Question
In class we implemented a map, which stores values by key. Add the following methods to both ArrayMap and LinkedMap: V remove (K key); V
In class we implemented a map, which stores values by key. Add the following methods to both ArrayMap and LinkedMap:
V remove (K key); V get (K key);
Then compile and run Maps.java. Your output should look like this for ArrayMap (and the mirror image for LinkedMap, since it adds to the front):
Here's the group {Maurice=20, Sylvia=18}
Leo is joining them {Maurice=20, Sylvia=18, Leo=7}
Sylvia just had a birthday Her previous age was 18 {Maurice=20, Sylvia=19, Leo=7}
Here are the people in the group [Maurice, Sylvia, Leo]
Is Sylvia in the group? yes {Maurice=20, Sylvia=19, Leo=7}
Sylvia has moved away She was 19 {Maurice=20, Leo=7} Is Sylvia in the group? no Trying to remove Sylvia again null
How old is Maurice? 20 Trying to add Kyle (age unknown) Key/value can't be null
/* * driver program for ArrayMap and LinkedMap */ public class Maps { public static void main (String [] args) { try { //Mapages = new ArrayMap (); Map ages = new LinkedMap (); // creating map ages.add("Maurice", 20); ages.add("Sylvia", 18); System.out.println(" Here's the group " + ages); // adding key not in map ages.add("Leo", 7); System.out.println(" Leo is joining the group " + ages); // adding key already in map (= replacing value) System.out.println(" Sylvia just had a birthday"); System.out.println("Her old age was " + ages.add("Sylvia", 19)); System.out.println(ages); // printing keys System.out.println(" Here are the people in the group"); System.out.println(ages.getKeys()); // searching for key System.out.println(" Is Sylvia in the group? " + (ages.containsKey("Sylvia") ? "yes" : "no")); System.out.println(ages); // removing pair System.out.println(" Sylvia has moved away"); System.out.println("Her age when she left " + ages.remove("Sylvia")); System.out.println(ages); System.out.println("Is Sylvia in the group? " + (ages.containsKey("Sylvia") ? "yes" : "no")); // trying to remove pair that isn't in map System.out.println("Trying to remove Sylvia again"); System.out.println(ages.remove("Sylvia")); // getting value for key System.out.println(" How old is Maurice? " + ages.get("Maurice")); // trying to add null value System.out.println(" Trying to add Kyle (his age is unknown"); ages.add("Kyle", null); } catch (IllegalArgumentException e) { System.out.println(e.getMessage()); } } }
/* * interface for a map * * data stored by key, with no duplicate keys allowed */ public interface Map{ V add (K key, V value); V remove (K key); V get(K key); boolean containsKey(K key); String getKeys(); int size(); String toString(); }
/* * array implementation of a map * * data stored by key, with no duplicate keys allowed */ public class ArrayMapimplements Map { private class Pair { private K key; private V value; public Pair(K key, V value) { this.key = key; this.value = value; } public String toString() { return key + "=" + value; } } public static final int DEFAULT_CAPACITY = 10; private Pair [] collection; private int size; public ArrayMap () { this(DEFAULT_CAPACITY); } @SuppressWarnings("unchecked") public ArrayMap (int capacity) { collection = (Pair []) new ArrayMap.Pair[capacity]; size = 0; } /* * if key in map, replaces old value with new and returns old value * otherwise adds key value pair and returns null */ public V add (K key, V value) { checkForNull(key, value); for (int i = 0; i < size; i++) { if (collection[i].key.equals(key)) { V oldValue = collection[i].value; collection[i].value = value; return oldValue; } } ensureSpace(); collection[size] = new Pair(key, value); size++; return null; } /* * removes key value pair from map and returns value * if key not found, returns null */ public V remove (K key) { return null; } /* * returns value stored for key * if key not found, returns null */ public V get(K key) { return null; } /* * returns true if key is in map */ public boolean containsKey(K key) { for (int i = 0; i < size; i++) { if (collection[i].key.equals(key)) { return true; } } return false; } public int size() { return size; } public String getKeys() { if (size == 0) { return "[]"; } String s = "["; for (int i = 0; i < size; i++) { s+= collection[i].key; if (i < size-1) s+= ", "; } return s + "]"; } public String toString() { if (size == 0) { return "{}"; } String s = "{"; for (int i = 0; i < size; i++) { s+= collection[i]; if (i < size-1) s += ", "; } return s + "}"; } private void checkForNull (K key, V value) { if (key == null || value == null) { throw new IllegalArgumentException("Key/value can't be null"); } } private void ensureSpace() { if (size == collection.length) { @SuppressWarnings("unchecked") Pair [] larger = (Pair []) new ArrayMap.Pair[size*2]; for (int i = 0; i < size; i++) { larger[i] = collection[i]; } collection = larger; larger = null; } } }
/* * linked implementation of a map * * data stored by key, with no duplicate keys allowed */ public class LinkedMapimplements Map { private class Node { private K key; private V value; private Node next; public Node(K key, V value) { this.key = key; this.value = value; next = null; } public String toString() { return key + "=" + value; } } private Node head; private int size; public LinkedMap () { head = null; size = 0; } /* * if key in map, replaces old value with new and returns old value * otherwise adds key value pair and returns null */ public V add (K key, V value) { checkForNull(key, value); Node current = head; for (int i = 0; i < size; i++) { if (current.key.equals(key)) { V oldValue = current.value; current.value = value; return oldValue; } current = current.next; } Node newest = new Node(key, value); newest.next = head; head = newest; size++; return null; } /* * removes key value pair from map and returns value * if key not found, returns null */ public V remove (K key) { return null; } /* * returns value associated with key * if key not found, returns null */ public V get(K key) { return null; } /* * returns true if key is in map */ public boolean containsKey(K key) { Node current = head; while (current != null) { if (current.key.equals(key)) { return true; } current = current.next; } return false; } public int size() { return size; } public String getKeys() { String list = "["; Node current = head; while (current.next != null) { list += current.key + ", "; current = current.next; } return list + current.key + "]"; } public String toString() { if (size == 0) { return "{}"; } String list = "{"; Node current = head; while (current.next != null) { list += current + ", "; current = current.next; } return list + current + "}"; } private void checkForNull (K key, V value) { if (key == null || value == null) { throw new IllegalArgumentException("Key/value can't be null"); } } private Node getNode (K key) { Node current = head; int count = 0; while (current != null) { if (current.key.equals(key)) { return current; } current = current.next; count++; } return null; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
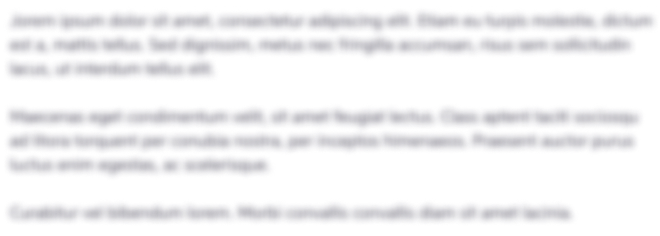
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started