Question
In Chapter 8, you created a Rental class for Sammys Seashore Supplies. Now extend the class to create a LessonWithRental class. In the extended class,
In Chapter 8, you created a Rental class for Sammys Seashore Supplies. Now extend the class to create a LessonWithRental class. In the extended class, include a new Boolean field that indicates whether a lesson is required or optional for the type of equipment rented. Also include a final array that contains Strings representing the names of the instructors for each of the eight equipment types, and store names that you choose in the array. Create a LessonWithRental constructor that requires arguments for an event number, minutes for the rental, and an integer equipment type. Pass the first two parameters to the Rental constructor, and assign the last parameter to the equipment type. For the first two equipment types (jet ski and pontoon boat), set the Boolean lesson required field to true; otherwise, set it to false. Also include a getInstructor() method that builds and returns a String including the String for the equipment type, a message that indicates whether a lesson is required, and the instructors name. Save the file as LessonWithRental.java.
The program uses an array of Rental objects and allows the user to sort Rentals in ascending order by contract number, equipment type, or price. Now modify the program to use an array of four LessonWithRental objects. Prompt the user for all values for each object, and then allow the user to continuously sort the LessonWithRental descriptions by contract number, equipment type, or price. Save the file as LessonWithRentalDemo.java.
class Rental { public static final int MINUTES_IN_HOUR = 60; public static final int HOUR_RATE = 40; public static final int CONTRACT_NUM_LENGTH = 4; public static final String[] EQUIP_TYPES = { "jet ski", "pontoon boat", "rowboat", "canoe", "kayak", "beach chair", "umbrella", "other" }; private String contractNumber; private int hours; private int extraMinutes; private double price; private String contactPhone; private int equipType; public Rental(String num, int minutes) { setContractNumber(num); setHoursAndMinutes(minutes); } public Rental() { this("A000", 0); } public void setContractNumber(String num) { boolean numOk = true; if (num.length() != CONTRACT_NUM_LENGTH || !Character.isLetter(num.charAt(0)) || !Character.isDigit(num.charAt(1)) || !Character.isDigit(num.charAt(2)) || !Character.isDigit(num.charAt(3))) contractNumber = "A000"; else contractNumber = num.toUpperCase(); } public void setHoursAndMinutes(int minutes) { hours = minutes / MINUTES_IN_HOUR; extraMinutes = minutes % MINUTES_IN_HOUR; if (extraMinutes <= HOUR_RATE) price = hours * HOUR_RATE + extraMinutes; else price = hours * HOUR_RATE + HOUR_RATE; } public String getContractNumber() { return contractNumber; } public int getHours() { return hours; } public int getExtraMinutes() { return extraMinutes; } public double getPrice() { return price; } public String getContactPhone() { String phone; phone = "(" + contactPhone.substring(0, 3) + ") " + contactPhone.substring(3, 6) + "-" + contactPhone.substring(6, 10); return phone; } public void setContactPhone(String phone) { final int VALID_LEN = 10; final String INVALID_PHONE = "0000000000"; contactPhone = ""; int len = phone.length(); for (int x = 0; x < len; ++x) { if (Character.isDigit(phone.charAt(x))) contactPhone += phone.charAt(x); } if (contactPhone.length() != VALID_LEN) contactPhone = INVALID_PHONE; } public void setEquipType(int eType) { if (eType < EQUIP_TYPES.length) equipType = eType; else equipType = EQUIP_TYPES.length - 1; } public int getEquipType() { return equipType; } public String getEquipTypeString() { return EQUIP_TYPES[equipType]; } }
import java.util.Scanner; public class RentalDemo { public static void main(String[] args) { String contractNum; int minutes; Rental[] rentals = new Rental[3]; int x; for (x = 0; x < rentals.length; ++x) { contractNum = getContractNumber(); minutes = getMinutes(); rentals[x] = new Rental(contractNum, minutes); rentals[x].setContactPhone(getPhone()); rentals[x].setEquipType(getType()); } for (x = 0; x < rentals.length; ++x) displayDetails(rentals[x]); } public static String getContractNumber() { String num; Scanner input = new Scanner(System.in); System.out.print(" Enter contract number >> "); num = input.nextLine(); return num; } public static int getMinutes() { int minutes; final int LOW_MIN = 60; final int HIGH_MIN = 7200; Scanner input = new Scanner(System.in); System.out.print("Enter minutes >> "); minutes = input.nextInt(); while (minutes < LOW_MIN || minutes > HIGH_MIN) { System.out.println("Time must be between " + LOW_MIN + " minutes and " + HIGH_MIN + " minutes"); System.out.print("Please reenter minutes >> "); minutes = input.nextInt(); } return minutes; } public static int getType() { int eType; Scanner input = new Scanner(System.in); System.out.println("Equipment types:"); for (int x = 0; x < Rental.EQUIP_TYPES.length; ++x) System.out.println(" " + x + " " + Rental.EQUIP_TYPES[x]); System.out.print("Enter equipment type >> "); eType = input.nextInt(); return eType; } public static void displayDetails(Rental r) { System.out.println(" Contract #" + r.getContractNumber()); System.out.println("For a rental of " + r.getHours() + " hours and " + r.getExtraMinutes() + " minutes, at a rate of $" + r.HOUR_RATE + " per hour and $1 per minute, the price is $" + r.getPrice()); System.out.println("Contact phone number is: " + r.getContactPhone()); System.out.println("Equipment rented is type #" + r.getEquipType() + " " + r.getEquipTypeString()); } public static Rental getLongerRental(Rental r1, Rental r2) { Rental longer = new Rental(); int minutes1; int minutes2; minutes1 = r1.getHours() * Rental.MINUTES_IN_HOUR + r1.getExtraMinutes(); minutes2 = r2.getHours() * Rental.MINUTES_IN_HOUR + r2.getExtraMinutes(); if (minutes1 >= minutes2) longer = r1; else longer = r2; return longer; } public static String getPhone() { String phone; Scanner input = new Scanner(System.in); System.out.print("Enter contact phone number >> "); phone = input.nextLine(); return phone; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
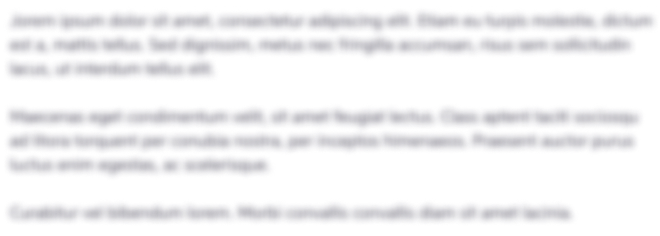
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started