Question
In climb.py, write a function climb(a,b) which takes in two integers a and b, and returns a list where each element at index i is
In climb.py, write a function climb(a,b) which takes in two integers a and b, and returns a list where each element at index i is the total number of distinct ways to climb from the step at index 0 to the step at index i. For climb(8,10), the elements of the result list look something like [ways to get to step at index 8, ways to get to step at index 9, ways to get to step at index 10].
For example:
climb(0, 3) will return [1,2,3,5]
o Explanation: The first three elements [1,2,3] are explained in the background. The element at index 4, 5 represents the number of different ways to reach the fourth step. The five different ways are listed below:
1 step + 1 step + 1 step + 1 step
1 step + 2 steps + 1 step
1 step + 1 step + 2 steps
2 steps + 2 steps
2 steps + 1 step + 1 step
Call function valid_range(a,b) in function climb(a,b)to check whether [a,b] is a valid range. If [a,b] is not a valid range, function climb(a,b) should return an empty list ([]).
Sol6:
Here's one possible implementation of the climb function:
def climb(a, b):
if not valid_range(a, b):
return []
steps = [1] * (b + 1)
for i in range(2, b + 1):
steps[i] = steps[i - 1] + steps[i - 2]
return steps[a:b + 1]
The climb function takes two integers a and b as input, representing the starting and ending steps of the staircase. The function first checks if the range specified by a and b is valid using the valid_range function. If the range is not valid, the function returns an empty list.
If the range is valid, the function initializes a list steps of length b+1, where each element is set to 1. The value of steps[i] represents the total number of distinct ways to climb from the step at index 0 to the step at index i.
The function then loops through the range 2 to b+1 and updates the value of steps[i] as the sum of the previous two values, steps[i-1] and steps[i-2]. This is because to reach the step at index i, one can either take a single step from the step at index i-1, or take two steps from the step at index i-2.
Finally, the function returns a sublist of steps from index a to index b.
Note: The valid_range function is not shown here, but it should be implemented as described in the previous question.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
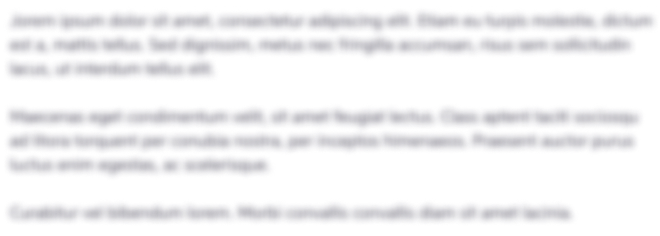
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started