no other process may have access to the shared variable.
Implementation Notes for Version 1 Use the Pthreads (POSIX threads) library for threads and mutexes. Multiple readers and writers must be supported, and you should easily be able to vary the number of readers and writers. (Using a constant for this is fine.) Readers must read the shared variable X number of times before exiting. (X can be represented as a constant in your program.) Writers must write a random integer to a shared integer variableWriters must write the shared variable X number of times before exiting. Readers must print: o the thread type (reader or writer), thread number and value read. o the number of readers present in the critical region when the value is read. Sample output reader 2 read 17 3 reader(s) Writers must print: o the thread type (reader or writer), thread number and value written. o The number of readers present in the critical region when the value is written (should be 0). Sample output writer 2 wrote 147 0 reader(s) Be sure your output is aligned in a way that is easy to read. This will make testing and debugging your program easier.Before a reader or writer attempts to access the shared variable, it should wait some random amount of time. This will help ensure that reads and writes do not occur all at once. o Use the function usleep() to put a thread to sleep. It takes one argument, a time in microseconds. I had my threads sleep randomly between 10 and 100 microseconds each time before accessing the shared variable. You will need to experiment to find random times that work for you.
Version 2 In most cases, we would like updates to take place as soon as possible. This version will favor writers. Your implementation should allow readers to enter the critical section until a writer arrives. When a reader arrives and a writer is waiting, the reader should be suspended behind the writer instead of being allowed to enter the critical region immediately. A writer will need to wait until all readers that are active in the critical region are finished but should not wait for readers that arrived after its arrival. If more than one writer is waiting, all writers should be allowed to write before any readers are allowed to read again.
Implementation Notes for Version 2 All of the implementation notes for version 1 apply to version 2. Use the Pthreads (POSIX threads) for condition variables. In addition to printing the thread type, thread number, value read, and the number of readers present in the critical region when the value is read, readers must print: o The number of readers waiting o The number of writers waiting Sample output: reader 1 read 71 1 reader(s), 1 reader(s) waiting, 1 writer(s) waiting In addition to printing the thread type, thread number, value written, and the number of readers present in the critical region when the value is read, readers must print: o The number of readers waiting o The number of writers waiting writer 2 wrote 192 0 reader(s), 1 reader(s) waiting, 0 writer(s) waiting
Step by Step Solution
There are 3 Steps involved in it
Step: 1
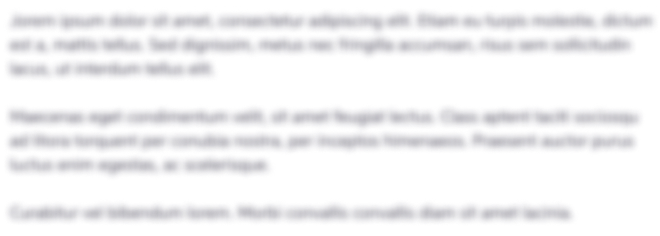
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started